题目二 磁盘调度算法的模拟实现及对比
时间: 2023-12-18 10:05:33 浏览: 20
好的,你的问题是磁盘调度算法的模拟实现及对比。磁盘调度算法是操作系统中的重要组成部分,用于优化磁盘访问的效率。常见的磁盘调度算法有FCFS、SSTF、SCAN、C-SCAN、LOOK和C-LOOK等。下面我将对这些算法进行简单介绍,并实现一个模拟程序进行对比。
1. FCFS(先来先服务):按照磁盘访问的请求顺序进行调度,不考虑请求的距离和方向,可能导致产生大量寻道时间。
2. SSTF(最短寻道时间优先):选择距离当前磁头最近的请求进行调度,可能会产生饥饿现象,即某些请求一直得不到满足。
3. SCAN(扫描算法):磁头按照一定方向移动,直到到达最边缘时改变方向,只考虑当前方向上的请求,可能会产生逆向请求等待的现象。
4. C-SCAN(循环扫描算法):与SCAN类似,但在到达最边缘时不改变方向,而是回到最开始的位置重新扫描。
5. LOOK(往返扫描算法):与SCAN类似,但在到达最边缘时不改变方向,而是直接返回到另一端。
6. C-LOOK(循环往返扫描算法):与LOOK类似,但在到达最边缘时不改变方向,而是回到最开始的位置重新扫描。
下面是一个磁盘调度算法的模拟程序示例:
```python
import queue
def fcfs(queue, start):
distance = 0
current = start
while not queue.empty():
next = queue.get()
distance += abs(next - current)
current = next
return distance
def sstf(queue, start):
distance = 0
current = start
while not queue.empty():
min_distance = float('inf')
min_index = -1
for i in range(queue.qsize()):
next = queue.get()
temp_distance = abs(next - current)
if temp_distance < min_distance:
min_distance = temp_distance
min_index = next
queue.put(next)
distance += min_distance
current = min_index
queue.get(current)
return distance
def scan(queue, start):
direction = 1
distance = 0
current = start
while not queue.empty():
min_distance = float('inf')
min_index = -1
for i in range(queue.qsize()):
next = queue.get()
temp_distance = abs(next - current)
if temp_distance < min_distance and (next - current) * direction >= 0:
min_distance = temp_distance
min_index = next
queue.put(next)
if min_index == -1:
direction = -direction
continue
distance += min_distance
current = min_index
queue.get(current)
return distance
def cscan(queue, start):
direction = 1
distance = 0
current = start
while not queue.empty():
min_distance = float('inf')
min_index = -1
for i in range(queue.qsize()):
next = queue.get()
temp_distance = abs(next - current)
if temp_distance < min_distance and (next - current) * direction >= 0:
min_distance = temp_distance
min_index = next
queue.put(next)
if min_index == -1:
distance += abs(current - 0)
current = 0
direction = 1
continue
distance += min_distance
current = min_index
queue.get(current)
return distance
def look(queue, start):
direction = 1
distance = 0
current = start
while not queue.empty():
min_distance = float('inf')
min_index = -1
for i in range(queue.qsize()):
next = queue.get()
temp_distance = abs(next - current)
if temp_distance < min_distance and (next - current) * direction >= 0:
min_distance = temp_distance
min_index = next
queue.put(next)
if min_index == -1:
direction = -direction
continue
distance += min_distance
current = min_index
queue.get(current)
return distance
def clook(queue, start):
direction = 1
distance = 0
current = start
while not queue.empty():
min_distance = float('inf')
min_index = -1
for i in range(queue.qsize()):
next = queue.get()
temp_distance = abs(next - current)
if temp_distance < min_distance and (next - current) * direction >= 0:
min_distance = temp_distance
min_index = next
queue.put(next)
if min_index == -1:
distance += abs(current - 0)
current = 0
direction = 1
continue
distance += min_distance
current = min_index
queue.get(current)
return distance
if __name__ == '__main__':
queue = queue.Queue()
for i in [98, 183, 37, 122, 14, 124, 65, 67]:
queue.put(i)
start = 53
print('FCFS:', fcfs(queue, start))
queue = queue.Queue()
for i in [98, 183, 37, 122, 14, 124, 65, 67]:
queue.put(i)
start = 53
print('SSTF:', sstf(queue, start))
queue = queue.Queue()
for i in [98, 183, 37, 122, 14, 124, 65, 67]:
queue.put(i)
start = 53
print('SCAN:', scan(queue, start))
queue = queue.Queue()
for i in [98, 183, 37, 122, 14, 124, 65, 67]:
queue.put(i)
start = 53
print('C-SCAN:', cscan(queue, start))
queue = queue.Queue()
for i in [98, 183, 37, 122, 14, 124, 65, 67]:
queue.put(i)
start = 53
print('LOOK:', look(queue, start))
queue = queue.Queue()
for i in [98, 183, 37, 122, 14, 124, 65, 67]:
queue.put(i)
start = 53
print('C-LOOK:', clook(queue, start))
```
这个程序可以模拟FCFS、SSTF、SCAN、C-SCAN、LOOK和C-LOOK等磁盘调度算法的执行过程,并计算出磁头的移动距离。在本程序中,模拟了一个磁盘请求队列,队列中的数字表示磁盘请求的磁道号,start表示磁头的初始位置。
以上就是磁盘调度算法的模拟实现及对比的介绍,希望对你有所帮助。
相关推荐
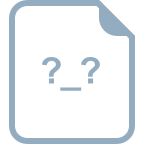
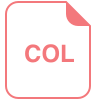












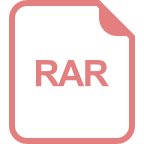