帮我看下这个方法怎么使用的27 /** 28 Compares the contents of two buffers. 29 30 This function compares Length bytes of SourceBuffer to Length bytes of DestinationBuffer. 31 If all Length bytes of the two buffers are identical, then 0 is returned. Otherwise, the 32 value returned is the first mismatched byte in SourceBuffer subtracted from the first 33 mismatched byte in DestinationBuffer. 34 35 If Length > 0 and DestinationBuffer is NULL, then ASSERT(). 36 If Length > 0 and SourceBuffer is NULL, then ASSERT(). 37 If Length is greater than (MAX_ADDRESS - DestinationBuffer + 1), then ASSERT(). 38 If Length is greater than (MAX_ADDRESS - SourceBuffer + 1), then ASSERT(). 39 40 @param DestinationBuffer A pointer to the destination buffer to compare. 41 @param SourceBuffer A pointer to the source buffer to compare. 42 @param Length The number of bytes to compare. 43 44 @return 0 All Length bytes of the two buffers are identical. 45 @retval Non-zero The first mismatched byte in SourceBuffer subtracted from the first 46 mismatched byte in DestinationBuffer. 47 48 **/ 49 INTN 50 EFIAPI 51 CompareMem ( 52 IN CONST VOID *DestinationBuffer, 53 IN CONST VOID *SourceBuffer, 54 IN UINTN Length 55 ) 56 { 57 if (Length == 0 || DestinationBuffer == SourceBuffer) { 58 return 0; 59 } 60 ASSERT (DestinationBuffer != NULL); 61 ASSERT (SourceBuffer != NULL); 62 ASSERT ((Length - 1) <= (MAX_ADDRESS - (UINTN)DestinationBuffer)); 63 ASSERT ((Length - 1) <= (MAX_ADDRESS - (UINTN)SourceBuffer)); 64 65 return InternalMemCompareMem (DestinationBuffer, SourceBuffer, Length); 66 }
时间: 2024-02-14 18:25:44 浏览: 156
这个方法是用来比较两个缓冲区的内容是否相同。它接受三个参数:DestinationBufferSourceBuffer和Length。
- DestinationBuffer是目缓冲区的指针,即要比的第一个缓冲区。
- SourceBuffer源缓冲区的指针,即要比较的第二个缓冲区- Length是要比较的字节数。
函数返回值为INTN类型。如果两个缓冲的所有字节都相同,则返回0否则,返回SourceBuffer中第一个不匹配的字节减去DestinationBuffer中第一个不匹配的字节的差值。
在函数内部,首先检查了一些特殊情况:
- 如果Length为0或者DestinationBuffer等于SourceBuffer,则直接返回0。
- 然后使用ASSERT宏断言了一些条件:
- 如果Length大于0且DestinationBuffer为NULL,则触发断言。
- 如果Length大于0且SourceBuffer为NULL,则触发断言。
- 如果Length大于(MAX_ADDRESS - DestinationBuffer + 1),则触发断言。
- 如果Length大于(MAX_ADDRESS - SourceBuffer + 1),则触发断言。
最后,调用了InternalMemCompareMem函数来执行实际的比较操作,并返回结果。
请注意,我只是根据代码分析给出了这个方法的大致作用和使用方式,具体的实现细节可能需要查看InternalMemCompareMem函数的代码。
相关问题
Write a simple function template for predict function isEqualTo that compares its two arguments of the same type with the equality operator (==) and returns true if they are equal and false if they are not equal. Use this function template in a program that calls isEqualTo only with a variety of built-in types. Now write a separate version of the program that calls isEqualTo with a user-defined class type Complex, but does not overload the equality operator. What happens when you attempt to run this program? Now overload the equality operator (with the operator function) operator ==. Now what happens when you attempt to run this program?
Here is the function template for isEqualTo:
```
template<typename T>
bool isEqualTo(const T& a, const T& b) {
return a == b;
}
```
Here is an example program that uses isEqualTo with built-in types:
```
#include <iostream>
int main() {
std::cout << std::boolalpha;
std::cout << isEqualTo(5, 5) << std::endl;
std::cout << isEqualTo(5, 7) << std::endl;
std::cout << isEqualTo('a', 'a') << std::endl;
std::cout << isEqualTo('a', 'b') << std::endl;
std::cout << isEqualTo(true, true) << std::endl;
std::cout << isEqualTo(true, false) << std::endl;
std::cout << isEqualTo(3.14, 3.14) << std::endl;
std::cout << isEqualTo(3.14, 2.71) << std::endl;
return 0;
}
```
When we attempt to run this program with the user-defined class type Complex, we get a compilation error because the compiler doesn't know how to compare two Complex objects.
To fix this, we can overload the equality operator in the Complex class:
```
class Complex {
public:
Complex(double real, double imag) : m_real(real), m_imag(imag) {}
double real() const { return m_real; }
double imag() const { return m_imag; }
private:
double m_real;
double m_imag;
};
bool operator==(const Complex& lhs, const Complex& rhs) {
return lhs.real() == rhs.real() && lhs.imag() == rhs.imag();
}
```
Now when we attempt to run the program with Complex objects, it works as expected:
```
#include <iostream>
class Complex {
public:
Complex(double real, double imag) : m_real(real), m_imag(imag) {}
double real() const { return m_real; }
double imag() const { return m_imag; }
private:
double m_real;
double m_imag;
};
bool operator==(const Complex& lhs, const Complex& rhs) {
return lhs.real() == rhs.real() && lhs.imag() == rhs.imag();
}
template<typename T>
bool isEqualTo(const T& a, const T& b) {
return a == b;
}
int main() {
std::cout << std::boolalpha;
std::cout << isEqualTo(Complex(1.0, 2.0), Complex(1.0, 2.0)) << std::endl;
std::cout << isEqualTo(Complex(1.0, 2.0), Complex(3.0, 4.0)) << std::endl;
return 0;
}
```
Output:
```
true
false
```
4 Experiments This section examines the effectiveness of the proposed IFCS-MOEA framework. First, Section 4.1 presents the experimental settings. Second, Section 4.2 examines the effect of IFCS on MOEA/D-DE. Then, Section 4.3 compares the performance of IFCS-MOEA/D-DE with five state-of-the-art MOEAs on 19 test problems. Finally, Section 4.4 compares the performance of IFCS-MOEA/D-DE with five state-of-the-art MOEAs on four real-world application problems. 4.1 Experimental Settings MOEA/D-DE [23] is integrated with the proposed framework for experiments, and the resulting algorithm is named IFCS-MOEA/D-DE. Five surrogate-based MOEAs, i.e., FCS-MOEA/D-DE [39], CPS-MOEA [41], CSEA [29], MOEA/DEGO [43] and EDN-ARM-OEA [12] are used for comparison. UF1–10, LZ1–9 test problems [44, 23] with complicated PSs are used for experiments. Among them, UF1–7, LZ1–5, and LZ7–9 have 2 objectives, UF8–10, and LZ6 have 3 objectives. UF1–10, LZ1–5, and LZ9 are with 30 decision variables, and LZ6–8 are with 10 decision variables. The population size N is set to 45 for all compared algorithms. The maximum number of FEs is set as 500 since the problems are viewed as expensive MOPs [39]. For each test problem, each algorithm is executed 21 times independently. For IFCS-MOEA/D-DE, wmax is set to 30 and η is set to 5. For the other algorithms, we use the settings suggested in their papers. The IGD [6] metric is used to evaluate the performance of each algorithm. All algorithms are examined on PlatEMO [34] platform.
4 实验
本节将研究所提出的IFCS-MOEA框架的有效性。首先,在第4.1节中介绍实验设置。其次,在第4.2节中研究IFCS对MOEA/D-DE的影响。然后,在第4.3节中,将IFCS-MOEA/D-DE与19个测试问题上的五种最先进的MOEA进行比较。最后,在第4.4节中,将IFCS-MOEA/D-DE与四种真实世界应用问题上的五种最先进的MOEA进行比较。
4.1 实验设置
实验中将MOEA/D-DE [23]与所提出的框架集成,得到的算法称为IFCS-MOEA/D-DE。比较使用了五种基于代理的MOEA,即FCS-MOEA/D-DE [39],CPS-MOEA [41],CSEA [29],MOEA/D-EGO [43]和EDN-ARM-OEA [12]。使用了具有复杂PS的UF1-10、LZ1-9测试问题[44,23]。其中,UF1-7、LZ1-5和LZ7-9具有2个目标,UF8-10和LZ6具有3个目标。UF1-10、LZ1-5和LZ9具有30个决策变量,LZ6-8具有10个决策变量。所有比较算法的种群大小N都设置为45。由于这些问题被视为昂贵的MOP [39],所以最大FE的数量设置为500。对于每个测试问题,每个算法都独立运行21次。对于IFCS-MOEA/D-DE,将wmax设置为30,将η设置为5。对于其他算法,我们使用其论文中建议的设置。使用IGD [6]指标评估每个算法的性能。所有算法都在PlatEMO [34]平台上进行检验。
阅读全文
相关推荐



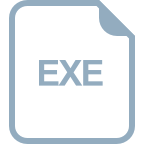













