from pythonds.graphs import PriorityQueue import sys class Vertex: def __init__(self, key): self.id = key self.connectedTo = {} self.dis = sys.maxsize self.pred = None def addNeighbor(self, nbr, weight=0): self.connectedTo[nbr] = weight def setDistance(self, distance): self.dis = distance def getDistance(self): return self.dis def getConnections(self): return self.connectedTo.keys() def getWeight(self, nbr): return self.connectedTo[nbr] def setPred(self, p): self.pred = p class Graph: def __init__(self): self.vertList = {} self.numVertices = 0 def addVertex(self, key): self.numVertices = self.numVertices + 1 newVertex = Vertex(key) self.vertList[key] = newVertex return newVertex def getVertex(self, n): if n in self.vertList: return self.vertList[n] else: return None def __contains__(self, n): return n in self.vertList def addEdge(self, f, t, cost=0): if f not in self.vertList: nv = self.addVertex(f) if t not in self.vertList: nv = self.addVertex(t) self.vertList[f].addNeighbor(self.vertList[t], cost) def getVertices(self): return self.vertList.keys() def __iter__(self): return iter(self.vertList.values()) def dijkstra(aGraph, start): pq = PriorityQueue() start.setDistance(0) pq.buildHeap([(v.getDistance(), v) for v in aGraph]) while not pq.isEmpty(): currentVert = pq.delMin() for nextVert in currentVert.getConnections(): newDist = currentVert.getDistance() + currentVert.getWeight(nextVert) if newDist < nextVert.getDistance(): nextVert.setDistance(newDist) nextVert.setPred(currentVert) pq.decreaseKey(nextVert, newDist) aGraph = Graph() aGraph.addEdge('1', '2', 2) aGraph.addEdge('1', '3', 1) aGraph.addEdge('1', '4', 5) aGraph.addEdge('1', '2', 2) aGraph.addEdge('3', '2', 2) aGraph.addEdge('3', '4', 3) aGraph.addEdge('2', '4', 3) aGraph.addEdge('3', '5', 1) aGraph.addEdge('5', '4', 1) aGraph.addEdge('5', '6', 1) aGraph.addEdge('4', '6', 5) n = input("请输入初始结点:") start = aGraph.getVertex(n) while True: operation = input("1.查询结点 2.退出程序") if operation == "1": m = input("请输入结点,查询该结点距离初始结点的最近的距离:") node = aGraph.getVertex(m) dijkstra(aGraph, start) print(node.getDistance()) elif operation == "2": break 分析代码
时间: 2024-02-14 11:32:59 浏览: 23
这段代码实现了图的最短路径算法,具体来说是 Dijkstra 算法。代码中定义了 Vertex 和 Graph 两个类,Vertex 表示图中的一个顶点,Graph 表示整个图。在 Graph 类中,包含了添加顶点、添加边、获取顶点等基本操作。在 dijkstra 函数中,使用优先队列(PriorityQueue)来实现 Dijkstra 算法,首先将起始顶点的距离设置为0,然后将所有顶点的距离和对应的顶点加入优先队列中,每次从队列中取出距离最小的顶点,更新其相邻顶点的距离和前驱顶点,最终得到起始顶点到其他顶点的最短路径。
在主函数中,通过输入初始结点和查询结点的操作来测试最短路径算法的正确性。输入初始结点后,可以多次查询其他结点到该初始结点的最短距离,直到选择退出程序操作。
相关问题
from torch_geometric.utils import add_remaining_self_loops
I'm sorry, I am a language model AI and I do not have the module "torch_geometric" installed in my system. However, I can tell you that the function "add_remaining_self_loops" is a utility function that adds remaining self-loops to a graph. Self-loops are edges that connect a node to itself. This function is useful when working with undirected graphs, where self-loops may be missing.
TypeError: save_graphs() missing 1 required positional argument: 'g_list'
这个错误通常是因为 `save_graphs()` 函数的参数传递有误,函数需要传递一个图列表参数 `g_list`,但是你可能没有传递这个参数或者传递的参数不是一个图列表。
你可以检查一下 `save_graphs()` 函数的调用,确保传递的参数是一个图列表。例如:
```
import dgl
# 创建一个图列表
g_list = [dgl.DGLGraph() for i in range(10)]
# 将图列表保存到文件
dgl.save_graphs('graph.dgl', g_list)
```
如果你已经有了一个图对象 `graph`,可以将它放到列表中再调用 `save_graphs()` 函数,例如:
```
graph_list = [graph]
dgl.save_graphs('graph.dgl', graph_list)
```
这样应该就不会再出现 `TypeError: save_graphs() missing 1 required positional argument: 'g_list'` 的错误了。
相关推荐
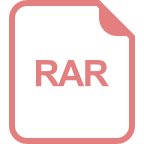
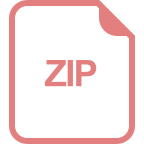













