用Java设计一个简单的图形软件系统,常见的图形有三角形,长方形,圆(椭圆)等,它们的功能有计算面积、计算周长、填充颜色等,每种图形还可以有自己的功能。请设计出这个简单的图形系统。
时间: 2024-06-09 10:10:57 浏览: 12
这个简单的图形系统可以使用Java图形界面(GUI)库来实现,比如Swing或JavaFX。下面是一个简单的设计:
1. 创建一个抽象类Shape作为所有图形的基类,其中包含计算面积和周长的抽象方法,以及填充颜色的成员变量和方法。
```java
public abstract class Shape {
protected Color fillColor;
public Shape(Color fillColor) {
this.fillColor = fillColor;
}
public abstract double getArea();
public abstract double getPerimeter();
public void setFillColor(Color fillColor) {
this.fillColor = fillColor;
}
public Color getFillColor() {
return fillColor;
}
}
```
2. 创建三角形、长方形和圆形这三个具体的图形类,它们都继承Shape类,并实现Shape类中的抽象方法。
```java
public class Triangle extends Shape {
private double a, b, c;
public Triangle(Color fillColor, double a, double b, double c) {
super(fillColor);
this.a = a;
this.b = b;
this.c = c;
}
@Override
public double getArea() {
double p = (a + b + c) / 2;
return Math.sqrt(p * (p - a) * (p - b) * (p - c));
}
@Override
public double getPerimeter() {
return a + b + c;
}
// Triangle-specific method
public boolean isRightTriangle() {
return a * a + b * b == c * c || b * b + c * c == a * a || c * c + a * a == b * b;
}
}
public class Rectangle extends Shape {
private double width, height;
public Rectangle(Color fillColor, double width, double height) {
super(fillColor);
this.width = width;
this.height = height;
}
@Override
public double getArea() {
return width * height;
}
@Override
public double getPerimeter() {
return 2 * (width + height);
}
// Rectangle-specific method
public boolean isSquare() {
return width == height;
}
}
public class Circle extends Shape {
private double radius;
public Circle(Color fillColor, double radius) {
super(fillColor);
this.radius = radius;
}
@Override
public double getArea() {
return Math.PI * radius * radius;
}
@Override
public double getPerimeter() {
return 2 * Math.PI * radius;
}
// Circle-specific method
public double getDiameter() {
return 2 * radius;
}
}
```
3. 创建一个GUI界面,用于用户输入各种图形的参数和填充颜色,并显示计算出的面积和周长。
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class ShapeGUI extends JFrame implements ActionListener {
private JComboBox<String> shapeComboBox;
private JTextField param1TextField, param2TextField, param3TextField;
private JButton calculateButton;
private JLabel areaLabel, perimeterLabel;
private JPanel shapePanel;
public ShapeGUI() {
setTitle("Simple Shape Calculator");
setSize(400, 300);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JPanel mainPanel = new JPanel();
mainPanel.setLayout(new BorderLayout());
JPanel inputPanel = new JPanel();
inputPanel.setLayout(new GridLayout(4, 2));
shapeComboBox = new JComboBox<String>(new String[]{"Triangle", "Rectangle", "Circle"});
shapeComboBox.addActionListener(this);
inputPanel.add(new JLabel("Shape:"));
inputPanel.add(shapeComboBox);
param1TextField = new JTextField();
inputPanel.add(new JLabel("Param 1:"));
inputPanel.add(param1TextField);
param2TextField = new JTextField();
inputPanel.add(new JLabel("Param 2:"));
inputPanel.add(param2TextField);
param3TextField = new JTextField();
inputPanel.add(new JLabel("Param 3:"));
inputPanel.add(param3TextField);
mainPanel.add(inputPanel, BorderLayout.NORTH);
shapePanel = new JPanel();
shapePanel.setPreferredSize(new Dimension(300, 150));
mainPanel.add(shapePanel, BorderLayout.CENTER);
JPanel outputPanel = new JPanel();
outputPanel.setLayout(new GridLayout(2, 2));
outputPanel.add(new JLabel("Area:"));
areaLabel = new JLabel("");
outputPanel.add(areaLabel);
outputPanel.add(new JLabel("Perimeter:"));
perimeterLabel = new JLabel("");
outputPanel.add(perimeterLabel);
mainPanel.add(outputPanel, BorderLayout.SOUTH);
calculateButton = new JButton("Calculate");
calculateButton.addActionListener(this);
mainPanel.add(calculateButton, BorderLayout.EAST);
setContentPane(mainPanel);
setVisible(true);
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == shapeComboBox) {
String shape = (String) shapeComboBox.getSelectedItem();
if (shape.equals("Triangle")) {
param1TextField.setText("");
param2TextField.setText("");
param3TextField.setText("");
param1TextField.setEnabled(true);
param2TextField.setEnabled(true);
param3TextField.setEnabled(true);
} else if (shape.equals("Rectangle")) {
param1TextField.setText("");
param2TextField.setText("");
param3TextField.setText("");
param1TextField.setEnabled(true);
param2TextField.setEnabled(true);
param3TextField.setEnabled(false);
} else if (shape.equals("Circle")) {
param1TextField.setText("");
param2TextField.setText("");
param3TextField.setText("");
param1TextField.setEnabled(true);
param2TextField.setEnabled(false);
param3TextField.setEnabled(false);
}
shapePanel.removeAll();
shapePanel.revalidate();
shapePanel.repaint();
} else if (e.getSource() == calculateButton) {
String shape = (String) shapeComboBox.getSelectedItem();
try {
if (shape.equals("Triangle")) {
double a = Double.parseDouble(param1TextField.getText());
double b = Double.parseDouble(param2TextField.getText());
double c = Double.parseDouble(param3TextField.getText());
Triangle t = new Triangle(Color.WHITE, a, b, c);
shapePanel = new TrianglePanel(t);
areaLabel.setText(String.format("%.2f", t.getArea()));
perimeterLabel.setText(String.format("%.2f", t.getPerimeter()));
} else if (shape.equals("Rectangle")) {
double width = Double.parseDouble(param1TextField.getText());
double height = Double.parseDouble(param2TextField.getText());
Rectangle r = new Rectangle(Color.WHITE, width, height);
shapePanel = new RectanglePanel(r);
areaLabel.setText(String.format("%.2f", r.getArea()));
perimeterLabel.setText(String.format("%.2f", r.getPerimeter()));
} else if (shape.equals("Circle")) {
double radius = Double.parseDouble(param1TextField.getText());
Circle c = new Circle(Color.WHITE, radius);
shapePanel = new CirclePanel(c);
areaLabel.setText(String.format("%.2f", c.getArea()));
perimeterLabel.setText(String.format("%.2f", c.getPerimeter()));
}
shapePanel.setPreferredSize(new Dimension(300, 150));
shapePanel.setBackground(Color.WHITE);
mainPanel.add(shapePanel, BorderLayout.CENTER);
mainPanel.revalidate();
mainPanel.repaint();
} catch (NumberFormatException ex) {
JOptionPane.showMessageDialog(this, "Invalid input", "Error", JOptionPane.ERROR_MESSAGE);
}
}
}
public static void main(String[] args) {
new ShapeGUI();
}
}
class TrianglePanel extends JPanel {
private Triangle triangle;
public TrianglePanel(Triangle triangle) {
this.triangle = triangle;
}
@Override
public void paintComponent(Graphics g) {
super.paintComponent(g);
int[] xPoints = {10, 150, 290};
int[] yPoints = {140, 10, 140};
g.setColor(triangle.getFillColor());
g.fillPolygon(xPoints, yPoints, 3);
g.setColor(Color.BLACK);
g.drawPolygon(xPoints, yPoints, 3);
}
}
class RectanglePanel extends JPanel {
private Rectangle rectangle;
public RectanglePanel(Rectangle rectangle) {
this.rectangle = rectangle;
}
@Override
public void paintComponent(Graphics g) {
super.paintComponent(g);
g.setColor(rectangle.getFillColor());
g.fillRect(10, 10, 280, 130);
g.setColor(Color.BLACK);
g.drawRect(10, 10, 280, 130);
}
}
class CirclePanel extends JPanel {
private Circle circle;
public CirclePanel(Circle circle) {
this.circle = circle;
}
@Override
public void paintComponent(Graphics g) {
super.paintComponent(g);
g.setColor(circle.getFillColor());
g.fillOval(10, 10, 280, 130);
g.setColor(Color.BLACK);
g.drawOval(10, 10, 280, 130);
}
}
```
4. 运行程序,输入图形参数和填充颜色,点击计算按钮,图形和计算结果将会显示在GUI界面上。
相关推荐
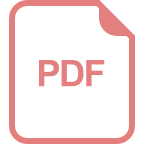
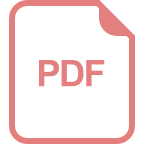


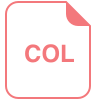
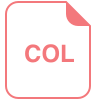
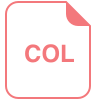









