在Linux内核驱动中,构建一个存放如下结构体的数组: struct msg { u16 module_id; u16 cmd_id; u16 cmd_subid; u16 complete; u8 data[128]; }; 请给出操作这个数组的函数,包括初始化,入数组,出数组,注销等。 再构建两个函数,在函数中使用操作数组的函数完成如下功能: 函数一初始化msg结构体,将msg所有成员设置为常数(其中msg的complete成员设置为0),向msg的data数组内放置两个unsigned int 类型数据a和b,之后将msg结构体放入数组中,触发函数二,使用usleep_range()函数等待函数二将msg的complete成员设置为1后,获取函数二放入的c并还原成unsigned int 类型,之后清除msg。 函数二需要从数组中取出msg,并进行解析:判断msg的module_id是否为0x1,如果不是,报错,如果是0x1,使用switch函数解析msg的cmd_id,再根据不同的cmd_id解析cmd_subid,具体解析内容为,取出在函数一向msg的data数组中放入的a和b,还原成unsigned int 类型数据,再将一个unsigned int 类型数据c=1000,放到msg的data数组内,之后,再将msg中的complete置1。请注意,确保函数二中操作的msg是函数一放入的msg,通过索引的方式找到唯一的msg
时间: 2024-04-05 09:30:54 浏览: 70
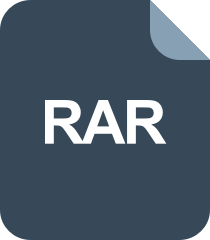
LINUX内核模块编程

以下是对应的操作数组的函数实现:
```
#define MSG_ARRAY_SIZE 100
// 定义全局变量存放msg数组和互斥锁
static struct msg msg_array[MSG_ARRAY_SIZE];
static int msg_array_index = 0;
static struct mutex msg_mutex;
// 初始化msg数组
void msg_array_init(void) {
mutex_init(&msg_mutex);
memset(msg_array, 0, sizeof(msg_array));
}
// 将msg结构体放入数组中
int msg_array_add(struct msg *new_msg) {
int i;
mutex_lock(&msg_mutex);
for (i = 0; i < MSG_ARRAY_SIZE; i++) {
if (msg_array[i].module_id == 0) {
memcpy(&msg_array[i], new_msg, sizeof(struct msg));
mutex_unlock(&msg_mutex);
return i;
}
}
mutex_unlock(&msg_mutex);
return -1;
}
// 从数组中取出msg
struct msg *msg_array_get(int index) {
struct msg *msg = NULL;
mutex_lock(&msg_mutex);
if (index >= 0 && index < MSG_ARRAY_SIZE && msg_array[index].module_id != 0) {
msg = &msg_array[index];
}
mutex_unlock(&msg_mutex);
return msg;
}
// 清除msg
void msg_array_remove(int index) {
mutex_lock(&msg_mutex);
if (index >= 0 && index < MSG_ARRAY_SIZE && msg_array[index].module_id != 0) {
memset(&msg_array[index], 0, sizeof(struct msg));
}
mutex_unlock(&msg_mutex);
}
// 函数一
void func_one(void) {
struct msg new_msg;
memset(&new_msg, 0, sizeof(struct msg));
new_msg.module_id = 0x1;
new_msg.cmd_id = 0x1;
new_msg.cmd_subid = 0x1;
new_msg.complete = 0;
unsigned int a = 100;
unsigned int b = 200;
memcpy(new_msg.data, &a, sizeof(unsigned int));
memcpy(new_msg.data + sizeof(unsigned int), &b, sizeof(unsigned int));
int index = msg_array_add(&new_msg);
// 触发函数二
while (msg_array_get(index)->complete == 0) {
usleep_range(1000, 2000);
}
unsigned int c = 0;
memcpy(&c, msg_array_get(index)->data, sizeof(unsigned int));
printk(KERN_INFO "func_one: c = %d\n", c);
msg_array_remove(index);
}
// 函数二
void func_two(struct msg *msg) {
if (msg->module_id != 0x1) {
printk(KERN_ERR "func_two: module_id is not 0x1\n");
return;
}
switch (msg->cmd_id) {
case 0x1:
switch (msg->cmd_subid) {
case 0x1:
unsigned int a = 0;
unsigned int b = 0;
memcpy(&a, msg->data, sizeof(unsigned int));
memcpy(&b, msg->data + sizeof(unsigned int), sizeof(unsigned int));
unsigned int c = 1000;
memcpy(msg->data, &c, sizeof(unsigned int));
msg->complete = 1;
printk(KERN_INFO "func_two: a = %d, b = %d, c = %d\n", a, b, c);
break;
default:
printk(KERN_ERR "func_two: invalid cmd_subid\n");
break;
}
break;
default:
printk(KERN_ERR "func_two: invalid cmd_id\n");
break;
}
}
```
阅读全文
相关推荐
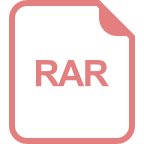









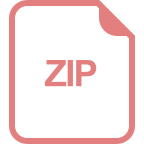
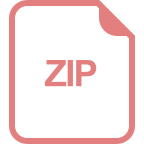
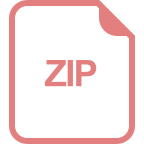
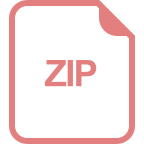