使用Pygame实现井字棋
时间: 2023-07-11 13:06:41 浏览: 53
好的,我理解了你的要求,我可以给你分享一下使用 Pygame 实现井字棋的代码。
```
import pygame
pygame.init()
# 设置游戏窗口
WINDOW_SIZE = (300, 300)
screen = pygame.display.set_mode(WINDOW_SIZE)
pygame.display.set_caption("Tic Tac Toe")
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
# 定义游戏状态
game_over = False
winner = None
# 创建游戏区域
board = [[None] * 3, [None] * 3, [None] * 3]
# 设置字体
font = pygame.font.SysFont(None, 30)
# 绘制游戏区域
def draw_board():
for row in range(3):
for col in range(3):
pygame.draw.rect(screen, WHITE, [(col * 100) + 10, (row * 100) + 10, 80, 80])
if board[row][col] == "X":
text = font.render("X", True, BLACK)
screen.blit(text, ((col * 100) + 35, (row * 100) + 35))
elif board[row][col] == "O":
text = font.render("O", True, BLACK)
screen.blit(text, ((col * 100) + 35, (row * 100) + 35))
# 检查游戏是否结束
def check_game_over():
global game_over, winner
# 检查行
for row in range(3):
if board[row][0] == board[row][1] == board[row][2] and board[row][0] is not None:
winner = board[row][0]
game_over = True
# 检查列
for col in range(3):
if board[0][col] == board[1][col] == board[2][col] and board[0][col] is not None:
winner = board[0][col]
game_over = True
# 检查对角线
if board[0][0] == board[1][1] == board[2][2] and board[0][0] is not None:
winner = board[0][0]
game_over = True
if board[0][2] == board[1][1] == board[2][0] and board[0][2] is not None:
winner = board[0][2]
game_over = True
# 检查是否平局
if all([all(row) for row in board]) and winner is None:
game_over = True
# 主循环
while not game_over:
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
if winner is None:
if event.type == pygame.MOUSEBUTTONDOWN:
# 获取鼠标点击位置
xpos, ypos = pygame.mouse.get_pos()
row = ypos // 100
col = xpos // 100
# 检查是否为合法位置
if board[row][col] is None:
# 设置该位置为当前玩家的符号
board[row][col] = "X"
# 切换到另一个玩家
if winner is None:
draw_board()
check_game_over()
pygame.display.update()
pygame.time.wait(500)
if winner is None:
open_spots = [(r, c) for r in range(3) for c in range(3) if board[r][c] is None]
if len(open_spots) > 0:
row, col = open_spots[0]
board[row][col] = "O"
draw_board()
pygame.display.update()
# 显示胜者
if winner == "X":
text = font.render("You win!", True, RED)
elif winner == "O":
text = font.render("Computer wins!", True, RED)
else:
text = font.render("Tie game!", True, RED)
screen.blit(text, (80, 140))
pygame.display.update()
# 等待用户关闭窗口
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
```
希望这个代码对你有帮助!
相关推荐
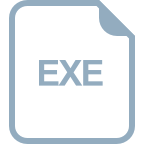
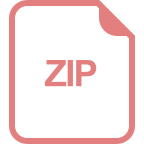











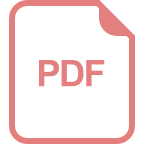
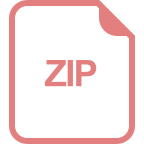