编写一个程序,分别使用字节流和字符流复制一个文本文件
时间: 2023-05-31 13:18:20 浏览: 196
### 回答1:
使用字节流复制文本文件:
```java
import java.io.*;
public class ByteStreamCopy {
public static void main(String[] args) {
try {
FileInputStream fis = new FileInputStream("source.txt");
FileOutputStream fos = new FileOutputStream("target.txt");
byte[] buffer = new byte[1024];
int len;
while ((len = fis.read(buffer)) != -1) {
fos.write(buffer, 0, len);
}
fis.close();
fos.close();
System.out.println("文件复制成功!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
使用字符流复制文本文件:
```java
import java.io.*;
public class CharStreamCopy {
public static void main(String[] args) {
try {
FileReader fr = new FileReader("source.txt");
FileWriter fw = new FileWriter("target.txt");
char[] buffer = new char[1024];
int len;
while ((len = fr.read(buffer)) != -1) {
fw.write(buffer, 0, len);
}
fr.close();
fw.close();
System.out.println("文件复制成功!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
### 回答2:
编写一个程序来复制文本文件需要考虑许多因素,如何打开文件、读写文件、关闭文件等等。在 Java 中,有两种流可以用来读写文本文件,分别是字节流和字符流。下面将分别介绍如何使用这两种流来复制一个文本文件。
使用字节流复制文本文件
字节流用于读写二进制文件,读取时返回的是字节,写入时也只能写字节。但是,我们可以将字节转换为字符串,然后对其进行进一步处理。以下是使用字节流复制文本文件的详细步骤:
1.创建一个 FileInputStream 对象,并用它打开待复制的文件。
2.创建一个 FileOutputStream 对象,并用它打开输出文件。
3.创建一个 byte 数组,用于存储读入的字节。
4.使用 FileInputStream 的 read() 方法从输入文件中读取字节,然后把它们写入到输出文件中。
5.重复步骤 4,直到文件结束。
6.关闭两个流。
下面是字节流复制文本文件的示例代码:
try (FileInputStream inputStream = new FileInputStream("input.txt");
FileOutputStream outputStream = new FileOutputStream("output.txt");) {
byte[] buffer = new byte[1024];
int length;
while ((length = inputStream.read(buffer)) > 0) {
outputStream.write(buffer, 0, length);
}
} catch (Exception e) {
e.printStackTrace();
}
使用字符流复制文本文件
字符流用于读写文本文件,读取时返回的是字符,写入时也只能写字符。相比之下,字符流更适合处理文本文件。以下是使用字符流复制文本文件的详细步骤:
1.创建一个 FileReader 对象,并用它打开待复制的文件。
2.创建一个 FileWriter 对象,并用它打开输出文件。
3.创建一个 char 数组,用于存储读入的字符。
4.使用 FileReader 的 read() 方法从输入文件中读取字符,然后把它们写入到输出文件中。
5.重复步骤 4,直到文件结束。
6.关闭两个流。
下面是字符流复制文本文件的示例代码:
try (FileReader reader = new FileReader("input.txt");
FileWriter writer = new FileWriter("output.txt");) {
char[] buffer = new char[1024];
int length;
while ((length = reader.read(buffer)) > 0) {
writer.write(buffer, 0, length);
}
} catch (Exception e) {
e.printStackTrace();
}
无论是使用字节流还是字符流来复制文本文件,都需要注意关闭流的操作。只有在保证流已经被关闭之后,我们才能确保文件的操作已经完成。
### 回答3:
在Java中,我们可以通过字节流和字符流来实现文件的复制。字节流以字节为单位进行文件操作,而字符流以Unicode字符为单位进行文件操作。下面我们将分别使用字节流和字符流来实现文件的复制。
使用字节流复制文本文件:
1. 创建一个FileInputStream对象和一个FileOutputStream对象,分别用于读取源文件和写入目标文件的数据。
2. 使用byte数组来缓存读取源文件的字节数据,每次读取一段数据,然后将其写入目标文件中。
3. 复制完成后,要记得关闭输入流和输出流。
以下是实现代码:
public static void copyFileByByte(String source, String target) throws IOException {
FileInputStream inputStream = null;
FileOutputStream outputStream = null;
try {
inputStream = new FileInputStream(source);
outputStream = new FileOutputStream(target);
byte[] buffer = new byte[1024];
int length;
while ((length = inputStream.read(buffer)) > 0) {
outputStream.write(buffer, 0, length);
}
} finally {
if (inputStream != null) {
inputStream.close();
}
if (outputStream != null) {
outputStream.close();
}
}
}
使用字符流复制文本文件:
1. 创建一个FileReader对象和一个FileWriter对象,分别用于读取源文件和写入目标文件的数据。
2. 使用char数组来缓存读取源文件的字符数据,每次读取一段数据,然后将其写入目标文件中。
3. 复制完成后,要记得关闭输入流和输出流。
以下是实现代码:
public static void copyFileByChar(String source, String target) throws IOException {
FileReader reader = null;
FileWriter writer = null;
try {
reader = new FileReader(source);
writer = new FileWriter(target);
char[] buffer = new char[1024];
int length;
while ((length = reader.read(buffer)) > 0) {
writer.write(buffer, 0, length);
}
} finally {
if (reader != null) {
reader.close();
}
if (writer != null) {
writer.close();
}
}
}
以上便是使用字节流和字符流分别实现文件复制的过程,两种方法均可以实现对文本文件的复制操作。
相关推荐
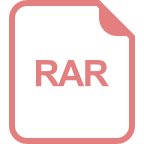
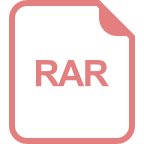
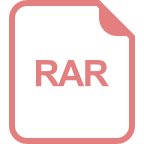













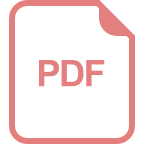
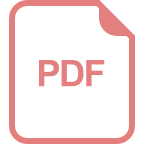
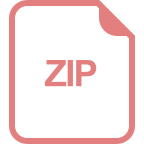