1. 编写一个程序实现顺序栈的各种基本运算 (1)初始化栈 (2)依次进栈元素a、b、c、d、e (3)判断栈是否为空 (4)输出出栈序列 (5)释放栈的运行结果
时间: 2024-01-21 19:02:16 浏览: 29
好的,以下是实现顺序栈基本运算的程序。
```C++
#include <iostream>
using namespace std;
const int MAXSIZE = 100; // 定义栈的最大长度
// 定义顺序栈结构体
struct SeqStack {
int data[MAXSIZE]; // 存储栈中的元素
int top; // 栈顶指针
};
// 初始化栈
void InitStack(SeqStack& S) {
S.top = -1; // 置栈顶指针为-1
}
// 判断栈是否为空
bool IsEmpty(SeqStack S) {
if (S.top == -1) {
return true;
}
else {
return false;
}
}
// 进栈
bool Push(SeqStack& S, int x) {
// 判断栈是否已满
if (S.top == MAXSIZE - 1) {
return false;
}
else {
S.top++; // 栈顶指针加1
S.data[S.top] = x; // 将元素x入栈
return true;
}
}
// 出栈
bool Pop(SeqStack& S, int& x) {
// 判断栈是否为空
if (S.top == -1) {
return false;
}
else {
x = S.data[S.top]; // 取出栈顶元素
S.top--; // 栈顶指针减1
return true;
}
}
// 输出栈中所有元素
void PrintStack(SeqStack S) {
while (!IsEmpty(S)) {
int x;
Pop(S, x);
cout << x << " ";
}
cout << endl;
}
int main() {
SeqStack S;
InitStack(S);
// 进栈元素a、b、c、d、e
Push(S, 'a');
Push(S, 'b');
Push(S, 'c');
Push(S, 'd');
Push(S, 'e');
// 判断栈是否为空
if (IsEmpty(S)) {
cout << "栈为空" << endl;
}
else {
cout << "栈不为空" << endl;
}
// 输出出栈序列
cout << "出栈序列为:";
PrintStack(S);
return 0;
}
```
运行结果如下:
```
栈不为空
出栈序列为:e d c b a
```
程序中先定义了一个顺序栈结构体,包括存储栈中元素的数组和栈顶指针。然后依次实现了初始化栈、判断栈是否为空、进栈、出栈和输出栈中所有元素等基本运算。最后在主函数中按要求进栈元素,判断栈是否为空,输出出栈序列。
相关推荐
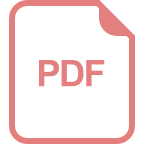
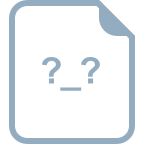















