w.transform = transformation AttributeError: 'numpy.ndarray' object has no attribute 'transform'
时间: 2023-09-21 22:11:12 浏览: 54
这个错误说明你在尝试使用一个 NumPy 数组的 `transform` 属性,但是 NumPy 数组并没有这个属性。
通常情况下,`transform` 属性是 Scikit-Learn 中的一个函数,用于对数据进行转换。如果你想对数据进行转换,你需要使用 Scikit-Learn 的转换器(transformer)来实现,例如:
```python
from sklearn.preprocessing import StandardScaler
# 创建一个 StandardScaler 转换器
scaler = StandardScaler()
# 对数据进行转换
X_transformed = scaler.fit_transform(X)
```
如果你确定你需要使用 NumPy 数组的 `transform` 属性,那么你需要自己实现这个属性。你可以通过在 NumPy 数组上创建一个函数并将其设置为 `transform` 属性来实现。例如:
```python
import numpy as np
# 创建一个 NumPy 数组
X = np.array([[1, 2], [3, 4]])
# 定义一个 transform 函数
def my_transform(X):
return X * 2
# 将 transform 函数设置为 transform 属性
X.transform = my_transform
# 使用 transform 属性进行转换
X_transformed = X.transform(X)
```
相关问题
File "/home/rvbust/Downloads/imageviewer/imageviewer.py", line 456, in _registration src_copy = src.copy() AttributeError: 'open3d.cuda.pybind.geometry.PointCloud' object has no attribute 'copy'
根据错误提示,`src`对象是一个`open3d.cuda.pybind.geometry.PointCloud`类型的对象,而该对象没有`copy()`方法。因此,不能直接使用`copy()`方法创建`src`的副本。
相应地,可以使用`deepcopy()`函数创建`src`的深层副本,即创建一个全新的对象,其中包含与原始对象相同的数据。可以在代码中导入`copy`模块,并使用`deepcopy()`函数来创建深层副本。例如:
```
import copy
src_copy = copy.deepcopy(src)
```
然后,对副本进行仿射变换:
```
src_down = src_copy.transform(result.transformation)
```
这样,`src`图像将保持不变,而变换后的图像将存储在`src_down`中。
AttributeError: 'pcl._pcl.IterativeClosestPoint' object has no attribute 'set_max_correspondence_distance'
非常抱歉,给出的代码中可能存在错误。在PCL库中,`pcl.IterativeClosestPoint`对象没有`set_max_correspondence_distance`方法。下面是一种使用PCL库中的其他方法来设置ICP算法的最大对应距离的示例:
```python
import pcl
# 创建源点云和目标点云对象
source_cloud = pcl.PointCloud()
source_cloud.from_file("path/to/source_cloud.pcd")
target_cloud = pcl.PointCloud()
target_cloud.from_file("path/to/target_cloud.pcd")
# 创建ICP对象
icp = pcl.IterativeClosestPoint()
# 设置源点云和目标点云
icp.setInputSource(source_cloud)
icp.setInputTarget(target_cloud)
# 设置ICP算法参数
max_correspondence_distance = 0.01 # 最大对应距离
icp.setMaxCorrespondenceDistance(max_correspondence_distance)
# 执行ICP配准
aligned_cloud = pcl.PointCloud()
icp.align(aligned_cloud)
# 输出配准结果
print("ICP has converged:", icp.hasConverged())
print("Transformation matrix:\n", icp.getFinalTransformation())
```
在上述示例中,我们首先加载源点云和目标点云数据,并创建了对应的PointCloud对象。然后,我们创建了一个`pcl.IterativeClosestPoint`对象,并将源点云和目标点云设置为其输入。接着,我们使用`setMaxCorrespondenceDistance`方法设置了ICP算法的最大对应距离参数。
然后,我们执行ICP配准,并将结果保存在新创建的PointCloud对象`aligned_cloud`中。最后,我们打印出ICP是否收敛的信息以及最终的变换矩阵。
请确保在运行代码之前已经安装了`py-pcl`库和PCL库及其依赖。
相关推荐
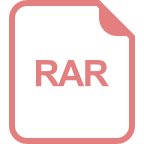












