opencvsharp 编写canny亚像素找边函数FindSubPixelEdge
时间: 2023-07-03 15:03:54 浏览: 170
OpenCvSharp是基于OpenCV的C#语言接口,可以方便地在C#中使用OpenCV的功能。对于Canny边缘检测算法,OpenCV提供了cv::Canny函数进行处理,不过该函数只能返回二值化的边缘图像,不能返回亚像素级别的边缘信息。如果需要获取亚像素级别的边缘信息,可以使用OpenCV提供的cv::findContours函数配合近似多边形算法进行处理。
下面是一个基于OpenCvSharp实现的FindSubPixelEdge函数的示例代码:
```csharp
public static Point[] FindSubPixelEdge(Mat src, int threshold1, int threshold2)
{
Mat edges = new Mat();
Cv2.Canny(src, edges, threshold1, threshold2);
Mat contours = new Mat();
Cv2.FindContours(edges, out contours, out _, RetrievalModes.List, ContourApproximationModes.ApproxTC89KCOS);
Point[][] approx = new Point[contours.Rows][];
for (int i = 0; i < contours.Rows; i++)
{
approx[i] = Cv2.ApproxPolyDP(contours.Row(i), Cv2.ArcLength(contours.Row(i), true) * 0.02, true);
}
Point[] subPixelEdges = new Point[approx.Length];
for (int i = 0; i < approx.Length; i++)
{
if (approx[i].Length >= 2)
{
double dx = approx[i][1].X - approx[i][0].X;
double dy = approx[i][1].Y - approx[i][0].Y;
double dist = Math.Sqrt(dx * dx + dy * dy);
if (dist > 0)
{
double nx = dy / dist;
double ny = -dx / dist;
double sumX = 0, sumY = 0, sumWeight = 0;
for (int j = 0; j < approx[i].Length; j++)
{
double x = approx[i][j].X;
double y = approx[i][j].Y;
double weight = Math.Abs(nx * (x - approx[i][0].X) + ny * (y - approx[i][0].Y));
sumX += x * weight;
sumY += y * weight;
sumWeight += weight;
}
subPixelEdges[i] = new Point(sumX / sumWeight, sumY / sumWeight);
}
}
}
return subPixelEdges;
}
```
该函数的主要思路是先使用Canny算法进行边缘检测,并使用findContours函数获取边缘轮廓信息。然后使用近似多边形算法对边缘轮廓进行简化,得到亚像素级别的边缘点信息。最后使用加权平均计算每个边缘点的精确位置。
阅读全文
相关推荐














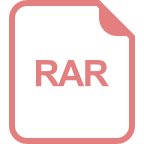
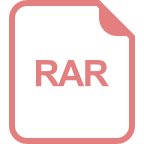

