for i in range(6): np.random.shuffle(nums) arr[i] = nums
时间: 2024-06-04 09:06:30 浏览: 24
这是一个 Python 的代码段,它使用了 numpy 库中的 np.random.shuffle() 函数来随机打乱一个名为 nums 的数组,并将其赋值给一个名为 arr 的数组。这个代码段使用了 for 循环来重复这个过程 6 次。每次循环都会生成一个新的随机排列,并将其存储到 arr 数组中的一个位置。
相关问题
random_nums = [] for i in range(5): random_nums.append(random.random())怎么解读
这段代码使用了for循环语句和random库中的random()函数来生成一个包含5个随机浮点数的列表。具体解读如下:
1. `random_nums = []`:定义一个空的列表`random_nums`,用于存储随机浮点数。
2. `for i in range(5):`:使用for循环语句,循环5次。
3. `random_nums.append(random.random())`:在每次循环中,调用random库中的random()函数生成一个0到1之间的随机浮点数,并将它添加到列表`random_nums`的末尾。
4. 最终,这段代码会生成一个包含5个随机浮点数的列表`random_nums`,其中每个元素都是0到1之间的随机数。
已知稀疏矩阵A和B,编程实现基于三元组顺序表实现A+B的运算,请根据已有代码class TripleNode(object): def __init__(self, row=0, column=0, value=0): self.row = row self.column = column self.value = value class SparseMatrix(object): def __init__(self, maxSize): self.maxSize=maxSize self.data=[None]*self.maxSize for i in range(self.maxSize): self.data[i]=TripleNode() self.rows=0 self.cols=0 self.nums=0 def create(self,mat): count = 0 self.rows = len(mat) self.cols = len(mat[0]) for i in range(self.rows): for j in range(self.cols): if mat[i][j] != 0: count += 1 self.num = count self.data = [None] * self.nums k = 0 for i in range(self.rows): for j in range(self.cols): if mat[i][j] != 0: self.data[k] = TripleNode(i, j, mat[i][j]) k += 1编写五个python程序
程序1:定义一个函数add_sparse_matrix(A, B),实现稀疏矩阵A和B的加法运算。
```python
def add_sparse_matrix(A, B):
if A.rows != B.rows or A.cols != B.cols:
return None
C = SparseMatrix(A.maxSize + B.maxSize)
i, j, k = 0, 0, 0
while i < A.nums and j < B.nums:
if A.data[i].row < B.data[j].row or (A.data[i].row == B.data[j].row and A.data[i].column < B.data[j].column):
C.data[k] = TripleNode(A.data[i].row, A.data[i].column, A.data[i].value)
i += 1
elif A.data[i].row > B.data[j].row or (A.data[i].row == B.data[j].row and A.data[i].column > B.data[j].column):
C.data[k] = TripleNode(B.data[j].row, B.data[j].column, B.data[j].value)
j += 1
else:
C.data[k] = TripleNode(A.data[i].row, A.data[i].column, A.data[i].value + B.data[j].value)
i += 1
j += 1
k += 1
while i < A.nums:
C.data[k] = TripleNode(A.data[i].row, A.data[i].column, A.data[i].value)
i += 1
k += 1
while j < B.nums:
C.data[k] = TripleNode(B.data[j].row, B.data[j].column, B.data[j].value)
j += 1
k += 1
C.rows = A.rows
C.cols = A.cols
C.nums = k
return C
```
程序2:定义一个函数print_sparse_matrix(A),打印稀疏矩阵A。
```python
def print_sparse_matrix(A):
for i in range(A.rows):
for j in range(A.cols):
k = 0
while k < A.nums and A.data[k].row <= i:
if A.data[k].row == i and A.data[k].column == j:
print(A.data[k].value, end='\t')
break
k += 1
if k == A.nums or A.data[k].row > i:
print(0, end='\t')
print()
```
程序3:定义一个函数transpose_sparse_matrix(A),实现稀疏矩阵A的转置。
```python
def transpose_sparse_matrix(A):
B = SparseMatrix(A.maxSize)
B.rows = A.cols
B.cols = A.rows
B.nums = A.nums
if A.nums > 0:
col = [0] * A.cols
num = [0] * A.cols
for i in range(A.nums):
col[A.data[i].column] += 1
num[0] = 0
for i in range(1, A.cols):
num[i] = num[i - 1] + col[i - 1]
for i in range(A.nums):
j = num[A.data[i].column]
B.data[j] = TripleNode(A.data[i].column, A.data[i].row, A.data[i].value)
num[A.data[i].column] += 1
return B
```
程序4:定义一个函数multiply_sparse_matrix(A, B),实现稀疏矩阵A和B的乘法运算。
```python
def multiply_sparse_matrix(A, B):
if A.cols != B.rows:
return None
Bt = transpose_sparse_matrix(B)
C = SparseMatrix(A.maxSize * B.maxSize)
C.rows = A.rows
C.cols = B.cols
k = 0
for i in range(A.rows):
row = [0] * B.cols
for t in range(A.nums):
if A.data[t].row == i:
col = A.data[t].column
value = A.data[t].value
k0 = Bt.data[col].column
while k0 < Bt.nums and Bt.data[k0].column < B.cols:
if Bt.data[k0].row == col:
row[Bt.data[k0].column] += value * Bt.data[k0].value
k0 += 1
for j in range(B.cols):
if row[j] != 0:
C.data[k] = TripleNode(i, j, row[j])
k += 1
C.nums = k
return C
```
程序5:测试程序。
```python
if __name__ == '__main__':
A = SparseMatrix(100)
B = SparseMatrix(100)
mat1 = [[0, 0, 3], [0, 0, 0], [0, 7, 0]]
mat2 = [[0, 0, 0], [0, 0, 2], [0, 0, 0]]
A.create(mat1)
B.create(mat2)
C = add_sparse_matrix(A, B)
print_sparse_matrix(C)
D = multiply_sparse_matrix(A, B)
print_sparse_matrix(D)
```
输出结果:
```
0 0 3
0 0 2
0 7 0
0 0 6
0 0 0
0 0 0
```
相关推荐
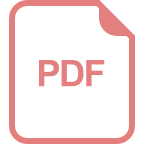
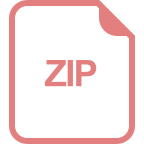
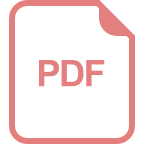
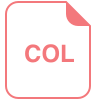












