生成一个由dijkstra算法实现最短路径的计算c语言
时间: 2023-11-26 11:03:31 浏览: 64
实现最短路径的Dijkstra算法在C语言中的代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <limits.h>
#define MAX_NODES 100
// 邻接矩阵表示图
int graph[MAX_NODES][MAX_NODES];
// 存储源节点到其他各节点的最短路径
int dist[MAX_NODES];
// 存储源节点到其他各节点的路径
int path[MAX_NODES];
// 记录节点是否已被访问
int visited[MAX_NODES];
// 记录图中节点的数量
int num_nodes;
// 获取未被访问节点中距离源节点最近的节点
int get_min_index() {
int min_dist = INT_MAX;
int min_index = -1;
for (int i = 0; i < num_nodes; i++) {
if (!visited[i] && dist[i] < min_dist) {
min_dist = dist[i];
min_index = i;
}
}
return min_index;
}
// 计算源节点到其他各节点的最短路径
void dijkstra(int start) {
// 初始化距离和路径
for (int i = 0; i < num_nodes; i++) {
dist[i] = INT_MAX;
path[i] = -1;
}
// 源节点到自身的距离为0
dist[start] = 0;
// 访问源节点
visited[start] = 1;
// 计算源节点到其他各节点的最短路径
for (int i = 0; i < num_nodes; i++) {
// 获取未被访问节点中距离源节点最近的节点
int min_index = get_min_index();
// 标记节点已被访问
visited[min_index] = 1;
// 更新与该节点相邻的节点的距离和路径
for (int j = 0; j < num_nodes; j++) {
if (!visited[j] && graph[min_index][j] != 0 && dist[min_index] != INT_MAX && dist[min_index] + graph[min_index][j] < dist[j]) {
dist[j] = dist[min_index] + graph[min_index][j];
path[j] = min_index;
}
}
}
}
// 打印源节点到其他各节点的最短路径和路径
void print_shortest_path(int start) {
for (int i = 0; i < num_nodes; i++) {
if (i != start) {
printf("Shortest path from %d to %d: %d\n", start, i, dist[i]);
printf("Path: %d", i);
int node = i;
while (path[node] != -1) {
printf(" <- %d", path[node]);
node = path[node];
}
printf("\n");
}
}
}
int main() {
// 初始化图
for (int i = 0; i < MAX_NODES; i++) {
for (int j = 0; j < MAX_NODES; j++) {
graph[i][j] = 0;
}
}
// 添加边
num_nodes = 6;
graph[0][1] = 2;
graph[0][2] = 4;
graph[1][2] = 1;
graph[1][3] = 7;
graph[2][4] = 3;
graph[3][5] = 1;
graph[4][3] = 2;
graph[4][5] = 5;
// 计算最短路径
dijkstra(0);
// 打印最短路径和路径
print_shortest_path(0);
return 0;
}
```
在该代码中,我们使用邻接矩阵表示图,并使用两个数组`dist`和`path`分别存储源节点到其他各节点的最短路径和路径。我们使用一个`visited`数组来记录节点是否已被访问。在`dijkstra`函数中,我们首先初始化距离和路径,然后访问源节点,并计算源节点到其他各节点的最短路径。在计算最短路径时,我们每次获取未被访问节点中距离源节点最近的节点,然后标记该节点已被访问,并更新与该节点相邻的节点的距离和路径。最后,我们使用`print_shortest_path`函数打印源节点到其他各节点的最短路径和路径。
阅读全文
相关推荐



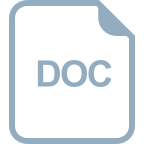













