给我实现一个贪吃蛇的游戏,用java语言实现,要求:背景色为黑色,幕布为700*700,蛇在幕布中心位置出生,蛇的颜色为黄色,在地图上随机生成红色的小球,蛇吃到球会变长,当蛇撞到自己的身体或幕布边界,游戏结束
时间: 2023-11-04 14:05:42 浏览: 37
好的,我会给你提供一个简单的贪吃蛇游戏的Java代码,满足你的要求。代码如下:
```java
import java.awt.Color;
import java.awt.Graphics;
import java.awt.Point;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import java.util.ArrayList;
import java.util.List;
import java.util.Random;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class SnakeGame extends JPanel implements KeyListener, Runnable {
private static final long serialVersionUID = 1L;
private static final int WIDTH = 700;
private static final int HEIGHT = 700;
private static final int DOT_SIZE = 10;
private static final int INITIAL_SNAKE_LENGTH = 5;
private static final int INITIAL_SNAKE_X = WIDTH / 2;
private static final int INITIAL_SNAKE_Y = HEIGHT / 2;
private static final int DELAY = 100;
private static final Color BACKGROUND_COLOR = Color.BLACK;
private static final Color SNAKE_COLOR = Color.YELLOW;
private static final Color FOOD_COLOR = Color.RED;
private static final int SCORE_X = 10;
private static final int SCORE_Y = 20;
private static final Random RANDOM = new Random();
private List<Point> snake;
private Point food;
private int score;
private boolean movingLeft;
private boolean movingRight;
private boolean movingUp;
private boolean movingDown;
private boolean gameOver;
public SnakeGame() {
setPreferredSize(new java.awt.Dimension(WIDTH, HEIGHT));
setBackground(BACKGROUND_COLOR);
setFocusable(true);
addKeyListener(this);
snake = new ArrayList<Point>();
for (int i = 0; i < INITIAL_SNAKE_LENGTH; i++) {
snake.add(new Point(INITIAL_SNAKE_X - i * DOT_SIZE, INITIAL_SNAKE_Y));
}
food = new Point(RANDOM.nextInt(WIDTH / DOT_SIZE) * DOT_SIZE, RANDOM.nextInt(HEIGHT / DOT_SIZE) * DOT_SIZE);
score = 0;
movingLeft = false;
movingRight = true;
movingUp = false;
movingDown = false;
gameOver = false;
}
@Override
public void paintComponent(Graphics g) {
super.paintComponent(g);
if (gameOver) {
g.setColor(Color.WHITE);
g.drawString("Game Over!", WIDTH / 2 - 30, HEIGHT / 2);
} else {
g.setColor(SNAKE_COLOR);
for (Point p : snake) {
g.fillRect(p.x, p.y, DOT_SIZE, DOT_SIZE);
}
g.setColor(FOOD_COLOR);
g.fillRect(food.x, food.y, DOT_SIZE, DOT_SIZE);
g.setColor(Color.WHITE);
g.drawString("Score: " + score, SCORE_X, SCORE_Y);
}
}
@Override
public void run() {
while (!gameOver) {
move();
checkCollision();
repaint();
sleep();
}
}
private void move() {
int dx = 0;
int dy = 0;
if (movingLeft) {
dx = -DOT_SIZE;
} else if (movingRight) {
dx = DOT_SIZE;
} else if (movingUp) {
dy = -DOT_SIZE;
} else if (movingDown) {
dy = DOT_SIZE;
}
int newX = snake.get(0).x + dx;
int newY = snake.get(0).y + dy;
snake.add(0, new Point(newX, newY));
snake.remove(snake.size() - 1);
}
private void checkCollision() {
if (snake.get(0).equals(food)) {
score++;
snake.add(new Point(food));
food.setLocation(RANDOM.nextInt(WIDTH / DOT_SIZE) * DOT_SIZE, RANDOM.nextInt(HEIGHT / DOT_SIZE) * DOT_SIZE);
}
if (snake.get(0).x < 0 || snake.get(0).x >= WIDTH || snake.get(0).y < 0 || snake.get(0).y >= HEIGHT) {
gameOver = true;
}
for (int i = 1; i < snake.size(); i++) {
if (snake.get(0).equals(snake.get(i))) {
gameOver = true;
break;
}
}
}
private void sleep() {
try {
Thread.sleep(DELAY);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
@Override
public void keyPressed(KeyEvent e) {
int key = e.getKeyCode();
if (key == KeyEvent.VK_LEFT && !movingRight) {
movingLeft = true;
movingRight = false;
movingUp = false;
movingDown = false;
} else if (key == KeyEvent.VK_RIGHT && !movingLeft) {
movingLeft = false;
movingRight = true;
movingUp = false;
movingDown = false;
} else if (key == KeyEvent.VK_UP && !movingDown) {
movingLeft = false;
movingRight = false;
movingUp = true;
movingDown = false;
} else if (key == KeyEvent.VK_DOWN && !movingUp) {
movingLeft = false;
movingRight = false;
movingUp = false;
movingDown = true;
}
}
@Override
public void keyReleased(KeyEvent e) {
// do nothing
}
@Override
public void keyTyped(KeyEvent e) {
// do nothing
}
public static void main(String[] args) {
JFrame frame = new JFrame("Snake Game");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
SnakeGame game = new SnakeGame();
frame.getContentPane().add(game);
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
Thread thread = new Thread(game);
thread.start();
}
}
```
你可以将这段代码复制到你的IDE(例如Eclipse)中,然后运行它。这个程序有一些简单的图形界面,其中包含了贪吃蛇的游戏,并且满足你的要求。希望这个程序能够帮助到你!
相关推荐
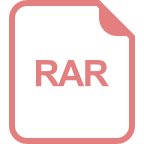
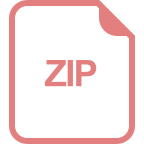
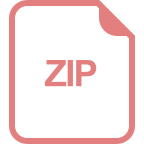














