#include<iostream> using namespace std; char map[101][101]; int num[101][101]; int main() { int h, w; cin >> h >> w; //输入网格图 for (int i = 1; i <= h; i++) { for (int j = 1; j <= w; j++) { cin >> map[i][j]; } } //障碍条件 num[1][1] = 1;//起点只有一种走法 for (int i = 2; i <= h; i++) { if (map[1][i] != '#')//如果该位置不是# { num[1][i] = num[1][i - 1];//只能从左边到达 } } for (int i = 2; i <= w; i++) { if (map[i][1] != '#')//如果该位置不是# { num[i][1] = num[i - 1][1];//只能从上边到达 } } //计算路径总数 for (int i = 2; i <= h; i++) { for (int j = 2; j <= w; j++) { if (map[i][j] != '#') { num[i][j] = num[i][j - 1] + num[i - 1][j];//能到达该位置的路径为上方位置和左方位置的路径之和 } } } cout << num[h][w] << endl; return 0; }这串代码为什么只需判断第一行和第一列是否为#
时间: 2023-11-25 15:04:19 浏览: 133
这段代码实现的是和我之前回答的问题类似的动态规划算法,用 $num_{i,j}$ 表示从起点到网格中第 $i$ 行第 $j$ 列的格子的不同走法数目。
对于第一行和第一列,因为只能从起点走到这些位置,所以它们的不同走法数目只能是 1。对于其他格子 $(i,j)$,它能到达的位置只有上方格子 $(i-1,j)$ 和左边格子 $(i,j-1)$,因此有:
$$
num_{i,j} = \begin{cases}
num_{i,j-1}+num_{i-1,j} & \text{if } map_{i,j}\neq\# \\
0 & \text{otherwise}
\end{cases}
$$
因为只有上方格子和左边格子能到达当前格子,所以其他格子不会影响当前格子的路径总数。因此只需要计算第一行和第一列,就可以根据递推式计算出所有格子的路径总数了。
相关问题
#include <stdio.h> #include <stdlib.h> #include <string.h> #include <pthread.h> #include <sys/time.h> #include <unistd.h> #include <pwd.h> #include <signal.h> #include <list> #include <algorithm> #include <iostream> #include <map> #include <string> #include <queue> #include <vector> #include <sstream> #define LOG_BRASERO_NUM 15 using namespace std; static char *g_cpBrasero[] = { (char *) "ID", (char *) "刻录时间", (char *) "刻录机型号", (char *) "光盘属性", (char *) "刻录状态", (char *) "计算机帐户", (char *) "文件名称", (char *) "文件大小", (char *) "文件类型", (char *) "测试1", (char *) "测试2", (char *) "测试3", (char *) "测试4", (char *) "测试5", (char *) "测试6", }; typedef struct _tagBraseroLog { char cpValue[1024]; } BRASEROLOG; int uosaarch_line_parse(char *pBuffer) { int index, len,lastLen; int ret = 0; char *begin = NULL; char *end = NULL; char *lastEnd = NULL; //debug printf("进入了扫描"); BRASEROLOG BraseroLog[LOG_BRASERO_NUM]; memset(&BraseroLog, 0, LOG_BRASERO_NUM * sizeof(BRASEROLOG)); for (index = 0; index < LOG_BRASERO_NUM; index++) { begin = strstr(pBuffer, g_cpBrasero[index]); if(NULL == begin) continue; begin=strstr(begin,"="); end = strstr(pBuffer, g_cpBrasero[index + 1]); //end--; if (begin != NULL) { len = strlen("="); unsigned long strSize = end - begin - len ; printf("BraseroLOg[%d]=%s\n",index,BraseroLog[index].cpValue); //strncpy(BraseroLog[index].cpValue, begin + len, std::min(strSize, sizeof(BraseroLog[index].cpValue) - 1)); // printf("PrintLog[%d] = %s\n",index,BraseroLog[index].cpValue); } return 0; } return 1; } int main(){ char a[500] = "ID=1689309873, 刻录时间=2023-07-14 12:44:34, 刻录机型号=TSSTcorp-CDDVDW-SE-218CB-R95M6YMDA00008, 光盘属性=DVD+R, 刻录状态=成功, 计算机帐户=hba, 文件名称=/home/hba/Desktop/刻录测试文件.txt, 文件大小=66 B, 文件类型=文档"; uosaarch_line_parse(a); return 0; }
你的代码主要是定义了一个函数 `uosaarch_line_parse` 来解析字符串,并将解析结果存储在 `BRASEROLOG` 结构体数组中。然后在 `main` 函数中调用了 `uosaarch_line_parse` 函数进行测试。
然而,在你的代码中有一些问题需要解决:
1. 在 `uosaarch_line_parse` 函数中,你使用了 `printf` 函数来打印调试信息。但是你没有在代码开头包含 `<stdio.h>` 头文件,所以编译器会发出警告。你需要在开头包含 `<stdio.h>` 头文件来解决这个问题。
2. 在 `uosaarch_line_parse` 函数中,你使用了 `strstr` 函数来查找字符串中的子字符串。然而,你没有检查 `strstr` 函数的返回值是否为 `NULL`。如果 `strstr` 函数找不到子字符串,它会返回 `NULL`,但你没有对这种情况进行处理。你需要在使用 `strstr` 函数之后,检查返回值是否为 `NULL`。
3. 在 `uosaarch_line_parse` 函数中,你使用了 `strncpy` 函数来将解析结果拷贝到 `BraseroLog` 结构体数组中。但是你注释掉了这行代码,并且没有使用其他方法将解析结果拷贝到结构体数组中。你需要取消注释这行代码,并确保解析结果正确地拷贝到结构体数组中。
4. 在 `uosaarch_line_parse` 函数中,你在循环的最后一行使用了 `return 1;`。这意味着只会处理第一个子字符串,并且函数会在第一个子字符串处理完成后立即返回。如果你想处理所有的子字符串并返回结果,你需要将 `return 1;` 移到循环结束后,以确保所有子字符串都被处理。
综上所述,你需要解决上述问题并进行适当的修改,以确保代码能够正确地解析字符串并返回结果。
c++实现#include <iostream>#include <string>#include <map>using namespace std;// 将字母转换为按键顺序string letter_to_key(char letter) { map<char, string> key_map = { {'a', "2"}, {'b', "22"}, {'c', "222"}, {'d', "3"}, {'e', "33"}, {'f', "333"}, {'g', "4"}, {'h', "44"}, {'i', "444"}, {'j', "5"}, {'k', "55"}, {'l', "555"}, {'m', "6"}, {'n', "66"}, {'o', "666"}, {'p', "7"}, {'q', "77"}, {'r', "777"}, {'s', "7777"}, {'t', "8"}, {'u', "88"}, {'v', "888"}, {'w', "9"}, {'x', "99"}, {'y', "999"}, {'z', "9999"}, {' ', "0"} }; return key_map[letter];}int main() { int t; cin >> t; for (int case_num = 1; case_num <= t; case_num++) { string s; cin >> s; string ans = ""; for (char letter : s) { ans += letter_to_key(letter); } cout << "Case#" << case_num << ": " << ans << endl; } return 0;}
这段代码实现了一个将字母转换为按键顺序的功能,使用了C++的STL库中的map数据结构。具体实现细节如下:
1. 包含了iostream、string和map三个头文件。
2. 使用了命名空间std。
3. 实现了一个letter_to_key函数,接受一个字母作为参数,返回一个按键序列。
4. 使用了一个map数据结构,将每个字母映射到对应的按键序列。
5. 在main函数中,先读入测试用例的数量t,然后针对每个测试用例,读入字符串s,使用letter_to_key函数将每个字母转换为按键序列,并将结果存储在ans中。最后输出答案。
需要注意的是,这段代码中的输入输出格式要求与题目要求有可能不一致,需要根据具体题目要求进行调整。
阅读全文
相关推荐
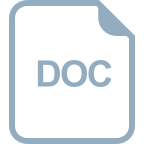
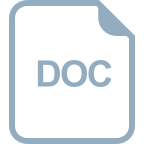
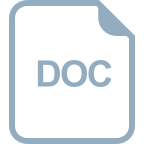


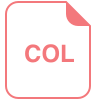
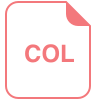










