void traverseDir(string path){ DIR *dir = opendir(path.c_str()); // 打开目录 if(!dir){ cerr << "Failed to open directory " << path << endl; return; } struct dirent *entry; while((entry = readdir(dir)) != NULL){ // 遍历目录 string name = entry->d_name; if(name == "." || name == "..") continue; string fullpath = path + "/" + name; struct stat st; if(stat(fullpath.c_str(), &st) == -1) continue; if(S_ISDIR(st.st_mode)){ // 目录 cout << "[dir] " << name << endl; traverseDir(fullpath); // 递归遍历子目录 } else if(S_ISREG(st.st_mode)){ // 文件 cout << "[file] " << name << endl; } } closedir(dir); }解释代码
时间: 2024-03-04 07:52:04 浏览: 165
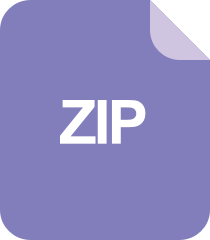
dir__list.zip_Free!_dir_lisr_php mysql_suorce
这段代码是 C++ 实现的遍历指定目录下所有文件和子目录的函数。它接受一个字符串参数 path,表示要遍历的目录路径。函数首先通过 opendir 函数打开目录,如果打开失败则输出错误信息并返回。接着使用 readdir 函数遍历目录,对于每个目录项,如果是 "." 或 ".." 则跳过,否则将目录项名加上路径后得到目录项的完整路径 fullpath,并通过 stat 函数获取文件状态信息。如果是目录则输出目录信息并递归调用 traverseDir 函数遍历子目录,否则输出文件信息。最后使用 closedir 函数关闭目录。
阅读全文
相关推荐
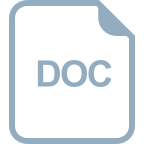
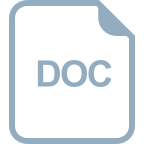

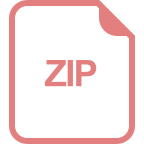
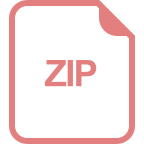












