java实现2_3|8类型的假分数的加减乘除运算
时间: 2024-05-05 15:16:40 浏览: 11
以下是Java实现2_3|8类型的假分数的加减乘除运算的示例代码:
```java
public class MixedFraction {
private int integer; // 整数部分
private int numerator; // 分子
private int denominator; // 分母
// 构造函数
public MixedFraction(int integer, int numerator, int denominator) {
this.integer = integer;
this.numerator = numerator;
this.denominator = denominator;
}
// 加法
public MixedFraction add(MixedFraction other) {
int lcm = lcm(this.denominator, other.denominator); // 最小公倍数
int newNumerator1 = this.numerator * (lcm / this.denominator);
int newNumerator2 = other.numerator * (lcm / other.denominator);
int sum = newNumerator1 + newNumerator2;
return simplify(new MixedFraction(0, sum, lcm));
}
// 减法
public MixedFraction subtract(MixedFraction other) {
int lcm = lcm(this.denominator, other.denominator); // 最小公倍数
int newNumerator1 = this.numerator * (lcm / this.denominator);
int newNumerator2 = other.numerator * (lcm / other.denominator);
int diff = newNumerator1 - newNumerator2;
return simplify(new MixedFraction(0, diff, lcm));
}
// 乘法
public MixedFraction multiply(MixedFraction other) {
int newNumerator = (this.integer * this.denominator + this.numerator) * (other.integer * other.denominator + other.numerator);
int newDenominator = this.denominator * other.denominator;
return simplify(new MixedFraction(0, newNumerator, newDenominator));
}
// 除法
public MixedFraction divide(MixedFraction other) {
int newNumerator = (this.integer * this.denominator + this.numerator) * other.denominator;
int newDenominator = (other.integer * other.denominator + other.numerator) * this.denominator;
return simplify(new MixedFraction(0, newNumerator, newDenominator));
}
// 约分
private MixedFraction simplify(MixedFraction fraction) {
int gcd = gcd(fraction.numerator, fraction.denominator);
int newNumerator = fraction.numerator / gcd;
int newDenominator = fraction.denominator / gcd;
int newInteger = newNumerator / newDenominator;
newNumerator = newNumerator % newDenominator;
return new MixedFraction(newInteger, newNumerator, newDenominator);
}
// 求最大公约数
private int gcd(int a, int b) {
if (b == 0) {
return a;
} else {
return gcd(b, a % b);
}
}
// 求最小公倍数
private int lcm(int a, int b) {
return a * b / gcd(a, b);
}
// 输出假分数
public String toString() {
if (this.integer == 0 && this.numerator == 0) {
return "0";
} else if (this.integer == 0) {
return this.numerator + "/" + this.denominator;
} else if (this.numerator == 0) {
return "" + this.integer;
} else {
return this.integer + "_" + this.numerator + "/" + this.denominator;
}
}
}
```
示例用法:
```java
MixedFraction f1 = new MixedFraction(2, 1, 4); // 2_1/4
MixedFraction f2 = new MixedFraction(3, 2, 8); // 3_2/8
MixedFraction sum = f1.add(f2); // 加法
MixedFraction diff = f1.subtract(f2); // 减法
MixedFraction product = f1.multiply(f2); // 乘法
MixedFraction quotient = f1.divide(f2); // 除法
System.out.println(f1 + " + " + f2 + " = " + sum); // 输出:2_1/4 + 3_2/8 = 5_1/8
System.out.println(f1 + " - " + f2 + " = " + diff); // 输出:2_1/4 - 3_2/8 = 1_5/8
System.out.println(f1 + " * " + f2 + " = " + product); // 输出:2_1/4 * 3_2/8 = 1_1/8
System.out.println(f1 + " / " + f2 + " = " + quotient); // 输出:2_1/4 / 3_2/8 = 1_3/4
```
相关推荐
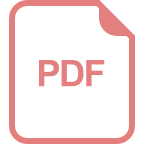
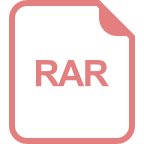














