shapely.unary.union
时间: 2024-04-29 12:20:05 浏览: 408
`shapely.unary_union` 是 Shapely 库中的一个函数,用于将一组几何对象合并为单个对象。具体而言,它将多边形、线、点等对象组成的集合合并为一个最小的几何对象,其边界为所有输入对象的联合边界。这个函数的调用方式为 `unary_union(geometry)`,其中 `geometry` 是一个几何对象的列表或集合。例如,如果你有两个多边形对象 `poly1` 和 `poly2`,你可以使用以下代码将它们合并为单个对象:
```python
from shapely.geometry import Polygon
from shapely.ops import unary_union
poly1 = Polygon([(0, 0), (0, 1), (1, 1), (1, 0)])
poly2 = Polygon([(1, 0), (1, 1), (2, 1), (2, 0)])
union_poly = unary_union([poly1, poly2])
```
`union_poly` 将是一个包含两个多边形的联合边界的对象。此函数对于空间分析和地理信息系统应用非常有用。
相关问题
Traceback (most recent call last): File "E:\code-study\coda\cross_nostopline.py", line 53, in <module> polygon = unary_union(rectangles) File "C:\Users\GW00321286\.conda\envs\python_39\lib\site-packages\shapely\ops.py", line 135, in unary_union return shapely.union_all(geoms, axis=None) File "C:\Users\GW00321286\.conda\envs\python_39\lib\site-packages\shapely\decorators.py", line 77, in wrapped return func(*args, **kwargs) File "C:\Users\GW00321286\.conda\envs\python_39\lib\site-packages\shapely\set_operations.py", line 413, in union_all collections = lib.create_collection(geometries, GeometryType.GEOMETRYCOLLECTION) TypeError: One of the arguments is of incorrect type. Please provide only Geometry objects.
这个错误的原因是你传递给unary_union方法的rectangles不是纯几何对象,而是GeoDataFrame中的几何列,因此需要从GeoDataFrame中提取几何列,然后再进行unary_union操作。修改代码如下:
```python
import geopandas as gpd
from shapely.ops import unary_union
# 读取GeoDataFrame
gdf = gpd.read_file('your_file.shp')
# 提取几何列
geometries = gdf.geometry.values
# 计算几何对象的并集
union_geometry = unary_union(geometries)
# 将并集几何对象转换为GeoDataFrame
union_gdf = gpd.GeoDataFrame(geometry=[union_geometry])
# 保存为文件
union_gdf.to_file('output.shp')
```
注意,这里的输出文件会是一个包含一个几何对象的GeoDataFrame,而不是原始数据的GeoDataFrame。
File "E:\code-study\coda\crossing\cross_test.py", line 105, in <module> extended_polygon = extend_polygon_edges(polygon, 5) File "E:\code-study\coda\crossing\cross_test.py", line 93, in extend_polygon_edges start_point = translate(point1, dx_normalized, dy_normalized) File "C:\Users\GW00321286\.conda\envs\python_39\lib\site-packages\shapely\affinity.py", line 241, in translate if geom.is_empty: AttributeError: 'tuple' object has no attribute 'is_empty'
非常抱歉给你带来困扰,我之前的回答中有一个错误。在 Shapely 库中,`translate` 函数的第一个参数应该是几何对象,而不是坐标点。因此,在使用 `translate` 函数之前,我们需要将起始点和结束点转换为 Point 对象。
以下是修正后的示例代码:
```python
from shapely.geometry import Polygon, LineString, Point
from shapely.ops import unary_union
from shapely.affinity import translate
def extend_polygon_edges(polygon, length):
# 获取多边形的边界线
boundary = polygon.boundary
# 初始化新的多边形
new_polygon = Polygon()
# 处理每条边界线
for line in boundary:
# 计算线段的单位向量
dx = line.coords[1][0] - line.coords[0][0]
dy = line.coords[1][1] - line.coords[0][1]
length_normalized = length / (dx**2 + dy**2)**0.5
dx_normalized = dx * length_normalized
dy_normalized = dy * length_normalized
# 创建新的起始点和结束点
start_point = translate(Point(line.coords[0]), dx_normalized, dy_normalized)
end_point = translate(Point(line.coords[1]), -dx_normalized, -dy_normalized)
# 将新的起始点、边界线、结束点依次添加到新的多边形中
new_polygon = unary_union([new_polygon, LineString([start_point, line, end_point])])
return new_polygon
# 创建一个不规则形状的多边形示例
polygon = Polygon([(0, 0), (1, 1), (2, 0), (1, -1)])
# 扩展多边形的边界线
extended_polygon = extend_polygon_edges(polygon, 5)
# 打印扩展后的多边形的顶点坐标
for vertex in extended_polygon.exterior.coords:
print(vertex)
```
这段代码会将起始点和结束点转换为 Point 对象,并使用 `translate` 函数对它们进行平移。然后,我们将新的起始点、边界线和结束点依次添加到新的多边形中,并打印扩展后的多边形的顶点坐标。
再次对之前的错误表示歉意,希望这个修正能够帮到你。如果还有其他问题,请随时提问。
阅读全文
相关推荐
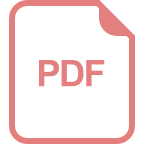
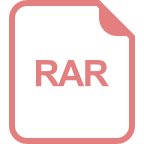
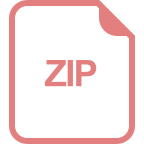













