用qt实现串口的收发数据
时间: 2024-05-09 21:16:47 浏览: 11
Qt提供了QSerialPort类来实现串口的收发数据。下面是一个简单的例子,演示了如何使用QSerialPort类。
```cpp
#include <QtSerialPort/QSerialPort>
#include <QtSerialPort/QSerialPortInfo>
#include <QDebug>
int main()
{
// 枚举可用的串口
foreach(const QSerialPortInfo &info, QSerialPortInfo::availablePorts())
{
qDebug() << "Name: " << info.portName();
qDebug() << "Description: " << info.description();
qDebug() << "Manufacturer: " << info.manufacturer();
}
// 打开串口
QSerialPort serial;
serial.setPortName("COM1"); // 设置串口名称
serial.setBaudRate(QSerialPort::Baud115200); // 设置波特率
serial.setDataBits(QSerialPort::Data8); // 设置数据位
serial.setParity(QSerialPort::NoParity); // 设置校验位
serial.setStopBits(QSerialPort::OneStop); // 设置停止位
serial.setFlowControl(QSerialPort::NoFlowControl); // 设置流控制
if (!serial.open(QIODevice::ReadWrite))
{
qDebug() << "Failed to open serial port";
return -1;
}
// 发送数据
QByteArray data = "Hello, world!";
qint64 bytesWritten = serial.write(data);
if (bytesWritten == -1)
{
qDebug() << "Failed to write the data to the serial port";
return -1;
}
// 接收数据
QByteArray responseData;
while (serial.waitForReadyRead(1000))
{
responseData += serial.readAll();
}
qDebug() << "Response: " << responseData;
// 关闭串口
serial.close();
return 0;
}
```
在这个例子中,我们首先使用QSerialPortInfo类枚举可用的串口。然后我们创建一个QSerialPort对象,设置串口的属性,打开串口并发送数据。最后我们从串口接收数据,关闭串口。
相关推荐
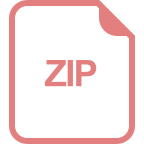














