#ifndef POINT_H#define POINT_Hclass Point {public: Point(double x = 0, double y = 0, double z = 0); double getX() const; double getY() const; double getZ() const;private: double x, y, z;};#endif
时间: 2023-11-20 07:07:17 浏览: 33
这是一个头文件 point.h,其中定义了一个名为 Point 的类,它有三个私有成员变量 x、y、z,分别表示三维空间中的坐标。类中有一个公有构造函数,可以传递三个参数,分别表示 x、y、z 坐标的值。另外,类中还有三个公有成员函数,分别为 getX()、getY()、getZ(),用来获取 x、y、z 坐标的值。
相关问题
(1)声明Point(点)类,由Point类派生出Circle(圆)类,再由Circle类派生出Cylinder(圆柱体)类。将类的定义部分分别作为3个头文件,对它们的成员函数的声明部分分别作为3个源文件(.cpp文件),在主函数中用#include命令把它们包含进来,形
Point.h头文件定义:
```cpp
#ifndef POINT_H
#define POINT_H
class Point {
public:
Point(double x = 0, double y = 0);
double getX() const;
double getY() const;
void setX(double x);
void setY(double y);
private:
double x;
double y;
};
#endif
```
Point.cpp源文件实现:
```cpp
#include "Point.h"
Point::Point(double x, double y) {
this->x = x;
this->y = y;
}
double Point::getX() const {
return x;
}
double Point::getY() const {
return y;
}
void Point::setX(double x) {
this->x = x;
}
void Point::setY(double y) {
this->y = y;
}
```
Circle.h头文件定义:
```cpp
#ifndef CIRCLE_H
#define CIRCLE_H
#include "Point.h"
class Circle : public Point {
public:
Circle(double x = 0, double y = 0, double r = 0);
double getR() const;
void setR(double r);
double area() const;
private:
double r;
};
#endif
```
Circle.cpp源文件实现:
```cpp
#include "Circle.h"
#include <cmath>
Circle::Circle(double x, double y, double r) : Point(x, y) {
this->r = r;
}
double Circle::getR() const {
return r;
}
void Circle::setR(double r) {
this->r = r;
}
double Circle::area() const {
return M_PI * r * r;
}
```
Cylinder.h头文件定义:
```cpp
#ifndef CYLINDER_H
#define CYLINDER_H
#include "Circle.h"
class Cylinder : public Circle {
public:
Cylinder(double x = 0, double y = 0, double r = 0, double h = 0);
double getH() const;
void setH(double h);
double volume() const;
private:
double h;
};
#endif
```
Cylinder.cpp源文件实现:
```cpp
#include "Cylinder.h"
#include <cmath>
Cylinder::Cylinder(double x, double y, double r, double h) : Circle(x, y, r) {
this->h = h;
}
double Cylinder::getH() const {
return h;
}
void Cylinder::setH(double h) {
this->h = h;
}
double Cylinder::volume() const {
return area() * h;
}
```
主函数中的头文件包含:
```cpp
#include "Point.h"
#include "Circle.h"
#include "Cylinder.h"
```
定义一个Point类,在此基础上,定义一个“Circle”派生类,要求:新增一个半径成员;能计算并输出圆的周长及加圆面积(类的定义保存在头文件中,测试程序用exp_405.cpp)。
好的,我明白了你的要求。以下是Point类和Circle类的定义:
```cpp
// Point.h
#ifndef POINT_H
#define POINT_H
class Point {
public:
Point(double x = 0.0, double y = 0.0) : x_(x), y_(y) {}
virtual ~Point() {}
double getX() const { return x_; }
double getY() const { return y_; }
void setX(double x) { x_ = x; }
void setY(double y) { y_ = y; }
private:
double x_, y_;
};
#endif // POINT_H
```
```cpp
// Circle.h
#ifndef CIRCLE_H
#define CIRCLE_H
#include "Point.h"
class Circle : public Point {
public:
Circle(double x = 0.0, double y = 0.0, double radius = 0.0)
: Point(x, y), radius_(radius) {}
virtual ~Circle() {}
double getRadius() const { return radius_; }
void setRadius(double radius) { radius_ = radius; }
double getCircumference() const { return 2 * 3.14159 * radius_; }
double getArea() const { return 3.14159 * radius_ * radius_; }
private:
double radius_;
};
#endif // CIRCLE_H
```
测试程序exp_405.cpp如下:
```cpp
#include <iostream>
#include "Circle.h"
using namespace std;
int main() {
Circle c(1.0, 2.0, 3.0);
cout << "Center: (" << c.getX() << ", " << c.getY() << ")" << endl;
cout << "Radius: " << c.getRadius() << endl;
cout << "Circumference: " << c.getCircumference() << endl;
cout << "Area: " << c.getArea() << endl;
return 0;
}
```
注意,为了计算圆的周长和面积,Circle类需要新增一个半径成员变量。getRadius()和setRadius()函数分别用于获取和设置半径。getCircumference()和getArea()函数分别用于计算周长和面积。测试程序中,我们创建了一个Circle对象c,并输出其圆心坐标、半径、周长和面积。
相关推荐
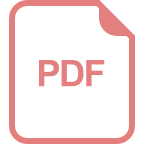












