names = {k: v for k, v in enumerate(model.names if hasattr(model, 'names') else model.module.names)}
时间: 2024-05-22 21:10:54 浏览: 13
这段代码是将模型中的名称信息存储在一个字典中,字典的键是名称的索引,字典的值是名称本身。具体来说,它首先检查模型中是否有名为“names”的属性,如果有,则使用该属性作为名称列表。否则,它假定模型是一个nn.DataParallel对象,并尝试使用模型的“module”属性来获取名称列表。最后,它使用enumerate函数将索引和名称对应起来,并将它们存储在字典中。
相关问题
yolov5中的DetectionModel代码是什么
DetectionModel是YOLOv5中的一个Python类,它位于yolov5/models/yolo.py文件中。
下面是DetectionModel类的代码:
```python
class DetectionModel(nn.Module):
def __init__(self, cfg):
super(DetectionModel, self).__init__()
self.model = attempt_load(cfg['weights'], map_location=torch.device('cpu')) # load FP32 model
self.model.eval()
self.model.float()
self.stride = torch.tensor(self.model.stride).float()
self.classes = self.model.module.names if hasattr(self.model, 'module') else self.model.names
self.autoshape = self.model.module.autoshape if hasattr(self.model, 'module') else self.model.autoshape
self.set_precision(cfg['precision'])
def forward(self, img, augment=False, visualize=False):
# Inference
pred = self.model(img, augment=augment, visualize=visualize)[0]
# Apply NMS
pred = non_max_suppression(pred, conf_thres=self.conf, iou_thres=self.iou, classes=self.classes,
agnostic=self.agnostic_nms, max_det=self.max_det)
# Process detections
for i, det in enumerate(pred): # detections per image
if self.training:
# Rescale boxes from img_size to relative 0-1
det[:, :4] /= self.img_size
det[:, :4] = scale_coords(img.shape[2:], det[:, :4], img.shape[2:]).round()
return pred
def set_precision(self, precision):
self.precision = precision
self.conf = 0.001 if precision == 'fp16' else 0.0001
self.iou = 0.65
self.agnostic_nms = False
self.max_det = 1000
if precision == 'int8':
self.model = torch.quantization.quantize_dynamic(
self.model, {torch.nn.Conv2d}, dtype=torch.qint8
)
self.model.qconfig = torch.quantization.get_default_qconfig('fbgemm')
self.model = torch.quantization.prepare(self.model)
self.model.eval()
```
该类接受一个配置字典作为参数,并从给定的权重加载YOLOv5模型。它还包含一个前向传递函数,该函数使用模型对图像进行推理并执行非最大抑制(NMS)以获取检测结果。此外,该类还包括设置模型精度(precision)的方法,以及一些与NMS相关的参数。
在YOLOv5的detect.py文件中如何加入检测FPS的代码
要在 YOLOv5 的 `detect.py` 文件中加入检测 FPS 的代码,可以在检测循环中记录每个图像的开始和结束时间,并根据时间差计算 FPS。以下是在 `detect.py` 文件中加入检测 FPS 的代码示例:
```python
import time
import cv2
import torch
from models.experimental import attempt_load
from utils.datasets import LoadStreams, LoadImages
from utils.general import check_img_size, non_max_suppression, apply_classifier, \
scale_coords, xyxy2xywh, plot_one_box, strip_optimizer, set_logging
from utils.torch_utils import select_device, load_classifier, time_synchronized
def detect(opt):
set_logging()
device = select_device(opt.device)
half = device.type != 'cpu' # half precision only supported on CUDA
# 加载模型
model = attempt_load(opt.weights, map_location=device) # load FP32 model
imgsz = check_img_size(opt.img_size, s=model.stride.max()) # check img_size
if half:
model.half() # to FP16
# 获取类别名称和颜色
names = model.module.names if hasattr(model, 'module') else model.names
colors = [[0, 255, 0]]
# 初始化摄像头或视频流
dataset = LoadStreams(opt.source, img_size=imgsz)
fps = dataset.cap.get(cv2.CAP_PROP_FPS) # 获取帧率
# 循环检测
total_time = 0.0
num_frames = 0
for path, img, im0s, vid_cap in dataset:
t1 = time_synchronized()
# 图像预处理
img = torch.from_numpy(img).to(device)
img = img.half() if half else img.float()
img /= 255.0
if img.ndimension() == 3:
img = img.unsqueeze(0)
# 模型推理
t2 = time_synchronized()
pred = model(img, augment=opt.augment)[0]
# 后处理
t3 = time_synchronized()
pred = non_max_suppression(pred, opt.conf_thres, opt.iou_thres, classes=opt.classes, agnostic=opt.agnostic_nms)
for i, det in enumerate(pred):
if len(det):
det[:, :4] = scale_coords(img.shape[2:], det[:, :4], im0s.shape).round()
for *xyxy, conf, cls in reversed(det):
c = int(cls)
label = f'{names[c]} {conf:.2f}'
plot_one_box(xyxy, im0s, label=label, color=colors[c], line_thickness=3)
# 显示图像
t4 = time_synchronized()
cv2.imshow('YOLOv5', im0s)
# 计算 FPS
t5 = time_synchronized()
num_frames += 1
total_time += t5 - t1
fps = num_frames / total_time
# 按 'q' 键退出
if cv2.waitKey(1) == ord('q'):
break
# 释放资源
cv2.destroyAllWindows()
dataset.stop()
# 输出 FPS
print(f'FPS: {fps:.2f}')
```
在上面的代码中,我们使用 `time_synchronized()` 函数记录每个步骤的开始和结束时间,并在循环结束时计算 FPS。我们还使用 OpenCV 的 `cv2.imshow()` 来显示图像。请注意,这里的 `fps` 变量是每个图像的 FPS,而不是全局 FPS。如果您需要计算全局 FPS,请计算所有图像的平均 FPS。
相关推荐
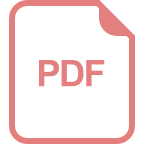
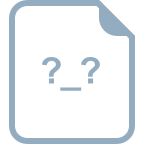
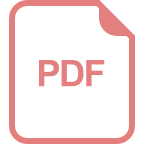












