使用Python读取Arduino串口实时显示数据并绘图,且设置一个循环队列,数据进入后先去队列排队,再定时器调用函数,同时能将历史数据(时间和电压)保存为csv格式的代码
时间: 2023-06-10 09:04:01 浏览: 210
可以尝试如下Python代码实现:
```python
import serial
import time
import csv
import matplotlib.pyplot as plt
# 设置串口参数:port、baudrate等
ser = serial.Serial('COM3', 9600, timeout=1)
# 初始化循环队列
queue_length = 10
voltage_queue = [0] * queue_length
# 定义定时器调用的函数
def draw_chart():
# 获取当前时间及电压
current_time = time.strftime('%Y-%m-%d %H:%M:%S', time.localtime())
voltage = ser.readline().strip().decode()
# 将当前数据放入循环队列中
voltage_queue.pop(0)
voltage_queue.append(float(voltage))
# 保存历史数据为csv格式
with open('data.csv', 'a', newline='') as csvfile:
writer = csv.writer(csvfile)
writer.writerow([current_time, voltage])
# 绘制实时图像
plt.plot(voltage_queue)
plt.xlabel('Time')
plt.ylabel('Voltage(V)')
plt.title('Real-time Plot')
plt.show()
# 每隔5秒钟调用一次画图函数
while True:
t1 = time.time()
draw_chart()
t2 = time.time()
time.sleep(max(5 - (t2 - t1), 0))
```
以上是读取Arduino串口实时显示数据并绘图、保存历史数据为csv格式的Python代码,具体功能如下:
1. 初始化串口,设置串口号及波特率等参数;
2. 初始化循环队列,用于存储最近10次电压数据;
3. 定义画图函数,其中会读取串口中最新的一次数据,将其放入循环队列中,保存历史数据到csv文件中,并绘制实时图像;
4. 在主循环中,每隔5秒钟调用一次画图函数。
阅读全文
相关推荐
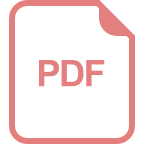
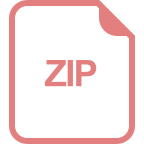
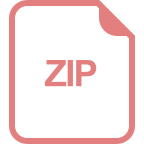















