用c语言写一个低通滤波器
时间: 2023-04-06 21:01:59 浏览: 210
可以使用C语言中的数字信号处理库(DSP库)来实现低通滤波器。以下是一个简单的示例代码:
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#include <dsp.h>
#define SAMPLE_RATE 44100
#define CUTOFF_FREQ 1000
int main()
{
// 初始化DSP库
dsp_init();
// 计算滤波器系数
float cutoff = CUTOFF_FREQ / (float)SAMPLE_RATE;
float b[3], a[3];
dsp_biquad_lowpass(cutoff, 0.707, b, a);
// 读取输入音频文件
FILE *input_file = fopen("input.wav", "rb");
if (input_file == NULL) {
printf("无法打开输入文件\n");
return 1;
}
// 创建输出音频文件
FILE *output_file = fopen("output.wav", "wb");
if (output_file == NULL) {
printf("无法创建输出文件\n");
return 1;
}
// 读取音频文件头
wav_header_t header;
fread(&header, sizeof(header), 1, input_file);
fwrite(&header, sizeof(header), 1, output_file);
// 处理音频数据
int16_t sample;
float x[3] = {0}, y[3] = {0};
while (fread(&sample, sizeof(sample), 1, input_file) == 1) {
// 将采样值转换为浮点数
float x0 = sample / 32768.0;
// 应用滤波器
float y0 = dsp_biquad(x0, b, a, x, y);
// 将浮点数转换为采样值
sample = round(y0 * 32768.0);
// 写入输出文件
fwrite(&sample, sizeof(sample), 1, output_file);
}
// 关闭文件
fclose(input_file);
fclose(output_file);
return 0;
}
阅读全文
相关推荐
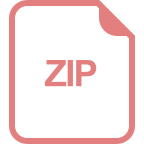

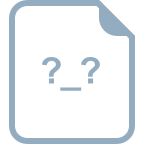


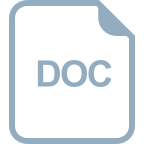
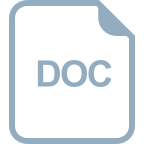




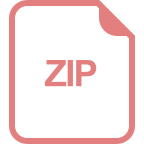



