用tensorflow对图像进行空间注意力机制,重点关注某些特征,相关代码
时间: 2023-07-04 08:27:23 浏览: 67
以下是使用TensorFlow实现空间注意力机制的代码,可以重点关注某些特征:
```python
import tensorflow as tf
class SpatialAttention(tf.keras.layers.Layer):
def __init__(self, num_filters):
super(SpatialAttention, self).__init__()
# Convolutional layers to extract features from the input image
self.conv1 = tf.keras.layers.Conv2D(filters=num_filters, kernel_size=3, strides=1, padding='same', activation='relu')
self.conv2 = tf.keras.layers.Conv2D(filters=num_filters, kernel_size=3, strides=1, padding='same', activation='relu')
# Convolutional layers to extract the spatial attention map
self.conv3 = tf.keras.layers.Conv2D(filters=1, kernel_size=3, strides=1, padding='same')
self.conv4 = tf.keras.layers.Conv2D(filters=1, kernel_size=3, strides=1, padding='same')
# Pooling layer to reduce the size of the spatial attention map
self.pool = tf.keras.layers.MaxPool2D(pool_size=(2, 2), strides=(2, 2), padding='same')
def call(self, inputs):
# Extract features from the input image
x = self.conv1(inputs)
x = self.conv2(x)
# Compute the spatial attention map
attn_map = self.conv3(x)
attn_map = self.pool(attn_map)
attn_map = self.conv4(attn_map)
attn_map = tf.nn.sigmoid(attn_map)
# Apply the attention map to the input image
x = tf.multiply(inputs, attn_map)
return x
```
以上代码实现了一个SpatialAttention层,可以在神经网络中使用空间注意力机制来重点关注某些特征。在模型的forward中,需要传入待加权的inputs,其中inputs的shape为[N, H, W, C],表示batch大小、图片高度、图片宽度和通道数。模型输出的shape也为[N, H, W, C],表示加权后的图片。
具体实现中,我们使用两个卷积层来从输入图片中提取特征,然后通过两个卷积层提取空间注意力图。最后,我们使用最大池化层来缩小空间注意力图的大小,并使用Sigmoid函数将其值映射到[0,1]之间。最后,我们将输入图片和空间注意力图相乘,得到加权后的图片。
相关推荐
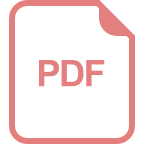
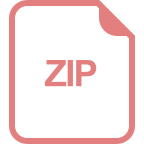














