用澳大利亚信贷批准数据集中的690个样本作为数据集,通过python代码分别用线性逻辑回归和非线性随机森林构建识别模型,进行特征选择并用准确度召回率混淆矩阵等指标对结果进行评估
时间: 2024-03-08 20:47:19 浏览: 256
好的,让我来回答你的问题。你想知道如何使用Python代码对澳大利亚信贷批准数据集进行线性逻辑回归和非线性随机森林分类,并进行特征选择,最后使用准确度、召回率和混淆矩阵等指标来评估模型的性能,是吗?
以下是一个大致的流程:
1. 加载数据集并进行数据预处理
首先,你需要加载数据集并进行一些简单的数据预处理,如缺失值填充和特征标准化。以下是一个示例代码:
``` python
import pandas as pd
from sklearn.preprocessing import StandardScaler
# 加载数据集
df = pd.read_csv('australian.csv')
# 填充缺失值
df.fillna(df.mean(), inplace=True)
# 特征标准化
scaler = StandardScaler()
X = scaler.fit_transform(df.iloc[:, :-1])
y = df.iloc[:, -1].values
```
2. 构建线性逻辑回归模型并进行特征选择
你可以使用Scikit-learn库中的逻辑回归算法来构建线性分类模型,并使用L1或L2正则化来进行特征选择。以下是一个示例代码:
``` python
from sklearn.linear_model import LogisticRegression
from sklearn.feature_selection import SelectFromModel
# 构建逻辑回归模型并进行特征选择
lr = LogisticRegression(penalty='l1', solver='liblinear', random_state=42)
selector = SelectFromModel(lr)
X_new = selector.fit_transform(X, y)
```
3. 构建非线性随机森林模型并进行特征选择
你可以使用Scikit-learn库中的随机森林算法来构建非线性分类模型,并使用基于特征重要性的方法来进行特征选择。以下是一个示例代码:
``` python
from sklearn.ensemble import RandomForestClassifier
from sklearn.feature_selection import SelectFromModel
# 构建随机森林模型并进行特征选择
rf = RandomForestClassifier(n_estimators=100, random_state=42)
selector = SelectFromModel(rf)
X_new = selector.fit_transform(X, y)
```
4. 评估模型性能
最后,你可以使用准确度、召回率和混淆矩阵等指标来评估模型的性能。以下是一个示例代码:
``` python
from sklearn.metrics import confusion_matrix, accuracy_score, recall_score
from sklearn.model_selection import train_test_split
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X_new, y, test_size=0.3, random_state=42)
# 构建逻辑回归模型并评估
lr = LogisticRegression(random_state=42)
lr.fit(X_train, y_train)
y_pred_lr = lr.predict(X_test)
print("逻辑回归模型的混淆矩阵:\n", confusion_matrix(y_test, y_pred_lr))
print("逻辑回归模型的准确度:", accuracy_score(y_test, y_pred_lr))
print("逻辑回归模型的召回率:", recall_score(y_test, y_pred_lr))
# 构建随机森林模型并评估
rf = RandomForestClassifier(n_estimators=100, random_state=42)
rf.fit(X_train, y_train)
y_pred_rf = rf.predict(X_test)
print("随机森林模型的混淆矩阵:\n", confusion_matrix(y_test, y_pred_rf))
print("随机森林模型的准确度:", accuracy_score(y_test, y_pred_rf))
print("随机森林模型的召回率:", recall_score(y_test, y_pred_rf))
```
请注意,以上代码仅是一个示例,你可能需要根据具体情况进行更改和调整。
阅读全文
相关推荐
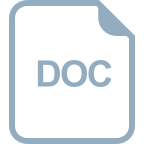
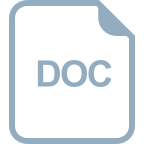
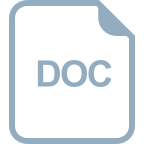



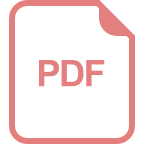
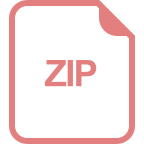
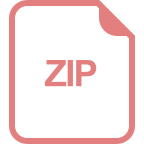
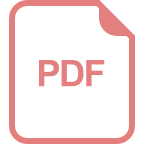
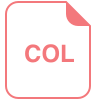
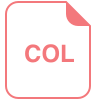
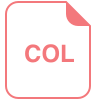
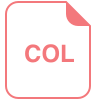




