linux发送数据给串口 cpp代码实现
时间: 2023-07-25 09:17:06 浏览: 49
以下是一个简单的 C++ 代码示例,用于向 Linux 中的串口发送数据:
```c++
#include <iostream>
#include <fcntl.h>
#include <termios.h>
#include <unistd.h>
int main()
{
int fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY | O_NDELAY);
if (fd == -1)
{
std::cout << "Error: Unable to open serial port." << std::endl;
return -1;
}
struct termios options;
tcgetattr(fd, &options);
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
options.c_cflag |= (CLOCAL | CREAD);
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
options.c_cflag &= ~CRTSCTS;
options.c_lflag &= ~(ICANON | ECHO | ECHOE | ISIG);
options.c_iflag &= ~(IXON | IXOFF | IXANY);
options.c_oflag &= ~OPOST;
options.c_cc[VMIN] = 0;
options.c_cc[VTIME] = 10;
tcsetattr(fd, TCSANOW, &options);
char data[] = "Hello, world!";
int len = sizeof(data) - 1;
int written = write(fd, data, len);
if (written != len)
{
std::cout << "Error: Unable to write to serial port." << std::endl;
return -1;
}
close(fd);
return 0;
}
```
在上面的示例中,我们首先使用 `open()` 函数打开 `/dev/ttyS0` 设备文件,该文件是 Linux 中的串口设备文件。我们需要设置一些选项,例如波特率、数据位、停止位等等,使串口与设备之间的通信正常工作。然后,我们将数据写入串口,最后关闭串口。
相关推荐
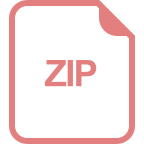
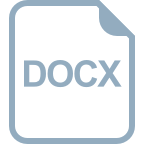














