vector<pair<int, int> > next_moves = generate_next_moves(); for (auto& next_move : next_moves) { int x = next_move.first; int y = next_move.second; gridInfo[x][y] = color; // 模拟落子 int score = -alpha_beta(depth - 1, -beta, -alpha, -color); // 递归搜索 gridInfo[x][y] = 0; // 撤销落子 if (score > best_score) { best_score = score; if (best_score > alpha) { alpha = best_score; } if (best_score >= beta) { break; // β剪枝 } } }你能和我解释一下next_move和bext_moves的区别吗
时间: 2024-02-14 08:08:22 浏览: 32
当代码中出现 `next_moves` 时,它是一个存储下一步可行落子位置的向量容器,每个元素是一个 `pair<int, int>` 类型的坐标,表示下一步可落子的位置。而 `next_move` 则是 `next_moves` 容器中的一个元素,也就是一个落子位置的坐标。在循环中,程序会遍历 `next_moves` 容器中的所有元素,对每个落子位置进行模拟落子、递归搜索、撤销落子等操作,以获取最优解。
相关问题
int alpha_beta_next(int depth, int alpha, int beta, int color) { if (depth == 0) { return evaluate(currBotColor); } vector<pair<int, int>> next_moves = generate_next_moves(); for (auto& next_move : next_moves) { int x = next_move.first; int y = next_move.second; gridInfo[x][y] = color; int score = -alpha_beta_next(depth - 1, -beta, -alpha, -color); gridInfo[x][y] = 0; if (score >= beta) { return score; } if (score > alpha) { alpha = score; } } return alpha; } int alpha_beta_two_steps(int depth, int alpha, int beta, int color) { if (depth == 0) { return evaluate(currBotColor); } vector<pair<int, int>> next_moves = generate_next_moves(); for (auto& next_move : next_moves) { int x1 = next_move.first; int y1 = next_move.second; gridInfo[x1][y1] = color; vector<pair<int, int>> next_moves2 = generate_next_moves(); for (auto& next_move2 : next_moves2) { int x2 = next_move2.first; int y2 = next_move2.second; gridInfo[x2][y2] = color; int score = -alpha_beta_two_steps(depth - 2, -beta, -alpha, -color); gridInfo[x2][y2] = 0; if (score >= beta) { gridInfo[x1][y1] = 0; return score; } if (score > alpha) { alpha = score; } } gridInfo[x1][y1] = 0; } return alpha; }在主函数中怎么调用
在主函数中,你可以先调用 generate_next_moves() 函数生成下一步可行的落子位置,然后根据你的需求选择使用 alpha_beta_next() 函数还是 alpha_beta_two_steps() 函数。调用时需要传入相应的参数,如下:
// 调用 alpha_beta_next()
int score = alpha_beta_next(depth, alpha, beta, currBotColor);
// 调用 alpha_beta_two_steps()
int score = alpha_beta_two_steps(depth, alpha, beta, currBotColor);
其中,depth 为搜索深度,alpha 和 beta 为剪枝过程中的参数,currBotColor 为当前自己的颜色。调用结束后,score 为搜索得到的最优分数。
vector<pair<int, int>> next_moves1 = generate_next_moves(); int best_score = INT_MIN; for (auto& next_move1 : next_moves1) { int x1 = next_move1.first; int y1 = next_move1.second; gridInfo[x1][y1] = currBotColor; // 针对当前的第一步落子位置进行 alpha-beta 剪枝搜索 int score1 = -alpha_beta(SEARCH_DEPTH - 1, INT_MIN, INT_MAX, -currBotColor); if (score1 > best_score) { // 如果当前位置是历史最优,则在可行的第二步落子位置中搜索 best_score = score1; vector<pair<int, int>> next_moves2= generate_next_moves(); for (auto& next_move2 : next_moves2) { int x2 = next_move2.first; int y2 = next_move2.second; gridInfo[x2][y2] = currBotColor; // 针对当前的第二步落子位置进行 alpha-beta 剪枝搜索 int score2 = -alpha_beta(SEARCH_DEPTH - 2, best_score, INT_MAX, -currBotColor); if (score2 > best_score) { // 如果当前位置是历史最优,则更新历史最优位置为当前位置 best_score = score2; X1 = x1; Y1 = y1; X2 = x2; Y2 = y2; } gridInfo[x2][y2] = 0; } } gridInfo[x1][y1] = 0; } } cout << X1 << ' ' << Y1 << ' ' << X2 << ' ' << Y2 << endl;完善代码,加上考虑对手的
以下是加上考虑对手的代码:
```
vector<pair<int, int>> next_moves1 = generate_next_moves();
int best_score = INT_MIN;
for (auto& next_move1 : next_moves1) {
int x1 = next_move1.first;
int y1 = next_move1.second;
gridInfo[x1][y1] = currBotColor;
int score1 = -alpha_beta(SEARCH_DEPTH - 1, INT_MIN, INT_MAX, -currBotColor);
if (score1 > best_score) {
// 如果当前位置是历史最优,则在可行的第二步落子位置中搜索
best_score = score1;
vector<pair<int, int>> next_moves2 = generate_next_moves();
for (auto& next_move2 : next_moves2) {
int x2 = next_move2.first;
int y2 = next_move2.second;
gridInfo[x2][y2] = -currBotColor; // 对手的行动
int score2 = -alpha_beta(SEARCH_DEPTH - 2, -best_score, -score1, currBotColor); // 注意alpha和beta的符号要反转
if (score2 > best_score) {
// 如果当前位置是历史最优,则更新历史最优位置为当前位置
best_score = score2;
X1 = x1;
Y1 = y1;
X2 = x2;
Y2 = y2;
}
gridInfo[x2][y2] = 0;
}
}
gridInfo[x1][y1] = 0;
}
cout << X1 << ' ' << Y1 << ' ' << X2 << ' ' << Y2 << endl;
```
在第二步搜索中,我们将对手的行动标记为相反的颜色,即 `-currBotColor`,并且在调用 `alpha_beta` 函数时,将传入的 `alpha` 和 `beta` 参数的符号取反,以反转搜索结果的最大值和最小值。具体来说,我们将 `alpha_beta(SEARCH_DEPTH - 2, best_score, INT_MAX, -currBotColor)` 改为 `-alpha_beta(SEARCH_DEPTH - 2, -best_score, -score1, currBotColor)`。这样,我们就能够在搜索过程中考虑对手的行动了。
相关推荐
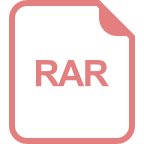
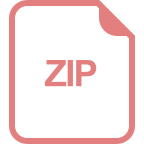




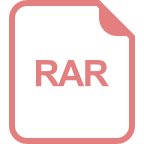
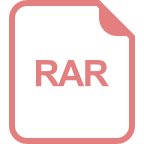
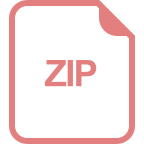
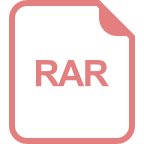
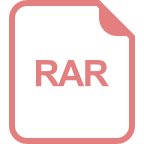
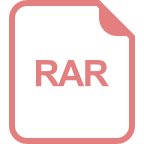