m = self.model[-1] # Detect() if isinstance(m, Detect): s = 128 # 2x min stride m.stride = torch.tensor([s / x.shape[-2] for x in self.forward(torch.zeros(1, ch, s, s))]) # forward m.anchors /= m.stride.view(-1, 1, 1) check_anchor_order(m) self.stride = m.stride self._initialize_biases()
时间: 2024-02-14 10:32:18 浏览: 27
这段代码出现在一个目标检测模型的类中,用于初始化模型的一些参数。首先,通过`self.model[-1]`获取模型的最后一层,判断这层是否为`Detect`类型。如果是,则将`stride`属性设置为一个长度为3的张量,其值为2倍的最小步长(`s=128`)除以输入张量的高度和宽度。然后,将`m.anchors`(anchors是目标检测中用于生成候选框的参考框)除以步长,以便与特征图上的网格对应。接下来,调用`check_anchor_order(m)`检查anchors的顺序是否正确。最后,调用`self._initialize_biases()`初始化模型的偏置项。
相关问题
解释代码: def detect_cma(self): # pass model = self.model output_size = self.output_size # source = self.img2predict # file/dir/URL/glob, 0 for webcam imgsz = [640, 640] # inference size (pixels) conf_thres = 0.25 # confidence threshold iou_thres = 0.45 # NMS IOU threshold max_det = 1000 # maximum detections per image # device = self.device # cuda device, i.e. 0 or 0,1,2,3 or cpu view_img = False # show results save_txt = False # save results to *.txt save_conf = False # save confidences in --save-txt labels save_crop = False # save cropped prediction boxes nosave = False # do not save images/videos classes = None # filter by class: --class 0, or --class 0 2 3 agnostic_nms = False # class-agnostic NMS augment = False # ugmented inference visualize = False # visualize features line_thickness = 3 # bounding box thickness (pixels) hide_labels = False # hide labels hide_conf = False # hide confidences half = False # use FP16 half-precision inference dnn = False # use OpenCV DNN for ONNX inference source = str(self.vid_source) device = select_device(self.device) stride, names, pt, jit, onnx = model.stride, model.names, model.pt, model.jit, model.onnx imgsz = check_img_size(imgsz, s=stride) # check image size save_img = not nosave and not source.endswith('.txt') # save inference images
这段代码用于使用 YOLOv5 模型对图像或视频进行目标检测。它首先从类的属性中获取模型、输出大小、视频源等信息。然后设置了一些参数,如推理尺寸、置信度阈值、NMS IOU 阈值等。接着根据设备类型选择使用 CPU 还是 GPU 进行推理。最后,它检查图像的大小是否符合模型要求,设置是否保存推理结果图片,然后返回检测结果。
class ComputeLoss: sort_obj_iou = False # Compute losses def __init__(self, model, autobalance=False): device = next(model.parameters()).device # get model device h = model.hyp # hyperparameters # Define criteria BCEcls = nn.BCEWithLogitsLoss(pos_weight=torch.tensor([h['cls_pw']], device=device)) BCEobj = nn.BCEWithLogitsLoss(pos_weight=torch.tensor([h['obj_pw']], device=device)) # Class label smoothing https://arxiv.org/pdf/1902.04103.pdf eqn 3 self.cp, self.cn = smooth_BCE(eps=h.get('label_smoothing', 0.0)) # positive, negative BCE targets # Focal loss g = h['fl_gamma'] # focal loss gamma if g > 0: BCEcls, BCEobj = FocalLoss(BCEcls, g), FocalLoss(BCEobj, g) m = de_parallel(model).model[-1] # Detect() module self.balance = {3: [4.0, 1.0, 0.4]}.get(m.nl, [4.0, 1.0, 0.25, 0.06, 0.02]) # P3-P7 self.ssi = list(m.stride).index(16) if autobalance else 0 # stride 16 index self.BCEcls, self.BCEobj, self.gr, self.hyp, self.autobalance = BCEcls, BCEobj, 1.0, h, autobalance self.na = m.na # number of anchors self.nc = m.nc # number of classes self.nl = m.nl # number of layers self.anchors = m.anchors self.device = device这个代码什么意思
这段代码是定义了一个 ComputeLoss 类,用于计算 YOLOv5 模型的损失值。在初始化时,会根据模型的超参数定义不同的损失函数,包括分类损失 BCEcls、目标检测损失 BCEobj 和 Focal Loss。同时还对正负样本进行了平滑处理,以及为不同的特征层定义了不同的权重平衡。在计算损失时,会用到模型的输出以及一些超参数,最终返回损失值。
相关推荐
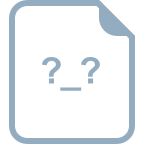












