用c语言编写程序 找出两个链表的公共结点 【问题描述】给定两个单链表,编写算法找出两个链表的公共结点。 【输入形式】 四行: 第一行:一个数字(第一个单链表中的元素个数) 第二行:第一个单链表中各个结点的值 第三行:一个数字(第二个单链表中的元素个数) 第四行:第二个单链表中各个结点的值 【输出形式】 两行: 第一行:两个单链表的公共元素个数 第二行:依次打印输出各个公共元素 【样例输入】 6 12 5 8 9 -23 16 4 16 21 9 3 【样例输出】 2 9 16
时间: 2023-05-29 22:04:55 浏览: 76
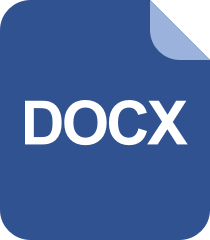
算法:给定一个链表,判断链表中是否存在环
#include <stdio.h>
#include <stdlib.h>
typedef struct node {
int value;
struct node* next;
} Node;
Node* createLinkedList(int n);
void printLinkedList(Node* head);
int getLength(Node* head);
Node* findFirstCommonNode(Node* head1, Node* head2);
int main() {
int n1, n2;
scanf("%d", &n1);
Node* head1 = createLinkedList(n1);
scanf("%d", &n2);
Node* head2 = createLinkedList(n2);
Node* commonNode = findFirstCommonNode(head1, head2);
int count = 0;
while (commonNode != NULL) {
count++;
commonNode = commonNode->next;
}
printf("%d\n", count);
commonNode = findFirstCommonNode(head1, head2);
while (commonNode != NULL) {
printf("%d ", commonNode->value);
commonNode = commonNode->next;
}
return 0;
}
Node* createLinkedList(int n) {
Node* head = NULL;
Node* current = NULL;
for (int i = 0; i < n; i++) {
int value;
scanf("%d", &value);
Node* node = (Node*) malloc(sizeof(Node));
node->value = value;
node->next = NULL;
if (head == NULL) {
head = node;
current = node;
} else {
current->next = node;
current = node;
}
}
return head;
}
void printLinkedList(Node* head) {
while (head != NULL) {
printf("%d ", head->value);
head = head->next;
}
printf("\n");
}
int getLength(Node* head) {
int length = 0;
while (head != NULL) {
length++;
head = head->next;
}
return length;
}
Node* findFirstCommonNode(Node* head1, Node* head2) {
int length1 = getLength(head1);
int length2 = getLength(head2);
if (length1 > length2) {
for (int i = 0; i < length1 - length2; i++) {
head1 = head1->next;
}
} else {
for (int i = 0; i < length2 - length1; i++) {
head2 = head2->next;
}
}
while (head1 != NULL && head2 != NULL && head1 != head2) {
head1 = head1->next;
head2 = head2->next;
}
return head1;
}
阅读全文
相关推荐
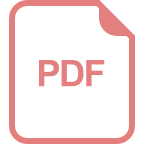
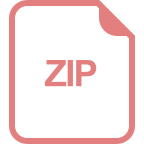















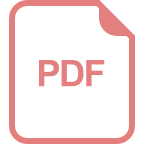