如何计算组合数的和,用C语言编写
时间: 2024-09-24 09:20:39 浏览: 53
在C语言中,计算组合数之和通常不是直接的数学运算,而是需要通过编程实现。组合数,即从n个不同元素中取出k个元素的不同组合数,可以用公式C(n, k) = n! / (k!(n-k)!)来表示,其中“!”表示阶乘。
对于大数值的组合数,可能会有溢出的问题,因此我们一般会使用动态规划或者递归的方法,但是为了效率,可以借助于一些数学库如`<math.h>`中的组合函数`组合`或者`comb`,如果您的系统支持的话。如果没有现成的库函数,你可以手动编写一个循环或递归算法来逐项累加组合数。
下面是一个简单的示例,展示如何使用递归来计算前n项的组合数之和:
```c
#include <stdio.h>
// 一个辅助函数计算组合数
unsigned long long combination(int n, int k) {
if (k == 0 || k == n)
return 1;
else
return n * combination(n - 1, k - 1) / k;
}
// 主函数计算组合数之和
unsigned long long combinations_sum(int n) {
unsigned long long sum = 0;
for (int i = 0; i <= n; ++i) {
sum += combination(n, i);
}
return sum;
}
int main() {
int n;
printf("Enter the value of n: ");
scanf("%d", &n);
unsigned long long result = combinations_sum(n);
printf("The sum of combinations from 0 to %d is: %llu\n", n, result);
return 0;
}
```
注意,这个程序可能在处理大值时会有性能问题,因为递归计算组合数会产生大量的重复计算。实际应用中,可能需要优化算法或者使用其他数据结构来存储中间结果。
阅读全文
相关推荐



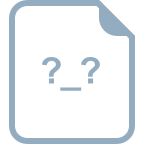














