使用C++实现计算一个简单表达式值。表达式包括正整数及零、圆括号和加减乘除4种运算符。建议步 骤:1)利用栈,将中缀表达式转换成后缀表达式;2)计算后缀表达式,从正整数及零数扩展到正实数
时间: 2024-10-23 17:15:20 浏览: 43
在C++中,我们可以使用栈数据结构来实现中缀表达式的转后缀(也称作逆波兰表示法,Reverse Polish Notation,RPN)以及后缀表达式的求值。以下是基本步骤:
1. **中缀表达式转后缀表达式**:
- 创建两个栈,一个用于操作数(stack_of_numbers),另一个用于操作符(stack_of_operators)。
- 遍历输入的中缀表达式,遇到数字直接压入操作数栈。
- 遇到左括号,压入栈。
- 遇到右括号,弹出操作符直到遇到左括号为止,并依次将其加入后缀表达式,然后弹出左括号。
- 遇到运算符,比较其优先级与当前栈顶操作符,如果小于等于则压入,大于则不断弹出并添加到后缀表达式,直到栈顶运算符优先级低于新运算符。
2. **计算后缀表达式**:
- 创建一个空的操作数栈(继续使用stack_of_numbers)。
- 遍历后缀表达式,对于每个元素,如果它是数字,则直接压入栈;如果是运算符,从栈中弹出足够次数的操作数进行计算(例如,`*`意味着前两个数相乘),并将结果压回栈。
- 最终栈内的唯一元素就是最终的结果。
示例C++代码片段:
```cpp
#include <iostream>
#include <stack>
#include <sstream>
std::string infixToRPN(std::string expression);
int evalPostfix(const std::string &postfix);
std::string precedence(char op) {
return op == '+' || op == '-' ? "0" : (op == '*' || op == '/' ? "1" : "2");
}
bool isOperator(char op) {
return op == '+' || op == '-' || op == '*' || op == '/';
}
std::string infixToRPN(std::string expression) {
std::stack<char> operators;
std::stringstream ss(expression);
std::string token;
std::string result;
while (ss >> token) {
if (!isOperator(token[0])) {
result += token + ' ';
} else {
while (!operators.empty() && precedence(operators.top()) >= precedence(token)) {
result += operators.top();
operators.pop();
}
operators.push(token[0]);
}
}
while (!operators.empty()) {
result += operators.top();
operators.pop();
}
return result;
}
int evalPostfix(const std::string &postfix) {
std::stack<int> stack;
for (char c : postfix) {
if (isdigit(c)) {
int num = 0;
while (isdigit(c)) {
num = num * 10 + (c - '0');
++c;
}
stack.push(num);
} else {
int b = stack.top(); stack.pop();
int a = stack.top(); stack.pop();
switch (c) {
case '+': stack.push(a + b); break;
case '-': stack.push(a - b); break;
case '*': stack.push(a * b); break;
case '/': stack.push(a / b); break;
}
}
}
return stack.top();
}
int main() {
std::string expression = "(1 + 2) * (3 - 4)";
std::string postfix = infixToRPN(expression);
int value = evalPostfix(postfix);
std::cout << "后缀表达式: " << postfix << "\n";
std::cout << "计算结果: " << value << "\n";
return 0;
}
```
阅读全文
相关推荐





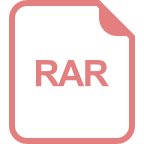











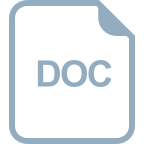
