vue 2 使用three.js 加载gltf模型 并且保证可以旋转 摄像头就在模型前面
时间: 2024-11-15 18:29:57 浏览: 5
在Vue 2中结合Three.js加载Gltf模型并实现实时旋转和相机跟随,你需要做以下几个步骤:
1. **安装依赖**:首先确保你的项目中已经安装了Vue和Three.js。如果还没有,你可以通过npm或yarn来添加:
```bash
npm install vue three @vue/three-core gltf-loader
# 或者
yarn add vue three @vue/three-core gltf-loader
```
2. **创建组件**:创建一个新的Vue组件,例如`GltfModel.vue`,并导入必要的模块:
```javascript
<template>
<div id="app">
<canvas ref="canvas"></canvas>
</div>
</template>
<script>
import * as THREE from 'three';
import { GLTFLoader } from '@loaders.gl/gltf';
export default {
setup() {
const canvas = this.$refs.canvas;
const scene = new THREE.Scene();
const camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000);
const renderer = new THREE.WebGLRenderer({ canvas });
// 初始化Three.js的场景、相机和渲染器
async function loadModel(url) {
const loader = new GLTFLoader();
const model = await loader.load(url);
scene.add(model.scene);
return model.scene;
}
// 加载模型并旋转
const modelNode = loadModel('path_to_your_model.gltf');
modelNode.rotation.y = -Math.PI / 2; // 将模型初始旋转90度使其正面朝向
// 相机跟随模型
function updateCamera() {
camera.position.set(
modelNode.position.x,
modelNode.position.y + 1, // 镜头离地面一点的高度,可根据需求调整
modelNode.position.z
);
camera.lookAt(modelNode.position);
renderer.render(scene, camera);
}
// 渲染和更新
function animate() {
requestAnimationFrame(animate);
updateCamera();
}
animate();
return { modelNode, camera, renderer };
},
};
</script>
```
3. **在父组件中使用**:将这个组件引入到你的主应用组件中,并绑定事件处理。
```html
<template>
<GltfModel />
</template>
```
阅读全文
相关推荐
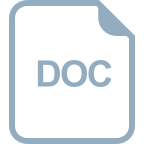
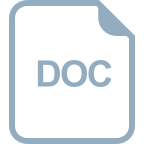
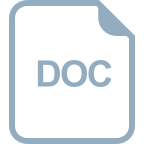
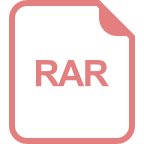












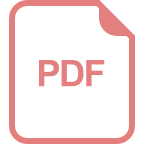

