python with关键词
时间: 2024-03-23 22:34:59 浏览: 50
Python中的`with`关键字用于创建一个上下文管理器,它可以确保在代码块执行完毕后正确地清理资源。常情况下,`with`语句用于处理文件、网络连接、数据库连接等需要手动关闭或释放的资源。
使用`with`关键字的语法如下:
```python
with 上下文表达式 as 变量:
# 执行代码块
```
在`with`语句中,上下文表达式通常是一个返回上下文管理器对象的表达式,该对象必须实现`__enter__()`和`__exit__()`方法。`__enter__()`方法在进入代码块之前被调用,而`__exit__()`方法在代码块执行完毕后被调用。
`with`语句的好处是它会自动处理资源的打开和关闭,无论代码块是否发生异常。即使在代码块中发生了异常,`__exit__()`方法也会被调用来确保资源的正确释放。
下面是一个使用`with`关键字处理文件的示例:
```python
with open('file.txt', 'r') as f:
data = f.read()
# 对文件进行操作
# 在代码块执行完毕后,文件会自动关闭
```
相关问题
python提取关键词
您可以使用Python中的第三方库jieba来提取关键词,具体步骤如下:
1. 安装jieba库,可以使用pip命令进行安装:
```
pip install jieba
```
2. 导入jieba库:
```python
import jieba
```
3. 加载停用词列表,停用词列表中包含了一些无意义的词汇,不应该被当做关键词输出:
```python
stop_words = set()
with open('stop_words.txt', 'r', encoding='utf-8') as f:
for line in f:
stop_words.add(line.strip())
```
4. 对文本进行分词:
```python
text = "这是一段需要进行关键词提取的文本"
words = jieba.cut(text)
```
5. 去除停用词:
```python
words_without_stopwords = [word for word in words if word not in stop_words]
```
6. 统计词频并排序:
```python
word_count = {}
for word in words_without_stopwords:
if word not in word_count:
word_count[word] = 1
else:
word_count[word] += 1
sorted_word_count = sorted(word_count.items(), key=lambda x: x[1], reverse=True)
```
7. 输出关键词:
```python
for item in sorted_word_count:
print(item[0], item[1])
```
需要注意的是,jieba库只能提取中文文本的关键词,如果需要处理英文文本,可以使用nltk库或者spaCy库等。另外,还可以使用TF-IDF算法来提取关键词,使用方法可以参考sklearn库中的TfidfVectorizer类。
python定义关键词
Python 的关键字是指被编程语言保留用于内部处理的单词,不允许被程序员用于变量名或函数名等。Python 3.x 中共有 35 个关键字,它们分别是:
```
False, None, True, and, as, assert, async, await, break, class, continue, def, del, elif, else, except, finally, for, from, global, if, import, in, is, lambda, nonlocal, not, or, pass, raise, return, try, while, with, yield
```
其中,`async` 和 `await` 是 Python 3.5 新增的关键字。
阅读全文
相关推荐
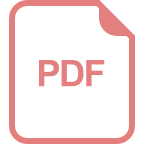
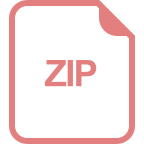
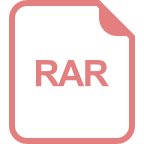













