series,dataframe
时间: 2023-11-18 09:53:10 浏览: 102
Series是pandas中的一个类似一维数组的对象,它可以存储不同类型的数据,并由一组数据和与之相关的索引组成。 您可以使用pd.Series()函数来创建Series对象,其中包括数据、索引等参数。例如,通过以下代码创建一个Series对象:
```python
import pandas as pd
series = pd.Series([['A1', 'A2', 'A3', 'A4'], ['1', '2', '3', '4'], 5, -5, 'test'], index=['A', 'B', 'C', 'D', 'E'])
```
您可以直接打印Series对象来查看其中的数据,也可以通过索引来获取某一行的数据,再进一步获取具体列的值。例如,通过以下代码获取Series中索引为'A'的行数据,并获取该行数据的前两个列的值:
```python
print(series)
print(series['A'])
print(series['A'][0:2])
```
DataFrame是pandas中的一个二维表格数据结构,它由行和列构成,并且每列可以有不同的数据类型。 您可以使用pd.DataFrame()函数来创建DataFrame对象,其中包括数据、列索引和行索引等参数。例如,通过以下代码创建一个DataFrame对象:
```python
import pandas as pd
import numpy as np
data = {'name': ['YYLin', 'YYLin_1', 'YYLin_2', 'YYLin_3', 'YYLin_4'],
'birth_day': [2000, 2001, 2002, 2001, 2002],
'age': [6, 7, 8, 9, 10]}
data_frame = pd.DataFrame(data, index=['one', 'two', 'three', 'four', 'five'])
```
您可以打印DataFrame对象来查看其中的数据和索引信息。例如,通过以下代码打印DataFrame中的列名称和对应的索引值:
```python
print(data_frame.columns)
print(data_frame.index)
```
您可以按列索引或行索引来获取DataFrame中的数据。例如,通过以下代码获取DataFrame中'name'列的数据和'three'行的数据:
```python
print(data_frame['name'])
print(data_frame.loc['three'])
```
您还可以在DataFrame中增加一列数据,并进行相应的操作。例如,通过以下代码增加一列名为'money'的数据,并判断'money'列中的值是否大于3:
```python
import numpy as np
data_frame['money'] = np.arange(5)
print(data_frame)
print(data_frame['money'] > 3)
print(data_frame[data_frame['money'] > 3])
```
这样,您可以得到'money'列中值大于3的数据。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *3* [Pandas入门第一章之Series和DataFrame](https://blog.csdn.net/qq_41776781/article/details/90730708)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT0_1"}}] [.reference_item style="max-width: 50%"]
- *2* [【Pandas数据结构--Series、DataFrame详解】](https://blog.csdn.net/qq_45186086/article/details/125200516)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT0_1"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
阅读全文
相关推荐
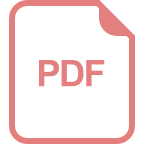
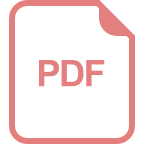
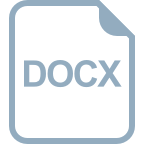
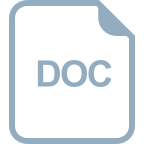
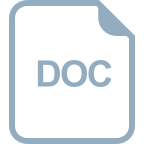













