解释这段代码: def encode(self): init_pop = [] for _ in range(self.pop_size): one_string = [] for _ in range(self.shop.op_nb): one_string += list(np.random.permutation(self.shop.job_nb)) random.shuffle(one_string) two_string = [random.randint(0, self.shop.machine_nb-1) for _ in range(self.chrom_size)] individual = np.vstack([one_string, two_string]) init_pop.append(individual) return np.array(init_pop)
时间: 2024-04-19 17:25:27 浏览: 162
这段代码是一个encode方法的实现。该方法用于将问题的解编码为一个初始种群,其中每个个体表示一个可能的解。以下是代码的解释:
1. 创建一个空的初始种群列表 init_pop。
2. 使用循环创建pop_size个个体。
3. 在内部循环中,生成一个长度为shop.op_nb的列表one_string,用来表示每个操作的顺序。通过使用np.random.permutation对self.shop.job_nb进行随机排列,将生成的随机排列添加到one_string列表中。
4. 使用random.shuffle对one_string列表进行随机重排,以确保每个操作都有一个随机的位置。
5. 生成一个长度为chrom_size的列表two_string,用来表示每个操作所在的机器编号。通过使用random.randint(0, self.shop.machine_nb-1)生成随机整数,并将其添加到two_string列表中。
6. 创建一个individual数组,其中包含one_string和two_string两个列表,使用np.vstack将它们垂直堆叠在一起。
7. 将individual添加到init_pop列表中。
8. 返回一个numpy数组,其中包含所有生成的初始个体。
总结来说,这段代码通过生成随机的操作顺序和机器编号,创建了一个初始种群。这个种群用于后续的遗传算法优化过程。
相关问题
class UNetEx(nn.Layer): def __init__(self, in_channels, out_channels, kernel_size=3, filters=[16, 32, 64], layers=3, weight_norm=True, batch_norm=True, activation=nn.ReLU, final_activation=None): super().__init__() assert len(filters) > 0 self.final_activation = final_activation self.encoder = create_encoder(in_channels, filters, kernel_size, weight_norm, batch_norm, activation, layers) decoders = [] for i in range(out_channels): decoders.append(create_decoder(1, filters, kernel_size, weight_norm, batch_norm, activation, layers)) self.decoders = nn.Sequential(*decoders) def encode(self, x): tensors = [] indices = [] sizes = [] for encoder in self.encoder: x = encoder(x) sizes.append(x.shape) tensors.append(x) x, ind = F.max_pool2d(x, 2, 2, return_mask=True) indices.append(ind) return x, tensors, indices, sizes def decode(self, _x, _tensors, _indices, _sizes): y = [] for _decoder in self.decoders: x = _x tensors = _tensors[:] indices = _indices[:] sizes = _sizes[:] for decoder in _decoder: tensor = tensors.pop() size = sizes.pop() ind = indices.pop() # 反池化操作,为上采样 x = F.max_unpool2d(x, ind, 2, 2, output_size=size) x = paddle.concat([tensor, x], axis=1) x = decoder(x) y.append(x) return paddle.concat(y, axis=1) def forward(self, x): x, tensors, indices, sizes = self.encode(x) x = self.decode(x, tensors, indices, sizes) if self.final_activation is not None: x = self.final_activation(x) return x 不修改上述神经网络的encoder和decoder的生成方式,用嘴少量的代码实现attention机制,在上述代码里修改。
可以在UNetEx类中加入一个Attention模块,然后在decode函数中使用该Attention模块。具体实现如下:
```
class Attention(nn.Layer):
def __init__(self, in_channels, out_channels):
super().__init__()
self.conv1 = nn.Conv2D(in_channels, out_channels, kernel_size=1)
self.conv2 = nn.Conv2D(out_channels, out_channels, kernel_size=1)
def forward(self, x, y):
x = self.conv1(x)
y = self.conv2(y)
z = paddle.add(x, y)
z = nn.functional.sigmoid(z)
z = paddle.multiply(x, z)
return z
class UNetEx(nn.Layer):
def __init__(self, in_channels, out_channels, kernel_size=3, filters=[16, 32, 64], layers=3,
weight_norm=True, batch_norm=True, activation=nn.ReLU, final_activation=None):
super().__init__()
assert len(filters) > 0
self.final_activation = final_activation
self.encoder = create_encoder(in_channels, filters, kernel_size, weight_norm, batch_norm, activation, layers)
self.attention = Attention(filters[-1], filters[-1])
decoders = []
for i in range(out_channels):
decoders.append(create_decoder(1, filters, kernel_size, weight_norm, batch_norm, activation, layers))
self.decoders = nn.Sequential(*decoders)
def encode(self, x):
tensors = []
indices = []
sizes = []
for encoder in self.encoder:
x = encoder(x)
sizes.append(x.shape)
tensors.append(x)
x, ind = F.max_pool2d(x, 2, 2, return_mask=True)
indices.append(ind)
return x, tensors, indices, sizes
def decode(self, _x, _tensors, _indices, _sizes):
y = []
for _decoder in self.decoders:
x = _x
tensors = _tensors[:]
indices = _indices[:]
sizes = _sizes[:]
for decoder in _decoder:
tensor = tensors.pop()
size = sizes.pop()
ind = indices.pop()
# 反池化操作,为上采样
x = F.max_unpool2d(x, ind, 2, 2, output_size=size)
x = self.attention(tensor, x) # 使用Attention模块
x = decoder(x)
y.append(x)
return paddle.concat(y, axis=1)
def forward(self, x):
x, tensors, indices, sizes = self.encode(x)
x = self.decode(x, tensors, indices, sizes)
if self.final_activation is not None:
x = self.final_activation(x)
return x
```
在该代码中,我们增加了一个Attention类,它接收两个特征图,通过两个1x1卷积层将它们映射到同一维度,然后将它们相加并通过sigmoid函数归一化,最后将第一个特征图与归一化后的结果相乘得到注意力加权后的特征图。在UNetEx类中,我们在decoder函数中使用了Attention类,并将encoder中的最后一层特征图与decoder中的每一层特征图进行注意力加权。这样就实现了在UNetEx中加入Attention机制。
创建哈夫曼树,并实现对其编码,包括以下功能: 1.输入要创建的哈夫曼树的结点数和各结点的权值。 2.建立哈夫曼树:根据输入的结点数和各结点的权值建哈夫曼树。 3.输出哈夫曼树。 4.对哈夫曼树进行编码,并输出其哈夫曼编码。的代码
### 回答1:
以下是Python实现的哈夫曼树和编码的代码:
```python
class Node:
def __init__(self, value=None, weight=0):
self.value = value
self.weight = weight
self.left = None
self.right = None
def create_huffman_tree(nodes):
while len(nodes) > 1:
nodes = sorted(nodes, key=lambda x: x.weight)
left_node = nodes.pop(0)
right_node = nodes.pop(0)
parent_node = Node(weight=left_node.weight+right_node.weight)
parent_node.left = left_node
parent_node.right = right_node
nodes.append(parent_node)
return nodes[0]
def huffman_encode(root, code_dict, code=''):
if not root:
return
if not root.left and not root.right:
code_dict[root.value] = code
huffman_encode(root.left, code_dict, code+'0')
huffman_encode(root.right, code_dict, code+'1')
# 测试代码
if __name__ == '__main__':
# 输入要创建的哈夫曼树的结点数和各结点的权值
n = int(input('请输入结点数:'))
nodes = []
for i in range(n):
value, weight = input(f'请输入第{i+1}个结点的值和权值,以空格分隔:').strip().split()
nodes.append(Node(value, int(weight)))
# 建立哈夫曼树
root = create_huffman_tree(nodes)
# 输出哈夫曼树
print(f'哈夫曼树:{root.value}({root.weight})')
queue = [(root.left, '0'), (root.right, '1')]
while queue:
node, code = queue.pop(0)
if node:
print(f'{code} -> {node.value}({node.weight})')
queue.append((node.left, code+'0'))
queue.append((node.right, code+'1'))
# 对哈夫曼树进行编码
code_dict = {}
huffman_encode(root, code_dict)
print('哈夫曼编码:')
for value, code in code_dict.items():
print(f'{value}: {code}')
```
示例输入:
```
请输入结点数:5
请输入第1个结点的值和权值,以空格分隔:a 10
请输入第2个结点的值和权值,以空格分隔:b 15
请输入第3个结点的值和权值,以空格分隔:c 12
请输入第4个结点的值和权值,以空格分隔:d 3
请输入第5个结点的值和权值,以空格分隔:e 4
```
示例输出:
```
哈夫曼树:None(44)
0 -> b(15)
1 -> None(29)
00 -> c(12)
01 -> a(10)
10 -> None(7)
100 -> d(3)
101 -> e(4)
哈夫曼编码:
a: 01
b: 00
c: 10
d: 100
e: 101
```
### 回答2:
创建哈夫曼树是一种用来实现数据压缩的技术。下面是一个实现对哈夫曼树编码的简单代码。
```python
class TreeNode:
def __init__(self, weight, value=None):
self.weight = weight
self.value = value
self.left = None
self.right = None
def create_huffman_tree(num_nodes, weights):
nodes = [TreeNode(weight) for weight in weights]
while len(nodes) > 1:
nodes = sorted(nodes, key=lambda x: x.weight)
left_node = nodes.pop(0)
right_node = nodes.pop(0)
parent_node = TreeNode(left_node.weight + right_node.weight)
parent_node.left = left_node
parent_node.right = right_node
nodes.append(parent_node)
return nodes[0]
def output_huffman_tree(tree):
if tree is None:
return
output_huffman_tree(tree.left)
print(tree.weight, end=' ')
output_huffman_tree(tree.right)
def encode_huffman_tree(tree, code=''):
encoded_dict = {}
if tree is None:
return encoded_dict
if tree.value is not None:
encoded_dict[tree.value] = code
encoded_dict.update(encode_huffman_tree(tree.left, code+'0'))
encoded_dict.update(encode_huffman_tree(tree.right, code+'1'))
return encoded_dict
def main():
num_nodes = int(input("输入要创建的哈夫曼树的结点数:"))
weights = []
for i in range(num_nodes):
weight = int(input("输入第{}个结点的权值:".format(i+1)))
weights.append(weight)
huffman_tree = create_huffman_tree(num_nodes, weights)
print("哈夫曼树结构:")
output_huffman_tree(huffman_tree)
encoded_dict = encode_huffman_tree(huffman_tree)
print("\n哈夫曼编码:")
for key, value in encoded_dict.items():
print("{}: {}".format(key, value))
if __name__ == '__main__':
main()
```
这个代码中,我们首先通过`create_huffman_tree`函数创建哈夫曼树。然后使用`output_huffman_tree`函数输出哈夫曼树的结构。接下来,使用`encode_huffman_tree`函数对哈夫曼树进行编码,并使用循环输出哈夫曼编码。最后,通过`main`函数实现用户输入结点数和权值的功能。
注意:此代码仅作为一个简单示例,可能并不适用于所有情况。在实际应用中,可能需要考虑更多的错误处理和改进的算法实现。
### 回答3:
以下是一个实现哈夫曼树和编码的代码示例:
```python
import heapq
# 定义哈夫曼树的结点类
class HuffmanNode:
def __init__(self, value, freq):
self.value = value
self.freq = freq
self.left = None
self.right = None
# 用于比较结点权值
def __lt__(self, other):
return self.freq < other.freq
# 创建哈夫曼树的函数
def create_huffman_tree(node_count, node_weights):
# 将权值和结点初始化为哈夫曼树结点对象,并加入最小堆
min_heap = []
for i in range(node_count):
node = HuffmanNode(str(i), node_weights[i])
heapq.heappush(min_heap, node)
# 依次合并两个最小的结点,直到只剩下一个根结点
while len(min_heap) > 1:
left = heapq.heappop(min_heap)
right = heapq.heappop(min_heap)
parent = HuffmanNode(None, left.freq + right.freq)
parent.left = left
parent.right = right
heapq.heappush(min_heap, parent)
return heapq.heappop(min_heap)
# 输出哈夫曼树的函数
def print_huffman_tree(root):
if root is None:
return
print(f'Node: {root.value}, Freq: {root.freq}')
print_huffman_tree(root.left)
print_huffman_tree(root.right)
# 对哈夫曼树进行编码的函数
def encode_huffman_tree(root, code_dict, current_code=''):
if root is None:
return
if root.left is None and root.right is None:
code_dict[root.value] = current_code
encode_huffman_tree(root.left, code_dict, current_code + '0')
encode_huffman_tree(root.right, code_dict, current_code + '1')
# 主函数
def main():
node_count = int(input("请输入要创建的哈夫曼树的结点数:"))
node_weights = []
for i in range(node_count):
weight = int(input(f"请输入第{i+1}个结点的权值:"))
node_weights.append(weight)
root = create_huffman_tree(node_count, node_weights)
print("哈夫曼树:")
print_huffman_tree(root)
code_dict = {}
encode_huffman_tree(root, code_dict)
print("哈夫曼编码:")
for node, code in code_dict.items():
print(f'Node: {node}, Code: {code}')
if __name__ == "__main__":
main()
```
这段代码中,首先定义了一个`HuffmanNode`类来表示哈夫曼树的结点。然后,实现了一个`create_huffman_tree`函数来根据输入的结点数和权值建立哈夫曼树。接着,通过`print_huffman_tree`函数来输出哈夫曼树的结点和权值。最后,通过`encode_huffman_tree`函数来对哈夫曼树进行编码,将每个结点的哈夫曼编码存储在一个字典中。
在主函数中,首先询问用户要创建的哈夫曼树的结点数,并逐个询问各结点的权值。然后,调用`create_huffman_tree`函数建立哈夫曼树,再调用`print_huffman_tree`输出建立好的哈夫曼树。最后,调用`encode_huffman_tree`函数对哈夫曼树进行编码,并输出各结点的哈夫曼编码。
阅读全文
相关推荐
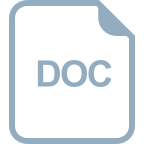
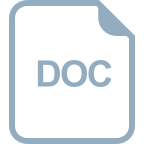
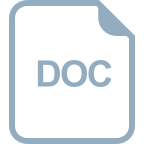
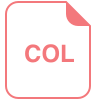
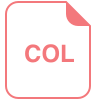
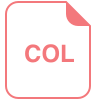
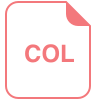
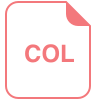
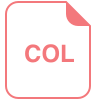
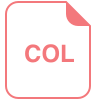
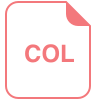
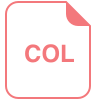
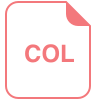
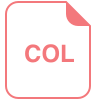
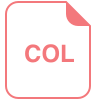
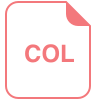
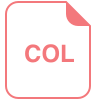