三维装箱matlab
时间: 2023-10-19 20:26:13 浏览: 47
在Matlab中进行三维装箱(3D bin packing)可以用以下步骤实现:
1. 定义问题:确定需要装箱的物体和容器的尺寸,以及装箱的约束条件。每个物体都有自己的尺寸和数量限制,而容器则有自己的尺寸限制。
2. 选择算法:根据具体需求选择合适的算法来解决三维装箱问题。常见的算法包括贪心算法、遗传算法、粒子群算法等。
3. 编写代码:根据选择的算法,使用Matlab编写相应的代码。可以通过定义适当的数据结构来表示物体和容器,以及编写相应的装箱函数和评估函数。
4. 调试和优化:运行代码进行测试,并根据结果进行调试和优化。可以通过调整算法参数、增加约束条件等方式来改进装箱效果。
5. 可视化结果:使用Matlab的绘图功能将装箱结果可视化,以便更直观地观察装箱效果。
需要注意的是,三维装箱是一个复杂的优化问题,具体的实现方式会受到问题规模、约束条件和算法选择等因素的影响。以上是一个基本的框架,具体实现时需要根据实际情况进行调整和修改。
相关问题
三维装箱 matlab
在 Matlab 中进行三维装箱问题的求解可以使用优化工具箱或者自定义算法。以下是一个简单的示例代码,用于演示如何使用整数线性规划方法解决三维装箱问题:
```matlab
% 定义输入数据
box_sizes = [10 20 30; 15 25 35; 8 12 17; 10 10 10]; % 箱子尺寸
items = [5 10 15; 8 12 7; 3 5 9; 6 7 4; 2 3 6]; % 物品尺寸
item_counts = [2 3 1 4 2]; % 物品数量
num_boxes = size(box_sizes, 1);
num_items = size(items, 1);
% 定义整数线性规划模型
intcon = 1:(num_boxes*num_items); % 决策变量为每个物品放入每个箱子的情况
lb = zeros(1, num_boxes*num_items); % 决策变量下界为0,即不放入
ub = ones(1, num_boxes*num_items); % 决策变量上界为1,即放入
Aeq = zeros(num_boxes+num_items, num_boxes*num_items); % 约束矩阵Aeq
beq = zeros(num_boxes+num_items, 1); % 等式约束向量beq
% 确定箱子容量约束
for i = 1:num_boxes
Aeq(i, ((i-1)*num_items+1):(i*num_items)) = ones(1, num_items); % 每个箱子内物品数量之和
beq(i) = 1; % 每个箱子限制为放入一个物品
end
% 确定物品数量约束
for i = 1:num_items
Aeq(num_boxes+i, i:num_items:(num_boxes*num_items)) = ones(1, num_boxes); % 每个物品放入所有箱子的数量之和
beq(num_boxes+i) = item_counts(i); % 物品数量限制
end
% 定义目标函数,最小化装箱体积
f = ones(1, num_boxes*num_items) * box_sizes(:,1).*box_sizes(:,2).*box_sizes(:,3);
% 使用整数线性规划求解器求解问题
x = intlinprog(f, intcon, [], [], Aeq, beq, lb, ub);
% 解析结果
x = reshape(x, num_items, num_boxes); % 将决策变量转换为物品放置矩阵
% 输出结果
for i = 1:num_boxes
disp(['Box ' num2str(i) ':']);
for j = 1:num_items
if x(j,i) > 0
disp(['Item ' num2str(j) ': ' num2str(x(j,i))]);
end
end
disp(' ');
end
```
这是一个简单的示例,假设箱子的限制是每个箱子只能放置一个物品,每个物品的数量也有限制。你可以根据你的具体问题进行适当地修改和调整。
希望能对你有所帮助!
三维装箱matlab代码
三维装箱问题是一个NP难问题,因此没有一种通用的最优解法。常见的解法包括启发式算法、贪心算法、遗传算法等。
以下是一个基于贪心算法的三维装箱Matlab代码示例,仅供参考。
```matlab
clc; clear; close all;
%% 生成测试数据
N = 50; % 物品数量
W = 10; % 容器宽度
H = 10; % 容器高度
L = 10; % 容器长度
w = rand(1, N) * W; % 物品宽度
h = rand(1, N) * H; % 物品高度
l = rand(1, N) * L; % 物品长度
%% 贪心算法
% 初始化容器
box = [W, H, L];
% 初始化物品坐标和状态
pos = zeros(N, 3);
rot = zeros(N, 3);
used = zeros(1, N);
% 装箱
for i = 1:N
% 找到最小空隙
min_gap = inf;
min_gap_pos = zeros(1, 3);
min_gap_box = zeros(1, 3);
min_gap_idx = -1;
min_gap_rot = zeros(1, 3);
for j = 1:N
if ~used(j)
for k = 1:6 % 6种旋转方式
[pos_tmp, box_tmp] = rotate_box(pos(j,:), [w(j),h(j),l(j)], rot(j,:), box);
gap = prod(box_tmp) - sum(used .* prod(box_tmp));
if gap < min_gap
min_gap = gap;
min_gap_pos = pos_tmp;
min_gap_box = box_tmp;
min_gap_idx = j;
min_gap_rot = rot(j,:);
end
rot(j,:) = mod(rot(j,:) + [90,0,0], 360);
if rot(j,1) == 0 % 旋转完毕
break;
end
end
end
end
% 更新状态
pos(min_gap_idx,:) = min_gap_pos;
box = min_gap_box;
used(min_gap_idx) = 1;
end
%% 可视化
figure;
hold on;
for i = 1:N
pos_tmp = pos(i,:);
box_tmp = [w(i),h(i),l(i)];
rot_tmp = rot(i,:);
[pos_tmp, box_tmp] = rotate_box(pos_tmp, box_tmp, rot_tmp, [W,H,L]);
draw_box(pos_tmp, box_tmp);
end
axis equal;
axis([0,W,0,H,0,L]);
grid on;
xlabel('x');
ylabel('y');
zlabel('z');
%% 函数库
function draw_box(pos, box)
x = box(1); y = box(2); z = box(3);
X = [0, x, x, 0, 0, x, x, 0];
Y = [0, 0, y, y, 0, 0, y, y];
Z = [0, 0, 0, 0, z, z, z, z];
for i = 1:8
p = [X(i); Y(i); Z(i)];
p = rotate_point(p, pos);
X(i) = p(1); Y(i) = p(2); Z(i) = p(3);
end
patch(X, Y, Z, 'red');
end
function p = rotate_point(p, pos)
theta = deg2rad(pos(4));
Rx = [1, 0, 0; 0, cos(theta(1)), -sin(theta(1)); 0, sin(theta(1)), cos(theta(1))];
Ry = [cos(theta(2)), 0, sin(theta(2)); 0, 1, 0; -sin(theta(2)), 0, cos(theta(2))];
Rz = [cos(theta(3)), -sin(theta(3)), 0; sin(theta(3)), cos(theta(3)), 0; 0, 0, 1];
R = Rz * Ry * Rx;
p = R * p + pos(1:3)';
end
function [pos_tmp, box_tmp] = rotate_box(pos, box, rot, container)
theta = deg2rad(rot);
Rx = [1, 0, 0; 0, cos(theta(1)), -sin(theta(1)); 0, sin(theta(1)), cos(theta(1))];
Ry = [cos(theta(2)), 0, sin(theta(2)); 0, 1, 0; -sin(theta(2)), 0, cos(theta(2))];
Rz = [cos(theta(3)), -sin(theta(3)), 0; sin(theta(3)), cos(theta(3)), 0; 0, 0, 1];
R = Rz * Ry * Rx;
box_tmp = R * box';
pos_tmp = pos;
pos_tmp(4) = mod(pos_tmp(4) + rot(1), 360);
if pos_tmp(4) == 90 || pos_tmp(4) == 270
pos_tmp(1:2) = pos_tmp(1:2) + (box(1:2) - box_tmp(1:2)) / 2;
else
pos_tmp(1:2) = pos_tmp(1:2) + (box(2:-1:1) - box_tmp(2:-1:1)) / 2;
end
if any(pos_tmp(1:3) + box_tmp' > container) % 碰到边界
pos_tmp(1:3) = pos_tmp(1:3) - (pos_tmp(1:3) + box_tmp' - container) .* (pos_tmp(1:3) + box_tmp' > container);
end
end
```
该代码首先生成了50个随机物品,接着使用贪心算法将它们装入一个10x10x10的容器中,并可视化结果。该算法先遍历每个物品,然后在容器中找到最小空隙,并将物品放入其中,直到所有物品都被放置。在遍历每个物品时,算法会尝试6种旋转方式,并选择能够获得最小空隙的方式。在每次放置物品时,算法会更新容器大小、物品坐标和状态,并继续寻找下一个最小空隙。最终结果如下:

需要注意的是,该算法可能不能得到最优解,并且在物品数量较大时可能耗时较长。因此,在实际应用中,可以考虑使用其他算法,并根据具体情况做出优化。
相关推荐










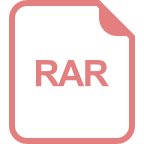