c++输入x和y,输出x年y月有多少天,需要考虑闰年一行一个整数,表示该年该月有多少天。
时间: 2024-09-15 14:12:21 浏览: 73
在 C++ 中,你可以通过创建一个函数来计算给定年份和月份的天数。首先,你需要处理闰年的判断,因为二月有28天(平年)或29天(闰年)。下面是一个简单的示例代码,它会根据用户输入的 x 和 y 计算并输出结果:
```cpp
#include <iostream>
using namespace std;
// 判断是否为闰年
bool is_leap_year(int year) {
if (year % 4 != 0)
return false;
else if (year % 100 != 0)
return true;
else if (year % 400 != 0)
return false;
else
return true;
}
// 给定月份和年份,返回该月天数
int get_days_in_month(int month, int year) {
switch (month) {
case 1:
case 3:
case 5:
case 7:
case 8:
case 10:
case 12:
return 31;
case 4:
case 6:
case 9:
case 11:
return 30;
case 2:
// 考虑闰年和平年
return is_leap_year(year) ? 29 : 28;
default:
// 非法月份
throw invalid_argument("Invalid month");
}
}
int main() {
int x, y;
cout << "请输入年份(x): ";
cin >> x;
cout << "请输入月份(y): ";
cin >> y;
try {
int days = get_days_in_month(y, x);
cout << "年" << x << "月有" << days << "天。\n";
} catch (invalid_argument &e) {
cerr << "错误: " << e.what() << endl;
}
return 0;
}
```
阅读全文
相关推荐
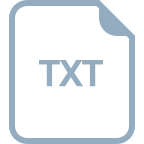
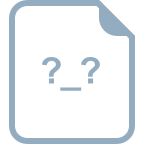
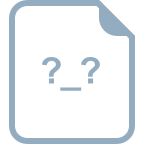
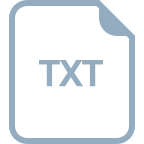
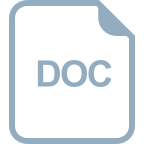
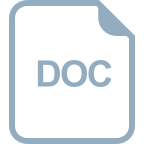
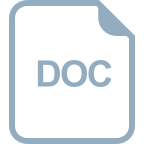
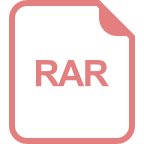
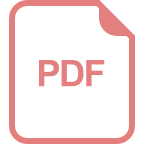





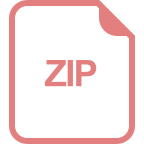