用Python 写出动态路径规划算法
时间: 2024-12-18 22:22:02 浏览: 2
动态路径规划算法,比如常见的Dijkstra算法或A*搜索算法,在Python中可以这样实现。这里以Dijkstra算法为例,它是用于寻找图中两点之间最短路径的经典算法。
首先,你需要安装`heapq`库,它能提供堆数据结构的支持。以下是Dijkstra算法的一个简单版本:
```python
import heapq
def dijkstra(graph, start):
distances = {node: float('infinity') for node in graph}
distances[start] = 0
queue = [(0, start)]
while queue:
current_distance, current_node = heapq.heappop(queue)
if current_distance > distances[current_node]:
continue # 已经找到更短的距离,跳过
for neighbor, weight in graph[current_node].items():
distance = current_distance + weight
if distance < distances[neighbor]:
distances[neighbor] = distance
heapq.heappush(queue, (distance, neighbor))
return distances
# 假设graph是一个字典,表示邻接权重,例如:
# graph = {
# 'A': {'B': 1, 'C': 4},
# 'B': {'A': 1, 'C': 2, 'D': 5},
# 'C': {'A': 4, 'B': 2, 'D': 1},
# 'D': {'B': 5, 'C': 1}
# }
start_node = 'A'
path_distances = dijkstra(graph, start_node)
print(f"从{start_node}到其他点的最短距离:", path_distances)
# 要获取完整的最短路径,可以用优先队列存储节点及其前驱,但这里简化示例
# 实现完整的路径跟踪可能需要辅助数据结构和递归等更多代码。
```
阅读全文
相关推荐
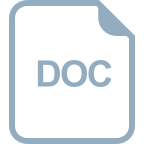
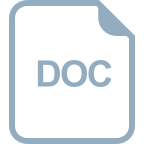
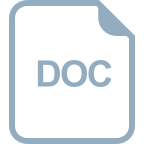



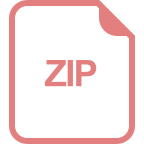
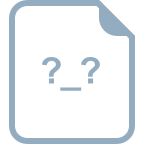
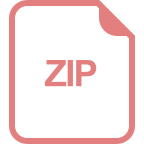
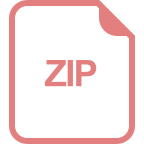








