python实现SHA256和RSA算法
时间: 2024-08-15 09:02:14 浏览: 86
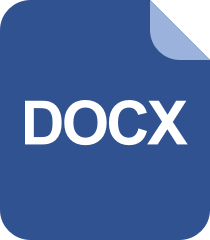
基于python的RSA算法数字签名生成软件设计与实现.docx
Python中可以使用内置的`hashlib`库来实现SHA256哈希函数,它是用于生成消息摘要的一种安全散列函数。你可以像下面这样使用:
```python
import hashlib
def sha256_hash(data):
sha256 = hashlib.sha256()
sha256.update(data.encode('utf-8'))
return sha256.hexdigest()
# 示例
data = "Hello, World!"
hashed_data = sha256_hash(data)
print("SHA256 hash:", hashed_data)
```
对于RSA加密算法,Python并没有直接提供内置支持,但你可以使用第三方库如`cryptography`。首先安装这个库:
```bash
pip install cryptography
```
然后你可以创建密钥对并进行加解密操作:
```python
from cryptography.hazmat.primitives import serialization
from cryptography.hazmat.primitives.asymmetric import rsa, padding
from cryptography.hazmat.backends import default_backend
def generate_rsa_keys():
private_key = rsa.generate_private_key(
public_exponent=65537,
key_size=2048,
backend=default_backend()
)
public_key = private_key.public_key()
return private_key, public_key
def encrypt(message, public_key):
encrypted = public_key.encrypt(
message.encode(),
padding.OAEP(
mgf=padding.MGF1(algorithm=hashes.SHA256()),
algorithm=hashes.SHA256(),
label=None
)
)
return encrypted
def decrypt(encrypted_message, private_key):
decrypted = private_key.decrypt(
encrypted_message,
padding.OAEP(
mgf=padding.MGF1(algorithm=hashes.SHA256()),
algorithm=hashes.SHA256(),
label=None
)
)
return decrypted.decode()
# 示例
private_key, public_key = generate_rsa_keys()
message = "This is a secret message"
encrypted = encrypt(message, public_key)
decrypted = decrypt(encrypted, private_key)
print("Encrypted:", encrypted)
print("Decrypted:", decrypted)
```
阅读全文
相关推荐
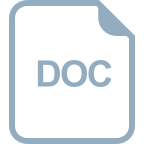
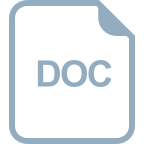
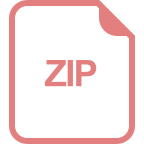
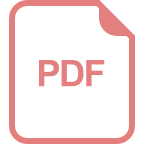













