如何在新增一个维度上拼接多个tensor,用python具体演示
时间: 2024-04-11 18:28:51 浏览: 189
要在新增的维度上拼接多个tensor,可以使用`torch.stack`函数。下面是一个具体的示例代码:
```python
import torch
# 创建两个示例tensor
tensor1 = torch.tensor([[1, 2, 3],
[4, 5, 6]])
tensor2 = torch.tensor([[7, 8, 9],
[10, 11, 12]])
# 在新增的维度上拼接两个tensor
stacked_tensor = torch.stack((tensor1, tensor2), dim=0)
print(stacked_tensor)
# 查看拼接后tensor的形状
print(stacked_tensor.shape)
```
运行以上代码,输出结果如下:
```
tensor([[[ 1, 2, 3],
[ 4, 5, 6]],
[[ 7, 8, 9],
[10, 11, 12]]])
torch.Size([2, 2, 3])
```
在`torch.stack`函数中,第一个参数是一个tuple或list,包含要拼接的tensor。第二个参数`dim`指定了新增的维度。以上示例将两个tensor在新增的维度(维度0)上拼接在一起。拼接后的tensor形状为`(2, 2, 3)`,其中第一个维度表示拼接的个数,第二个和第三个维度保持不变。
相关问题
如何拼接多个tensor,用python具体演示
可以使用`torch.cat`函数来拼接多个tensor。下面是一个具体的示例代码:
```python
import torch
# 创建两个示例tensor
tensor1 = torch.tensor([[1, 2, 3],
[4, 5, 6]])
tensor2 = torch.tensor([[7, 8, 9],
[10, 11, 12]])
# 在行维度上拼接两个tensor
concatenated_tensor = torch.cat((tensor1, tensor2), dim=0)
print(concatenated_tensor)
# 在列维度上拼接两个tensor
concatenated_tensor = torch.cat((tensor1, tensor2), dim=1)
print(concatenated_tensor)
```
运行以上代码,输出结果如下:
```
tensor([[ 1, 2, 3],
[ 4, 5, 6],
[ 7, 8, 9],
[10, 11, 12]])
tensor([[ 1, 2, 3, 7, 8, 9],
[ 4, 5, 6, 10, 11, 12]])
```
`torch.cat`函数的第一个参数是一个tuple或list,包含要拼接的tensor。第二个参数`dim`指定了拼接的维度,0表示在行维度上拼接,1表示在列维度上拼接。以上示例分别在行维度和列维度上将两个tensor拼接在一起。
python tensor向量拼接
在 Python 中,您可以使用 TensorFlow 库中的 `tf.concat()` 函数来进行张量(tensor)的拼接。`tf.concat()` 函数将多个张量沿指定的维度进行拼接。
以下是一个示例代码,演示如何在 TensorFlow 中进行张量的拼接:
```python
import tensorflow as tf
# 创建两个张量
tensor1 = tf.constant([[1, 2, 3], [4, 5, 6]])
tensor2 = tf.constant([[7, 8, 9], [10, 11, 12]])
# 在第一个维度进行拼接
result = tf.concat([tensor1, tensor2], axis=0)
# 打印结果
print(result)
```
输出结果为:
```
tf.Tensor(
[[ 1 2 3]
[ 4 5 6]
[ 7 8 9]
[10 11 12]], shape=(4, 3), dtype=int32)
```
在这个示例中,我们创建了两个2维张量 `tensor1` 和 `tensor2`。然后,我们使用 `tf.concat()` 函数将它们沿着第一个维度进行拼接,即在行方向上进行拼接。最后,我们打印出结果张量 `result`。
您可以通过调整 `axis` 参数来指定其他要拼接的维度。例如,如果您想在列方向上进行拼接,可以将 `axis=1`。
希望这可以帮助到您!如果您有任何其他问题,请随时提问。
阅读全文
相关推荐
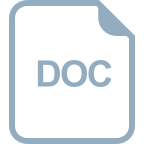
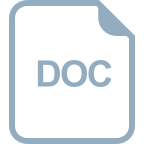
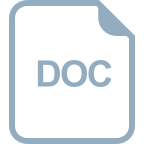
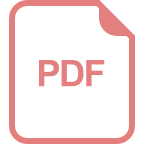
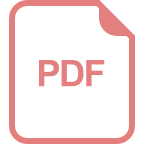











