QTcpSocket *clientSocket = qobject_cast<QTcpSocket*>(sender());是什么意思 如何获得发送数据的客户端
时间: 2024-04-17 12:23:23 浏览: 107
这行代码是一个类型转换,用于将信号发送者(sender)转换为QTcpSocket类型的指针。在网络编程中,当服务器接收到客户端发送的数据时,通过信号槽机制,可以通过这行代码来获取发送数据的客户端的QTcpSocket对象。
具体来说,当服务器接收到客户端发送的数据时,会触发一个信号,信号的发送者就是发送数据的客户端的QTcpSocket对象。通过使用qobject_cast<QTcpSocket*>(sender()),可以将发送者转换为QTcpSocket类型的指针,然后可以使用该指针来操作和获取发送数据的客户端的信息。
请注意,这行代码需要在接收数据的槽函数中使用,并且确保发送者是一个QTcpSocket对象。
相关问题
qt tcp发送数据到指定客户端
在Qt中,可以使用QTcpServer和QTcpSocket进行TCP通信,实现发送数据到指定客户端的功能。
1. 首先,创建一个QTcpServer对象,并在指定的端口上监听连接请求:
```cpp
QTcpServer server;
if (!server.listen(QHostAddress::Any, port)) {
qDebug() << "Server could not start!";
return;
}
```
2. 当有新的连接请求到达时,通过accept()函数接受连接,并创建一个QTcpSocket对象来处理与客户端的通信:
```cpp
void MyClass::newConnection()
{
while (server.hasPendingConnections()) {
QTcpSocket *socket = server.nextPendingConnection();
connect(socket, SIGNAL(readyRead()), this, SLOT(readData()));
// 保存客户端的Socket对象,可以通过socket指针标识不同的客户端
// ...
}
}
```
3. 当需要发送数据到指定客户端时,找到对应的QTcpSocket对象,使用write()函数发送数据:
```cpp
QTcpSocket *clientSocket; // 指向指定客户端的QTcpSocket对象
QByteArray data = "Hello, client!";
clientSocket->write(data);
```
4. 最后,需要在QTcpSocket对象的connected()和disconnected()等信号的槽函数中处理连接状态的变化,并进行必要的清理和断开操作:
```cpp
void MyClass::clientConnected()
{
QTcpSocket *clientSocket = qobject_cast<QTcpSocket*>(sender());
if (clientSocket) {
// 连接建立成功的处理操作
// ...
}
}
void MyClass::clientDisconnected()
{
QTcpSocket *clientSocket = qobject_cast<QTcpSocket*>(sender());
if (clientSocket) {
// 断开连接的处理操作
// ...
}
}
```
通过以上步骤,我们可以在Qt中实现发送数据到指定客户端的TCP通信功能。当收到指定客户端的连接时,将其对应的QTcpSocket对象保存下来,然后可以通过该对象发送数据到该客户端。同时,需要注意在连接状态改变时进行相应的处理操作。
用qt写一段代码实现两个上位机通过代理连接两个下位机,两个下位机收到命令后会发送的数据,发送的数据会在两台上位机上同时显示出来
好的,以下是一个简单的实现思路和代码示例:
1. 在Server端建立一个TCP服务器,等待Client的连接请求,代码如下:
```cpp
QTcpServer *server = new QTcpServer(this);
connect(server, &QTcpServer::newConnection, this, &Server::handleNewConnection);
if (!server->listen(QHostAddress::Any, 1234)) {
qCritical() << "Failed to start server!";
return;
}
qInfo() << "Server started listening on port 1234!";
```
2. 在Server的handleNewConnection槽函数中,接受Client的连接请求,并为其创建一个QTcpSocket,代码如下:
```cpp
void Server::handleNewConnection() {
QTcpSocket *clientSocket = server->nextPendingConnection();
connect(clientSocket, &QTcpSocket::readyRead, this, &Server::handleReadyRead);
connect(clientSocket, &QTcpSocket::disconnected, clientSocket, &QObject::deleteLater);
qInfo() << "Client connected!";
}
```
3. 在Client端,建立一个QTcpSocket,通过代理连接Server,代码如下:
```cpp
QNetworkProxy proxy;
proxy.setType(QNetworkProxy::Socks5Proxy);
proxy.setHostName("proxy.example.com");
proxy.setPort(1080);
QNetworkProxy::setApplicationProxy(proxy);
QTcpSocket *socket = new QTcpSocket(this);
connect(socket, &QTcpSocket::readyRead, this, &Client::handleReadyRead);
connect(socket, &QTcpSocket::disconnected, this, &QObject::deleteLater);
socket->connectToHost("server.example.com", 1234);
if (!socket->waitForConnected()) {
qCritical() << "Failed to connect to server!";
return;
}
qInfo() << "Connected to server!";
```
4. 在Server端的handleReadyRead槽函数中,接收Client发送的命令,将命令发送给两个下位机,并等待下位机的回复,代码如下:
```cpp
void Server::handleReadyRead() {
QTcpSocket *clientSocket = qobject_cast<QTcpSocket *>(sender());
QDataStream in(clientSocket);
while (in.device()->bytesAvailable() > 0) {
QByteArray command;
in >> command;
qInfo() << "Received command: " << command;
// Send command to both devices
device1Socket->write(command);
device2Socket->write(command);
// Wait for reply from devices
QByteArray reply1 = device1Socket->readAll();
QByteArray reply2 = device2Socket->readAll();
// Send reply to client
QByteArray reply;
reply.append(reply1);
reply.append(reply2);
clientSocket->write(reply);
}
}
```
5. 在Client端的handleReadyRead槽函数中,接收Server发送的数据,将数据显示在程序中,并将数据发送给另一个上位机,代码如下:
```cpp
void Client::handleReadyRead() {
QDataStream in(socket);
while (in.device()->bytesAvailable() > 0) {
QByteArray data;
in >> data;
qInfo() << "Received data: " << data;
// Display data in UI
ui->textEdit->append(QString::fromUtf8(data));
// Forward data to other client
otherClientSocket->write(data);
}
}
```
注意:上面的示例代码只是一个简单的演示,实际实现中需要根据具体需求进行修改。另外,建议将连接、发送和接收等操作都放在单独的线程中进行,以免阻塞主线程。
阅读全文
相关推荐
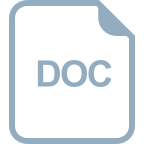
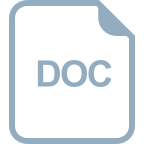
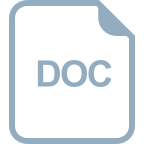
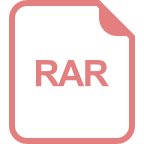
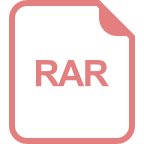
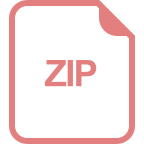










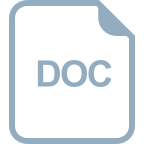