word文档(包含图片)转换成html在页面展示 vue3
时间: 2023-10-13 15:03:06 浏览: 1120
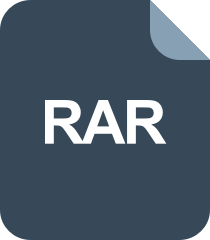
exportToWord导出页面到word文档,亲测可用
要将包含图片的Word文档转换为在页面上展示的HTML,可以使用Vue3框架来实现。
首先,需要将Word文档转换为HTML格式。可以使用开源库如`mammoth.js`来实现。该库可以将Word文档中的内容转换为HTML,包括格式和图片。
然后,在Vue3项目中创建一个组件,用于展示转换后的HTML内容。可以在组件的`data`选项中定义一个变量,用于存储转换后的HTML内容。可以使用`v-html`指令将HTML内容直接插入到组件的模板中。
在组件的生命周期钩子`mounted`中,可以调用转换Word文档的方法,并将转换后的HTML内容存储在组件的变量中。这样,在组件模板中可以通过`v-html`指令将HTML内容渲染出来。
另外,需要确保图片能够正确显示。可以将转换后的HTML中的图片路径修改为项目中存储图片的路径。可以使用Vue3提供的静态资源加载策略,通过`require`或者直接在模板中使用相对路径来引用图片。
最后,将该组件在Vue3的根组件中引入,并在页面中使用该组件即可展示转换后的HTML内容。
总结一下,将Word文档转换为HTML需要使用开源库实现,然后通过Vue3的组件和指令来展示转换后的HTML内容。同时,需要处理好图片的显示问题。这样就可以在页面上展示转换后的Word文档了。
阅读全文
相关推荐
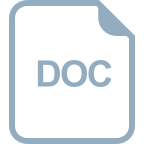
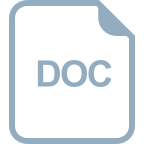















