帮我写一个树莓派串口通讯代码
时间: 2024-06-12 20:08:27 浏览: 95
当在树莓派上进行串口通讯时,我们可以使用 C 语言编写代码来实现。以下是一个简单的示例代码,用于在树莓派上通过串口通信发送和接收数据。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <fcntl.h>
#include <unistd.h>
#include <termios.h>
int main() {
int serial_port = open("/dev/ttyS0", O_RDWR); // 打开串口设备文件 "/dev/ttyS0"
if (serial_port < 0) {
perror("无法打开串口");
return -1;
}
struct termios tty;
memset(&tty, 0, sizeof(tty)); // 清空结构体
if (tcgetattr(serial_port, &tty) != 0) {
perror("获取串口属性失败");
return -1;
}
// 设置波特率为9600
cfsetospeed(&tty, B9600);
cfsetispeed(&tty, B9600);
tty.c_cflag &= ~PARENB; // 禁用奇偶校验
tty.c_cflag &= ~CSTOPB; // 设置停止位为1
tty.c_cflag &= ~CSIZE; // 重置数据位
tty.c_cflag |= CS8; // 设置数据位为8
tty.c_cflag &= ~CRTSCTS; // 禁用硬件流控制
tty.c_cflag |= CREAD | CLOCAL; // 启用读取和忽略控制位
tty.c_iflag &= ~(IXON | IXOFF | IXANY); // 禁用软件流控制
tty.c_lflag = 0;
tty.c_oflag = 0;
tty.c_cc[VMIN] = 0; // 读取的最小字符数
tty.c_cc[VTIME] = 10; // 读取超时时间(单位:百毫秒)
if (tcsetattr(serial_port, TCSANOW, &tty) != 0) {
perror("设置串口属性失败");
return -1;
}
char tx_buffer[] = "Hello, Raspberry Pi!";
char rx_buffer[256];
printf("发送数据:%s\n", tx_buffer);
write(serial_port, tx_buffer, strlen(tx_buffer)); // 发送数据
usleep(100000); // 等待接收数据
int num_bytes = read(serial_port, &rx_buffer, sizeof(rx_buffer)); // 接收数据
if (num_bytes > 0) {
rx_buffer[num_bytes] = '\0';
printf("接收到的数据:%s\n", rx_buffer);
} else {
printf("未接收到数据\n");
}
close(serial_port); // 关闭串口
return 0;
}
```
这段代码演示了如何打开串口设备文件,设置串口属性,发送数据,接收数据,并关闭串口。请注意,此代码假设你的树莓派上的串口设备文件为 "/dev/ttyS0",波特率为 9600。
请注意,在树莓派上运行此代码时,你需要有足够的权限来访问串口设备文件。你可以使用 `sudo` 命令运行该程序,或者将自己添加到 dialout 用户组以获取对串口的访问权限。
阅读全文
相关推荐
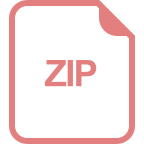

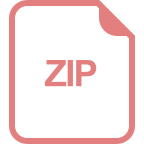
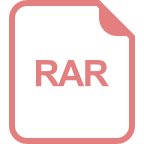
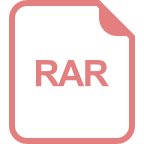
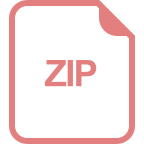
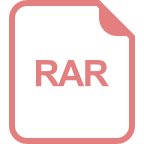
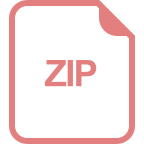
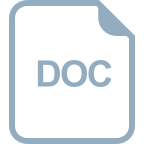
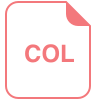



