c语言分支限界法实现背包问题
时间: 2024-02-20 22:55:29 浏览: 84
C语言中的分支限界法可以用来解决背包问题。背包问题是一个经典的组合优化问题,目标是在给定的一组物品中选择一些物品放入背包中,使得背包的总重量不超过背包的容量,同时价值最大化。
分支限界法是一种穷举搜索的方法,通过剪枝操作来减少搜索空间,提高求解效率。具体实现背包问题的分支限界法可以按照以下步骤进行:
1. 定义一个结构体来表示物品,包括物品的重量和价值。
2. 定义一个优先队列(也可以使用堆)来存储待扩展的节点,节点包括当前已选择的物品、当前背包的重量、当前背包的价值以及当前已选择的物品的下标。
3. 初始化优先队列,将初始节点加入队列。
4. 进入循环,直到队列为空或者找到最优解为止:
- 从队列中取出一个节点。
- 如果当前节点已经选择了所有物品或者当前背包已满,则更新最优解。
- 否则,根据当前节点的选择情况,生成两个子节点:选择下一个物品放入背包和不选择下一个物品放入背包。
- 对于每个子节点,计算其上界(即剩余物品的价值和当前背包的价值之和),如果上界大于当前最优解,则将子节点加入队列。
5. 返回最优解。
这样,通过分支限界法可以找到背包问题的最优解。
相关问题
用c语言分支限界法实现01背包问题
以下是用C语言实现01背包问题的分支限界法的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_N 100 // 背包最大物品数量
#define MAX_W 1000 // 背包最大容纳重量
// 物品结构体
typedef struct item {
int id; // 物品编号
int weight; // 物品重量
int profit; // 物品价值
float bound; // 松弛上界
} item;
// 节点结构体
typedef struct node {
int level; // 节点所在层级
int profit; // 当前总价值
int weight; // 当前总重量
float bound; // 松弛上界
} node;
// 全局变量
int n; // 物品数量
int w; // 背包容量
item items[MAX_N]; // 物品数组
float max_profit; // 最大收益
int opt_x[MAX_N]; // 最优解
// 比较函数,用于qsort排序
int cmp(const void *a, const void *b) {
item *p1 = (item *)a;
item *p2 = (item *)b;
float r1 = (float)p1->profit / p1->weight;
float r2 = (float)p2->profit / p2->weight;
return r2 > r1 ? 1 : -1;
}
// 计算松弛上界
float get_bound(node n) {
int level = n.level;
float bound = n.profit;
int w = n.weight;
while (level < n && w < W) {
if (w + items[level].weight <= W) {
w += items[level].weight;
bound += items[level].profit;
} else {
float remain = W - w;
bound += remain * (float)items[level].profit / items[level].weight;
break;
}
level++;
}
return bound;
}
// 分支限界法
void branch_bound() {
node q[MAX_N]; // 队列
int head = 0, tail = 0;
node u, v;
u.level = -1;
u.profit = u.weight = 0;
u.bound = get_bound(u);
q[tail++] = u;
while (head < tail) {
u = q[head++];
if (u.bound > max_profit) {
v.level = u.level + 1;
v.weight = u.weight + items[v.level].weight;
v.profit = u.profit + items[v.level].profit;
v.bound = get_bound(v);
if (v.weight <= W && v.profit > max_profit) {
max_profit = v.profit;
for (int i = 0; i <= v.level; i++) {
opt_x[items[i].id] = items[i].weight;
}
}
if (v.bound > max_profit) {
q[tail++] = v;
}
v.weight = u.weight;
v.profit = u.profit;
v.bound = get_bound(v);
if (v.bound > max_profit) {
q[tail++] = v;
}
}
}
}
// 主函数
int main() {
scanf("%d %d", &n, &W);
for (int i = 0; i < n; i++) {
scanf("%d %d", &items[i].weight, &items[i].profit);
items[i].id = i;
}
qsort(items, n, sizeof(item), cmp);
branch_bound();
printf("最大收益为:%f\n", max_profit);
printf("最优解为:\n");
for (int i = 0; i < n; i++) {
printf("%d ", opt_x[i]);
}
printf("\n");
return 0;
}
```
这个程序实现了01背包问题的分支限界法,使用了一个node结构体表示当前节点的状态,其中包含了当前的层级、总价值、总重量和松弛上界。程序使用了一个队列来保存当前的节点,每次从队列中取出一个节点进行拓展,计算出其子节点的状态,加入队列中。在拓展节点时,如果当前节点的松弛上界大于当前最大收益,则继续拓展,否则直接舍弃。
这个程序的时间复杂度为O(2^n),因为最坏情况下需要遍历所有可能的节点。
C语言分支限界法背包问题代码
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct {
int weight; // 物品重量
int value; // 物品价值
float ratio; // 物品价值与重量的比值
} Item;
int compare(const void *a, const void *b) {
Item *itemA = (Item *)a;
Item *itemB = (Item *)b;
return (itemB->ratio - itemA->ratio);
}
float knapsack(int capacity, Item items[], int n) {
qsort(items, n, sizeof(Item), compare); // 按照物品价值与重量的比值进行降序排序
int currentWeight = 0; // 当前背包重量
float currentValue = 0; // 当前背包价值
for (int i = 0; i < n; i++) {
if (currentWeight + items[i].weight <= capacity) {
currentWeight += items[i].weight;
currentValue += items[i].value;
} else {
int remainingCapacity = capacity - currentWeight;
currentValue += items[i].ratio * remainingCapacity;
break;
}
}
return currentValue;
}
int main() {
int capacity = 50; // 背包容量
int n = 5; // 物品数量
Item items[n];
items[0].weight = 10;
items[0].value = 60;
items[0].ratio = 6;
items[1].weight = 20;
items[1].value = 100;
items[1].ratio = 5;
items[2].weight = 30;
items[2].value = 120;
items[2].ratio = 4;
items[3].weight = 40;
items[3].value = 140;
items[3].ratio = 3.5;
items[4].weight = 50;
items[4].value = 160;
items[4].ratio = 3.2;
float maxValue = knapsack(capacity, items, n);
printf("Max value: %.2f\n", maxValue);
return 0;
}
```
阅读全文
相关推荐
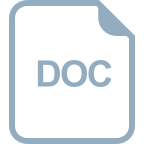
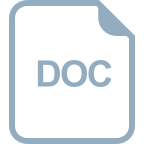
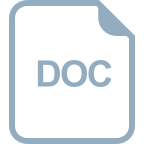













