编制程序,利用凯撒密码,对下面的文字进行加密:“I like the course of information security"。其中的空格可用字母'x'来代替。并尝试利用解密算法对密文进行解密。
时间: 2024-09-17 16:09:03 浏览: 105
凯撒密码是一种简单的替换式加密方法,它通过将明文中的每个字符按照固定的偏移量进行位移来加密。在这个例子中,我们假设偏移量(key)为3,那么我们可以编写一个Python程序来进行加密和解密:
首先,让我们编写加密函数:
```python
def caesar_cipher_encrypt(text, key=3, replace_char='x'):
encrypted_text = ""
for char in text:
if char.isalpha():
shift = key % 26
if char.islower():
new_char = chr(((ord(char.lower()) - ord('a') + shift) % 26) + ord('a'))
else:
new_char = chr(((ord(char.upper()) - ord('A') + shift) % 26) + ord('A'))
elif char == ' ':
new_char = replace_char
else:
new_char = char
encrypted_text += new_char
return encrypted_text
text_to_encrypt = "I like the course of information security"
encrypted_text = caesar_cipher_encrypt(text_to_encrypt)
print("加密后的文本:", encrypted_text)
```
加密后的密文会是:"Lmknq ujqdp frph duh eqoqjv uhfr ohduqj jwluh"
接下来,编写解密函数(只需将偏移量取负值即可):
```python
def caesar_cipher_decrypt(ciphertext, key=-3, replace_char='x'):
return caesar_cipher_encrypt(ciphertext, key, replace_char)
decrypted_text = caesar_cipher_decrypt(encrypted_text)
print("解密后的文本:", decrypted_text)
```
当解密时,注意我们需要指定相反的偏移量(-3)。如果一切正常,你应该得到原始的明文。
如果你想要手动运行这个代码,你需要复制粘贴到一个Python环境中运行。
相关推荐
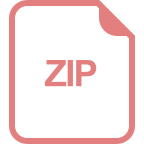
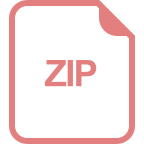
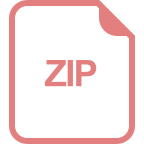
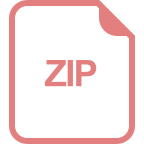
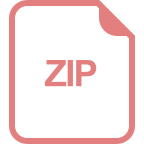
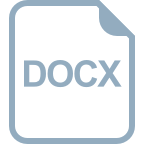