Python中求三角函数的导数
时间: 2023-11-16 22:52:23 浏览: 193
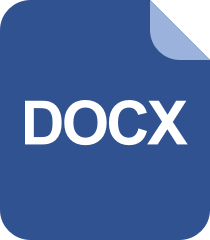
python中三角函数的表示
在Python中,可以使用math模块来计算三角函数的导数。具体来说,可以使用math.sin()、math.cos()和math.tan()函数来计算正弦、余弦和正切函数的值,并使用sympy库中的diff()函数来计算导数。
下面是一个示例代码,演示如何计算sin(x)和cos(x)的导数:
```python
import math
import sympy as sp
x = sp.symbols('x')
f = sp.sin(x)
g = sp.cos(x)
df = sp.diff(f, x)
dg = sp.diff(g, x)
print("sin(x)的导数:", df)
print("cos(x)的导数:", dg)
```
输出:
```
sin(x)的导数: cos(x)
cos(x)的导数: -sin(x)
```
阅读全文
相关推荐
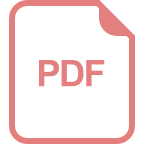

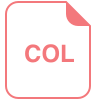
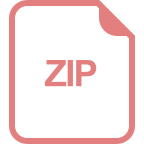
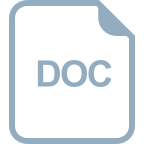
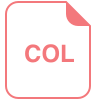
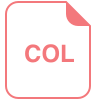
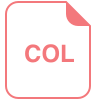
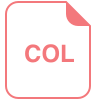
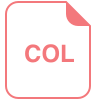
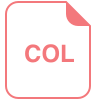
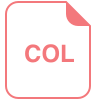
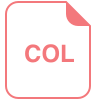
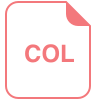
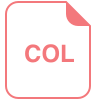
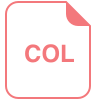
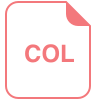