标签格式转换json转txt
时间: 2023-08-17 09:11:40 浏览: 117
你可以使用Python的内置库`json`来实现将JSON格式转换为TXT格式。下面是一个示例代码:
```python
import json
def json_to_txt(json_data, txt_file):
with open(txt_file, 'w') as file:
json.dump(json_data, file, indent=4)
# 示例JSON数据
json_data = {
"name": "John",
"age": 30,
"city": "New York"
}
# 转换为TXT格式
json_to_txt(json_data, 'data.txt')
```
以上代码将会把JSON数据转换为TXT格式,并保存到名为`data.txt`的文件中。你可以根据自己的需求修改JSON数据和文件名。转换后的TXT文件将会包含美观的缩进格式。
相关问题
json转txt yolo
你可以使用Python中的`json`和`txt`库来实现将JSON格式转换为Yolo格式的TXT文件。以下是一个示例代码:
```python
import json
def convert_json_to_yolo(json_file, txt_file):
with open(json_file, 'r') as f:
data = json.load(f)
with open(txt_file, 'w') as f:
for obj in data['objects']:
label = obj['label']
x, y, w, h = obj['bbox']
# 转换坐标为Yolo格式
x_center = x + w / 2
y_center = y + h / 2
width = w
height = h
# 将转换后的信息写入TXT文件
line = f"{label} {x_center} {y_center} {width} {height}\n"
f.write(line)
# 示例调用
json_file = 'input.json'
txt_file = 'output.txt'
convert_json_to_yolo(json_file, txt_file)
```
请将上述代码中的`json_file`替换为你的JSON文件路径,将`txt_file`替换为你想要保存Yolo格式结果的TXT文件路径。运行代码后,它将从JSON文件中提取对象的标签和边界框信息,并将它们转换为Yolo格式的TXT文件。
json转txt yolov7
以下是将json文件转换为yolov7所需的txt标签格式的Python脚本示例:
```python
import json
def convert_json_to_txt(json_file, txt_file):
with open(json_file, 'r') as f:
data = json.load(f)
with open(txt_file, 'w') as f:
for obj in data['objects']:
class_id = obj['class_id']
points = obj['points']
line = f"{class_id} "
for point in points:
line += f"{point['x']} {point['y']} "
f.write(line.strip() + "\n")
# 指定json文件路径和输出的txt文件路径
json_file = 'path/to/input.json'
txt_file = 'path/to/output.txt'
# 调用函数进行转换
convert_json_to_txt(json_file, txt_file)
```
请注意,你需要将`path/to/input.json`替换为实际的json文件路径,将`path/to/output.txt`替换为你想要保存txt文件的路径。
阅读全文
相关推荐
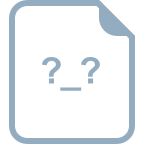
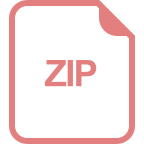
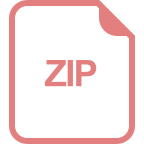
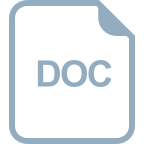
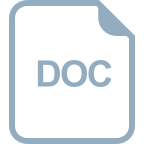
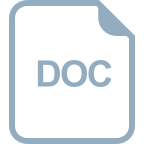







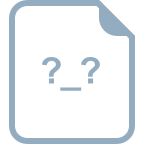