给定一个集合,使用集合的内置方法 remove或discard来删除集合内的非整型数据,然后将集合转换成列表并对其进行排序。
时间: 2024-09-19 21:05:09 浏览: 38
首先,你需要导入需要的Python库,如`collections`(如果你正在处理的是Python字典或集合)或`list`(用于操作列表)。假设你有一个混合类型的序列,例如:
```python
mixed_sequence = [1, 'a', 2, 'b', 3, 'c']
```
你可以按照以下步骤操作:
1. 使用`filter()`函数过滤出只有整数的部分,因为`filter()`接受一个函数作为参数,该函数会作用于每个元素并返回True或False,表示是否保留该元素。这里可以使用`isinstance()`检查元素是否为int。
```python
integer_sequence = list(filter(lambda x: isinstance(x, int), mixed_sequence))
```
2. 接下来,你可以使用`sorted()`函数对剩下的整数进行排序,`sorted()`默认会升序排列。
```python
sorted_list = sorted(integer_sequence)
```
如果你想使用集合(set)而不是列表,并且集合不允许有重复值,可以先转换到集合再转回列表:
```python
# 如果你想保持唯一值
integer_set = {x for x in mixed_sequence if isinstance(x, int)}
sorted_list = list(sorted(integer_set))
```
如果你想要删除集合(set)中的某个元素而不关心其类型,可以使用`discard()`方法:
```python
# 从集合中移除特定的整数值,比如移除3
integer_set.discard(3)
sorted_list = list(sorted(integer_set))
```
阅读全文
相关推荐
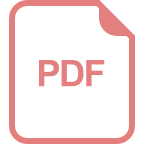
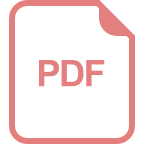
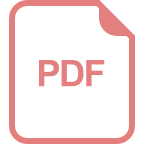
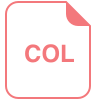




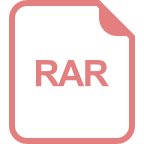
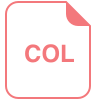








