c#:声明抽象类形状(Shape)作为基类,派生类为2个:矩形(Rectangle)和圆形(Circle);形状中含有两个抽象方法:计算面积和显示信息。利用多态性实现,计算两种不同形状的面积和显示对应形状的信息。
时间: 2024-12-11 15:28:18 浏览: 22
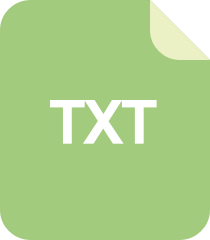
c++程序编程
在C#中,你可以创建一个抽象基类`Shape`,然后让`Rectangle`和`Circle`类继承自它,并实现抽象方法。抽象类允许你定义共同的行为,而抽象方法则需要在其派生类中提供具体的实现。以下是代码示例:
```csharp
using System;
// 抽象基类 Shape
public abstract class Shape
{
// 抽象方法 - 计算面积
public abstract double CalculateArea();
// 抽象方法 - 显示信息
public abstract void DisplayInfo();
}
// 派生类 Rectangle
public class Rectangle : Shape
{
private double width;
private double height;
public Rectangle(double width, double height)
{
this.width = width;
this.height = height;
}
public override double CalculateArea()
{
return width * height;
}
public override void DisplayInfo()
{
Console.WriteLine($"Rectangle: Width={width}, Height={height}");
}
}
// 派生类 Circle
public class Circle : Shape
{
private double radius;
public Circle(double radius)
{
this.radius = radius;
}
public override double CalculateArea()
{
return Math.PI * radius * radius;
}
public override void DisplayInfo()
{
Console.WriteLine($"Circle: Radius={radius}");
}
}
// 使用多态性示例
public static void Main()
{
Shape rectangle = new Rectangle(5, 4);
Shape circle = new Circle(3);
rectangle.DisplayInfo(); // 输出:Rectangle: Width=5, Height=4
rectangle.CalculateArea(); // 输出:20 (矩形面积)
circle.DisplayInfo(); // 输出:Circle: Radius=3
circle.CalculateArea(); // 输出:28.274333882308138 (圆的面积)
}
```
在这个例子中,尽管`CalculateArea`和`DisplayInfo`方法在`Shape`基类中被标记为抽象的,但每个派生类都实现了它们,展示了多态性。
阅读全文
相关推荐
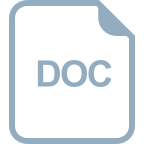
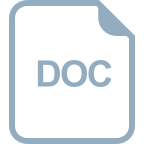















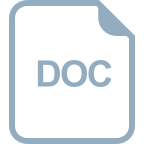