揭秘Java最大公约数算法的奥秘:从数学到代码实践
发布时间: 2024-08-27 22:27:03 阅读量: 32 订阅数: 29 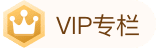
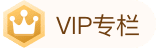
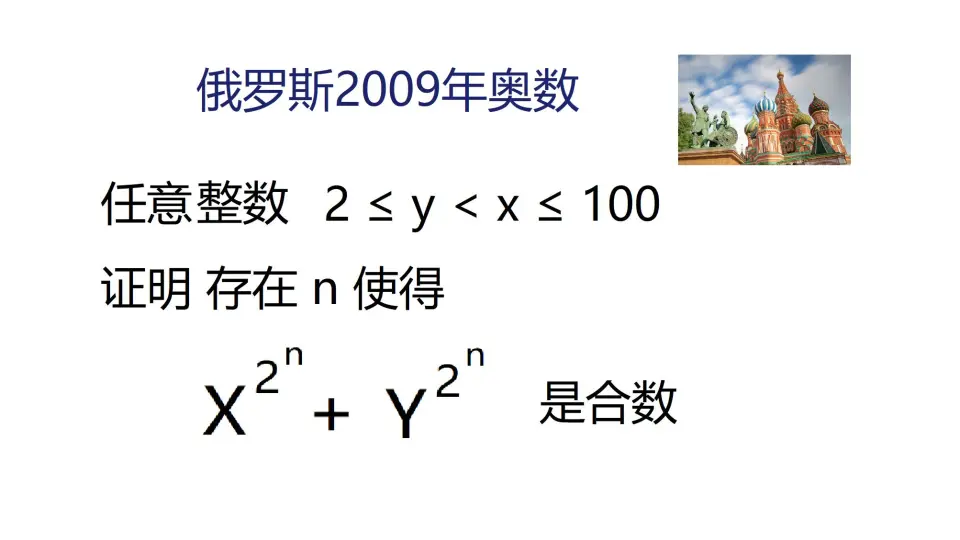
# 1. 最大公约数的数学基础**
最大公约数(Greatest Common Divisor,GCD)是两个或多个整数中最大的公因子。在数学中,最大公约数有重要的应用,例如分数化简、约数和倍数的计算等。
最大公约数的计算有多种方法,其中最常见的是辗转相除法。辗转相除法的原理是:对于两个整数a和b,如果a除以b的余数为r,那么a和b的最大公约数等于b和r的最大公约数。
# 2. Java最大公约数算法的实现
### 2.1 辗转相除法
#### 2.1.1 算法原理
辗转相除法是求解最大公约数的一种经典算法,其原理基于以下定理:
**定理:** 对于两个正整数 a 和 b,如果 b 不为 0,则 a 和 b 的最大公约数等于 a 除以 b 的余数和 b 的最大公约数。
#### 2.1.2 代码实现
```java
public static int gcd(int a, int b) {
if (b == 0) {
return a;
} else {
return gcd(b, a % b);
}
}
```
**代码逻辑分析:**
* 如果 b 为 0,则 a 和 b 的最大公约数为 a,直接返回 a。
* 否则,计算 a 除以 b 的余数,并将其赋值给 a。
* 将 b 赋值给 b,继续递归调用 gcd 方法,直到 b 为 0。
### 2.2 递归算法
#### 2.2.1 算法原理
递归算法也是求解最大公约数的一种方法,其原理与辗转相除法类似,但采用递归的方式实现。
#### 2.2.2 代码实现
```java
public static int gcd(int a, int b) {
if (a == 0) {
return b;
} else if (b == 0) {
return a;
} else {
return gcd(b, a % b);
}
}
```
**代码逻辑分析:**
* 如果 a 为 0,则 a 和 b 的最大公约数为 b,直接返回 b。
* 如果 b 为 0,则 a 和 b 的最大公约数为 a,直接返回 a。
* 否则,计算 a 除以 b 的余数,并将其赋值给 a。
* 将 b 赋值给 b,继续递归调用 gcd 方法,直到 a 或 b 为 0。
# 3. 最大公约数算法的应用**
### 3.1 分数化简
#### 3.1.1 分数化简的原理
分数化简是指将一个分数化简为最简形式,即分子和分母都没有公约数的分数。最大公约数算法可以用来化简分数,具体步骤如下:
1. 求分子和分母的最大公约数。
2. 用分子除以最大公约数,得到化简后的分子。
3. 用分母除以最大公约数,得到化简后的分母。
例如,化简分数 12/18:
1. 求最大公约数:gcd(12, 18) = 6。
2. 化简后的分子:12 / 6 = 2。
3. 化简后的分母:18 / 6 = 3。
因此,化简后的分数为 2/3。
#### 3.1.2 代码实现
```java
import java.util.Scanner;
public class FractionSimplification {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
// 获取分子和分母
System.out.println("请输入分子:");
int numerator = input.nextInt();
System.out.println("请输入分母:");
int denominator = input.nextInt();
// 求最大公约数
int gcd = gcd(numerator, denominator);
// 化简分数
numerator /= gcd;
denominator /= gcd;
// 输出化简后的分数
System.out.println("化简后的分数:" + numerator + "/" + denominator);
}
// 求最大公约数
public static int gcd(int a, int b) {
if (b == 0) {
return a;
} else {
return gcd(b, a % b);
}
}
}
```
**代码逻辑分析:**
1. `gcd` 方法使用辗转相除法求最大公约数。
2. `main` 方法获取分子和分母,调用 `gcd` 方法求最大公约数,然后化简分数。
3. 化简后的分数通过 `System.out.println` 输出。
### 3.2 约数和倍数的计算
#### 3.2.1 约数和倍数的定义
* **约数:**一个数能被另一个数整除的数。
* **倍数:**一个数能被另一个数整除的数。
例如,6 的约数有 1、2、3、6,6 的倍数有 6、12、18、24。
#### 3.2.2 代码实现
```java
import java.util.Scanner;
public class FactorsAndMultiples {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
// 获取一个数
System.out.println("请输入一个数:");
int number = input.nextInt();
// 计算约数
System.out.println("约数:");
for (int i = 1; i <= number; i++) {
if (number % i == 0) {
System.out.print(i + " ");
}
}
// 计算倍数
System.out.println("\n倍数:");
for (int i = 1; i <= number; i++) {
if (i % number == 0) {
System.out.print(i + " ");
}
}
}
}
```
**代码逻辑分析:**
1. `main` 方法获取一个数,然后计算约数和倍数。
2. 计算约数时,遍历 1 到该数,判断每个数是否能整除该数。
3. 计算倍数时,遍历 1 到该数,判断每个数是否能被该数整除。
4. 约数和倍数通过 `System.out.print` 输出。
# 4. 最大公约数算法的优化
### 4.1 快速幂算法
#### 4.1.1 快速幂算法的原理
快速幂算法是一种用于快速计算大数幂的算法。其基本思想是将指数分解为二进制位,并通过逐位计算的方式来减少乘法运算的次数。
具体来说,快速幂算法的步骤如下:
1. 将指数转换为二进制表示。
2. 从二进制表示的最低位开始,依次判断当前位是否为 1。
3. 如果当前位为 1,则将当前结果与底数相乘。
4. 将底数平方,并将指数右移一位。
5. 重复步骤 3 和 4,直到指数为 0。
#### 4.1.2 代码实现
```java
public static long fastPow(long base, long exponent) {
long result = 1;
while (exponent > 0) {
if ((exponent & 1) == 1) {
result *= base;
}
base *= base;
exponent >>= 1;
}
return result;
}
```
**代码逻辑分析:**
* `base` 为底数,`exponent` 为指数。
* `result` 存储计算结果。
* 循环遍历指数的二进制位,判断当前位是否为 1。
* 如果当前位为 1,则将结果与底数相乘。
* 将底数平方,并将指数右移一位。
* 重复上述步骤,直到指数为 0。
### 4.2 二进制位运算优化
#### 4.2.1 二进制位运算的原理
二进制位运算是一种利用二进制位进行计算的算法。在最大公约数算法中,可以通过利用二进制位运算来优化辗转相除法。
具体来说,辗转相除法中需要不断地求两个数的余数。而二进制位运算中的 `&` 操作可以快速求出两个数的公共二进制位,从而减少除法运算的次数。
#### 4.2.2 代码实现
```java
public static int gcd(int a, int b) {
if (b == 0) {
return a;
}
return gcd(b, a % b);
}
public static int gcdOptimized(int a, int b) {
while (b != 0) {
int temp = a;
a = b;
b = temp & b;
}
return a;
}
```
**代码逻辑分析:**
* `gcd` 函数为原始的辗转相除法实现。
* `gcdOptimized` 函数为优化后的版本,利用了二进制位运算。
* 循环遍历两个数,不断地将较大数与较小数的 `&` 结果作为新的较小数。
* 当较小数为 0 时,较大会数即为最大公约数。
# 5. **5.1 多个整数的最大公约数**
**5.1.1 算法原理**
计算多个整数的最大公约数可以采用递归的方法。首先,计算前两个整数的最大公约数,然后将这个最大公约数与第三个整数计算最大公约数,以此类推,直到计算出所有整数的最大公约数。
**5.1.2 代码实现**
```java
public static int gcd(int... numbers) {
if (numbers.length == 0) {
throw new IllegalArgumentException("No numbers provided");
}
int result = numbers[0];
for (int i = 1; i < numbers.length; i++) {
result = gcd(result, numbers[i]);
}
return result;
}
```
**代码说明:**
* `gcd` 方法接收可变数量的整数作为参数,并返回这些整数的最大公约数。
* 如果没有提供任何数字,则抛出 `IllegalArgumentException`。
* 循环遍历所有数字,并逐个计算最大公约数。
* `gcd` 方法是递归调用的,直到只有一个数字剩余为止。
0
0
相关推荐
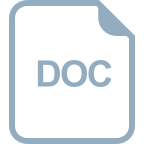
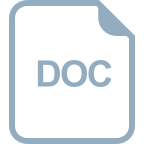
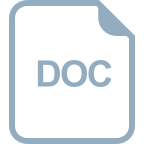
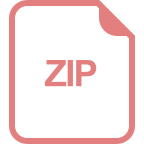
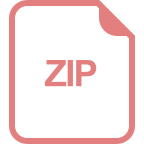
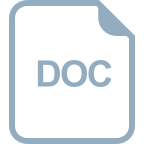