Java最大公约数算法:在计算机图形学中的应用解析
发布时间: 2024-08-27 22:56:02 阅读量: 21 订阅数: 11 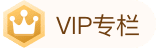
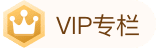
# 1. Java最大公约数算法**
最大公约数(GCD)算法在计算机图形学中广泛应用,用于计算两个或多个整数的最大公约数。在Java中,可以使用以下算法计算GCD:
```java
public static int gcd(int a, int b) {
if (b == 0) {
return a;
} else {
return gcd(b, a % b);
}
}
```
该算法基于欧几里得算法,通过递归地计算余数来逐步缩小输入整数,直到余数为0,此时返回非0整数作为GCD。
# 2. 最大公约数算法在计算机图形学中的理论基础
### 2.1 最大公约数算法的基本原理
最大公约数(Greatest Common Divisor,GCD)算法是一种用于计算两个或多个整数的最大公约数的算法。最大公约数是这些整数的公约数中最大的一个。
在计算机图形学中,最大公约数算法用于解决各种问题,例如:
* **三维建模:**计算多边形网格的顶点、边和面的数量。
* **纹理映射:**计算纹理坐标的整数部分。
* **动画:**计算关键帧之间的插值。
最常用的最大公约数算法是欧几里得算法,该算法基于以下定理:
> 两个整数 a 和 b 的最大公约数等于 a 和 b 的余数的最大公约数。
欧几里得算法通过反复计算余数来求解最大公约数。算法从计算 a 除以 b 的余数开始,然后将 b 替换为余数,并重复该过程,直到余数为 0。此时,最后一个非零余数就是 a 和 b 的最大公约数。
### 2.2 最大公约数算法在计算机图形学中的应用场景
#### 2.2.1 三维建模
在三维建模中,最大公约数算法用于计算多边形网格的顶点、边和面的数量。多边形网格是由顶点、边和面组成的三维对象。
为了计算顶点、边和面的数量,需要计算网格中每个多边形的顶点数、边数和面数。最大公约数算法可以用来计算每个多边形的顶点数和边数。
**代码块:**
```python
def compute_polygon_info(polygon):
"""
计算多边形的顶点数和边数。
Args:
polygon (list): 多边形顶点列表。
Returns:
tuple: 顶点数和边数。
"""
num_vertices = len(polygon)
num_edges = num_vertices - 1
return num_vertices, num_edges
```
**逻辑分析:**
该代码块计算多边形的顶点数和边数。顶点数等于多边形顶点列表的长度。边数等于顶点数减去 1。
#### 2.2.2 纹理映射
在纹理映射中,最大公约数算法用于计算纹理坐标的整数部分。纹理坐标是用于将纹理映射到三维模型上的坐标。
为了计算纹理坐标的整数部分,需要将纹理坐标除以纹理大小。纹理大小是纹理的宽度或高度。最大公约数算法可以用来计算纹理坐标除以纹理大小的余数。
**代码块:**
```python
def compute_texture_coordinate(texture_coordinate, texture_size):
"""
计算纹理坐标的整数部分。
Args:
texture_coordinate (float): 纹理坐标。
texture_size (int): 纹理大小。
Returns:
int: 纹理坐标的整数部分。
"""
return texture_coordinate // texture_size
```
**逻辑分析:**
该代码块计算纹理坐标的整数部分。纹理坐标除以纹理大小,然后取商作为整数部分。
#### 2.2.3 动画
在动画中,最大公约数算法用于计算关键帧之间的插值。关键帧是动画中定义对象位置、旋转和缩放的帧。
为了计算关键帧之间的插值,需要计算关键帧之间的时间差。最大公约数算法可以用来计算关键帧之间的时间差的最小公约数。
**代码块:**
```python
def compute_time_difference(keyframe1, keyframe2):
"""
计算关键帧之间的时间差的最小公约数。
Args:
keyframe1 (dict): 关键帧 1。
keyframe2 (dict): 关键帧 2。
Returns:
int: 关键帧之间的时间差的最小公约数。
"""
time_difference = keyframe2["time"] - keyframe1["time"]
gcd = math.gcd(time_difference, 1)
return gcd
```
**逻辑分析:**
该代码块计算关键帧之间的时间差的最小公约数。时间差是关键帧 2 的时间减去关键帧 1 的时间。最小公约数是时间差和 1 的最大公约数。
# 3.1 最大公约数算法在三维建模中的应用
在三维建模中,最大公约数算法被广泛用于简化模型的几何形状,减少模型的复杂度。具体来说,可以通过以下步骤应用最大公约数算法:
1. **提取模型的边缘和面:**将三维模型分解为其组成边缘和面。
2. **计算边缘和面的最大公约数:**对于每对边缘或面,计算它们的长度或面积的最大公约数。
3. **合并具有相同最大公约数的边缘或面:**将具有相同最大公约数的边缘或面合并为一个新的边缘或面。
通过这种方法,可以简化模型的几何形状,同时保持其基本特征。这对于减少模型的复杂度和提高渲染效率非常重要。
**代码块:**
```python
def simplify_model(model):
# 提取模型的边缘和面
```
0
0
相关推荐
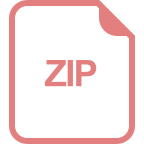
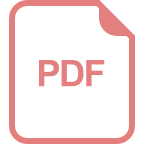
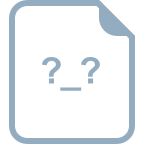





